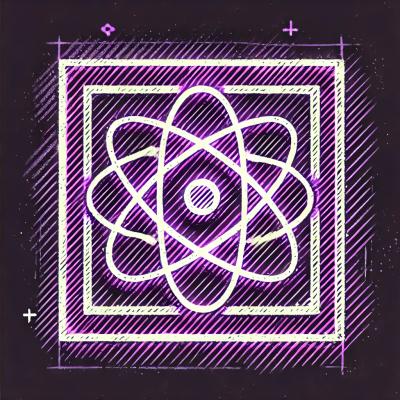
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
github.com/larry868/timeline/v2
timeline package provides 3 main types:
Duration
type extends default time.Duration
with additional Days, Month and Year methods, and with a special formating function.
Days
, Month
and Year
methods provides usefull calculations based on the following typical time values:
1 day = 24 hours
1 year = 365.25 days in average because of leap years = 8766 hours
1 month = 1 year / 12 = 30.4375 days = 730.5 hours
1 quarter = 1 year / 4 = 121.75 days = 2922 hours
Duration provides also a special formating function FormatOrderOfMagnitude
to produce an output to give an order of magnitude of the duration, at a choosen order, in a human-reading way like [-][100Y][12M][31d][24h][60m][99s][~]
with:
~
output meaning the duration in not exactExample
// more than one month
d7 := Duration(1*Month + 4*Day + 2*time.Hour + 35*time.Minute + 25*time.Second)
// Format with Order Of Magnitude of 3
// here the output starts with Months (because there is no years)
// so an order of magnitude of 3 gives 3 time components from Months: Month, Days, and Hours.
// considering lower orders not significant, or dust
fmt.Printf("%s\n", d7.FormatOrderOfMagnitude(3)) // prints: 1M4d2h~
A TimeSlice can be easily created with literal values:
ts := &TimeSlice{
From: time.Date(2022, 1, 1, 0, 0, 0, 0, time.UTC),
To: time.Date(2022, 1, 2, 0, 0, 0, 0, time.UTC),}
A TimeSlice can also be created with a factory function with a defined d duration and a starting time.
ts := MakeTimeSlice(time.Now(), 1 * Day)
TimeSlice provides basic functions to proceed with infinite boundaries (zero times) and with chronological or anti-chronological direction.
TimeSlice advanced features:
The TimeMask type provides the following scanning possibilities:
MASK_MINUTE
MASK_MINUTEx15
MASK_HALFHOUR
MASK_HOUR
MASK_HOURx4
MASK_HALFDAY
MASK_DAY
MASK_MONTH
MASK_QUARTER
MASK_YEAR
go get -u github.com/sunraylab/timeline/v2@latest
v2.5.0:
v2.4.x:
v2.3.1:
v2.3.0:
v2.2.2:
v2.2.1:
v2.1.3:
v2.1.2 :
v2.1.0:
v2.0.0:
v1.3.0:
v1.2.1:
v1.2.0:
v1.1.0:
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.