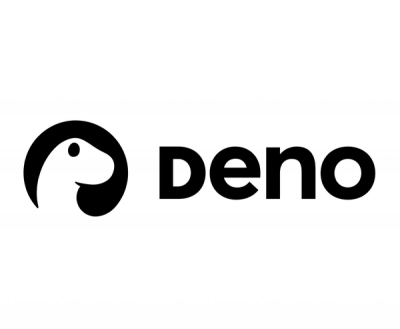
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
github.com/kelr/gundyr
Gundyr provides an easy to use interface to the Helix Twitch API and Twitch PubSub.
It handles both app access as well as user access tokens. All tokens used are automatically refreshed.
This is a work in progress and not all Helix or PubSub endpoints are supported yet.
$ go get github.com/kelr/gundyr
Documentation can be found at godoc. Examples can be found in the examples directory.
cfg := &gundyr.HelixConfig{
ClientID: clientID,
ClientSecret: clientSecret,
}
c, err := gundyr.NewHelix(cfg)
if err != nil {
log.Fatal(err)
}
userID, err := c.UserToID("kyrotobi")
if err != nil {
log.Fatal(err)
}
log.Println(userID)
If an OAuth2 token is not provided to HelixConfig, authentication will attempt to use the OAuth2 Client Credentials flow to obtain a App Access token.
// See examples/auth_token.go on creating/retrieving tokens.
cfg := &gundyr.HelixConfig{
ClientID: clientID,
ClientSecret: clientSecret,
Scopes: []string{"user:read:email"},
RedirectURI: redirectURI,
Token: token,
}
c, err := gundyr.NewHelix(cfg)
if err != nil {
log.Fatal(err)
}
email, err := c.GetUserEmail("your-username")
if err != nil {
log.Fatal(err)
}
log.Println(email)
func handleChannelPoints(event *pubsub.ChannelPointsEvent) {
fmt.Println(event.Redemption.Reward.Title)
}
func main() {
scopes := []string{"channel:read:redemptions"}
// Setup OAuth2 configuration
config, err := auth.NewUserAuth(clientID, clientSecret, redirectURI, &scopes)
if err != nil {
log.Fatal(err)
}
// See examples/auth_token.go for an example on creating a new token.
token, err := auth.RetrieveTokenFile(config, tokenFile)
if err != nil {
log.Fatal(err)
}
// Create a PubSub client and listen to the topics.
client := pubsub.NewClient(userID, token)
client.ListenChannelPoints(handleChannelPoints)
client.Connect()
select {}
}
Any and all contributions or bug fixes are appreciated.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.