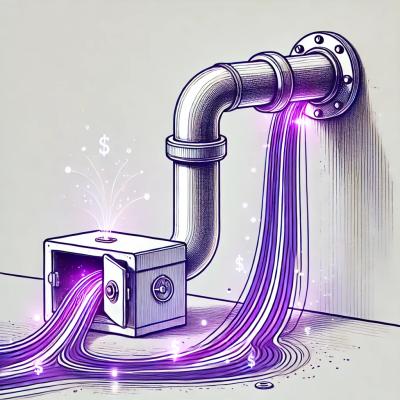
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
github.com/darkrockmountain/gomail
This project provides implementations for sending emails using different services including SMTP, Gmail API, Microsoft Graph API, SendGrid, AWS SES, Mailgun, Mandrill, Postmark, and SparkPost. Each implementation follows a common interface, allowing for flexibility and easy integration with various email services.
go get github.com/darkrockmountain/gomail
git clone https://github.com/darkrockmountain/gomail.git
cd gomail
go mod tidy
providers/smpt/smtp_email_sender.go
.smtpExample()
function to send a test email.providers/gmail/gmail_email_sender.go
.gExample()
function to send a test email.providers/gmail/gmail_email_sender_oauth2.go
.gExampleOauth2()
function to send a test email.providers/microsoft365/microsoft365_email_sender.go
.msGraphExample()
function to send a test email.providers/sendgrid/sendgrid_email_sender.go
.sendgridExample()
function to send a test email.providers/ses/ses_email_sender.go
.sesExample()
function to send a test email.providers/mailgun/mailgun_email_sender.go
.mailgunExample()
function to send a test email.providers/mandrill/mandrill_email_sender.go
.mandrillExample()
function to send a test email.providers/postmark/postmark_email_sender.go
.postmarkExample()
function to send a test email.providers/sparkpost/sparkpost_email_sender.go
.sparkpostExample()
function to send a test email.For detailed instructions on obtaining the necessary credentials for each implementation, refer to the respective documentation files in the docs
directory.
This project is licensed under the Apache-2.0 License - see the LICENSE file for details.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.