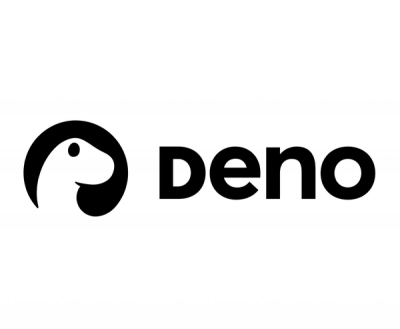
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
github.com/bnkamalesh/webgo/v2
WebGo is a minimalistic framework for Go to build web applications (server side). Unlike full-fledged frameworks out there, it tries to get out of the developers' way as soon as possible. It has always been and will always be Go standard library compliant. With the HTTP handlers having the same signature as http.HandlerFunc.
WebGo provides the following features/capabilities
The multiplexer/router is one of the most important component of a web application. It helps identifying HTTP requests and pass them on to respective handlers. A handler is uniquely identified using a URI. WebGo supports defining URIs with the following patterns
/api/users
/api/users/:userID
userID
(named URI parameter)/api/users/johndoe/account
. It only matches till /api/users/johndoe/
/api/users/:misc*
misc
/api/users
If multiple patterns match the same URI, the first matching handler would be executed. Refer to the sample to see how routes are configured. A WebGo Route is defined as following:
webgo.Route{
Name string
Method string
Pattern string
TrailingSlash bool
FallThroughPostResponse bool
Handlers []http.HandlerFunc
}
You can access the URI named parameters using the Context
function.
func helloWorld(w http.ResponseWriter, r *http.Request) {
// WebGo context
wctx := webgo.Context(r)
// URI paramaters, map[string]string
params := wctx.Params
// route, the webgo.Route which is executing this request
route := wctx.Route
webgo.R200(
w,
fmt.Sprintf(
"Route name: '%s', params: '%s'",
route.Name,
params,
),
)
}
Handler chaining lets you execute multiple handlers for a given route. Execution of a chain can be configured to run even after a previous handler has written a response to the http response. This is made possible by setting FallThroughPostResponse
to true
(refer sample).
webgo.Route{
Name: "chained",
Method: http.MethodGet,
Pattern: "/api",
TrailingSlash: false,
FallThroughPostResponse: true,
Handlers []http.HandlerFunc{
handler1,
handler2,
.
.
.
}
}
WebGo middleware lets you wrap all the routes with a middleware. Unlike handler chaining, applies to the whole router. All middlewares should be of type Middlware. The router exposes a method Use to add a Middleware the to the router. Following code shows how a middleware can be used in WebGo.
import (
"github.com/bnkamalesh/webgo"
"github.com/bnkamalesh/webgo/middleware"
)
func routes() []*webgo.Route {
return []*webgo.Route{
&webo.Route{
Name: "home",
Pattern: "/",
Handlers: []http.HandlerFunc{
func(w http.ResponseWriter, r *http.Request) {
webgo.R200(w, "home")
}
},
},
}
}
func main() {
router := webgo.NewRouter(*webgo.Config, routes())
router.Use(middleware.AccessLog)
router.Start()
}
Any number of middleware can be added to the router, the order of execution of middleware would be LIFO (Last In First Out). i.e. in case of the following code
func main() {
router.Use(middleware.AccessLog)
router.Use(middleware.CorsWrap())
}
CorsWrap would be executed first, followed by AccessLog.
WebGo provides a few helper functions.
Few more helper functions are available, you can check them here.
When using Send
or SendResponse
, the response is wrapped in WebGo's response struct and is sent as JSON.
{
"data": "<any valid JSON payload>",
"status": "<HTTP status code, of type integer>"
}
When using SendError
, the response is wrapped in WebGo's error response struct and is sent as JSON.
{
"errors": "<any valid JSON payload>",
"status": "<HTTP status code, of type integer>"
}
HTTPS server can be started easily, by providing the key & cert file. You can also have both HTTP & HTTPS servers running side by side.
Start HTTPS server
cfg := &webgo.Config{
Port: "80",
HTTPSPort: "443",
CertFile: "/path/to/certfile",
KeyFile: "/path/to/keyfile",
}
router := webgo.NewRouter(cfg, routes())
router.StartHTTPS()
Starting both HTTP & HTTPS server
cfg := &webgo.Config{
Port: "80",
HTTPSPort: "443",
CertFile: "/path/to/certfile",
KeyFile: "/path/to/keyfile",
}
router := webgo.NewRouter(cfg, routes())
go router.StartHTTPS()
router.Start()
Graceful shutdown lets you shutdown the server without affecting any live connections/clients connected to the server. It will complete executing all the active/live requests before shutting down.
Sample code to show how to use shutdown
func main() {
osSig := make(chan os.Signal, 5)
cfg := &webgo.Config{
Host: "",
Port: "8080",
ReadTimeout: 15 * time.Second,
WriteTimeout: 60 * time.Second,
ShutdownTimeout: 15 * time.Second,
}
router := webgo.NewRouter(cfg, routes())
go func() {
<-osSig
// Initiate HTTP server shutdown
err := router.Shutdown()
if err != nil {
fmt.Println(err)
os.Exit(1)
} else {
fmt.Println("shutdown complete")
os.Exit(0)
}
// If you have HTTP server running, you can use the following code
// err := router.ShutdownHTTPS()
// if err != nil {
// fmt.Println(err)
// os.Exit(1)
// } else {
// fmt.Println("shutdown complete")
// os.Exit(0)
// }
}()
signal.Notify(osSig, os.Interrupt, syscall.SIGTERM)
router.Start()
for {
// Prevent main thread from exiting, and wait for shutdown to complete
time.Sleep(time.Second * 1)
}
}
WebGo exposes a singleton+global logger variable LOGHANDLER with which you can plugin your custom logger. Any custom logger should implement WebGo's Logger interface.
type Logger interface {
Debug(data ...interface{})
Info(data ...interface{})
Warn(data ...interface{})
Error(data ...interface{})
Fatal(data ...interface{})
}
A fully functional sample is provided here. You can try the following API calls with the sample app.
http://localhost:8080/
http://localhost:8080/matchall/
/matchall
will be matched because it has a wildcard variable/api/<single parameter>
If you have Go installed on your system, open your terminal and:
$ cd $GOPATH/src
$ mkdir -p github.com/bnkamalesh
$ cd github.com/bnkamalesh
$ git clone https://github.com/bnkamalesh/webgo.git
$ cd webgo
$ go run cmd/main.go
Info 2019/07/09 18:35:54 HTTP server, listening on :8080
Or if you have Docker, open your terminal and:
$ git clone https://github.com/bnkamalesh/webgo.git
$ cd webgo
$ docker run \
-p 8080:8080 \
-v ${PWD}:/go/src/github.com/bnkamalesh/webgo/ \
-w /go/src/github.com/bnkamalesh/webgo/cmd \
--rm -ti golang:latest go run main.go
Info 2019/07/09 18:35:54 HTTP server, listening on :8080
The gopher used here was created using Gopherize.me. WebGo stays out of developers' way, and they can sitback and enjoy a cup of coffee just like this gopher, while using WebGo.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.