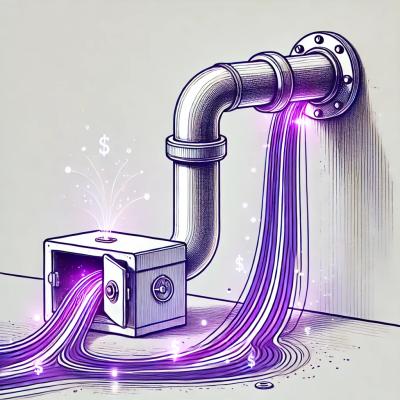
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
github.com/naufalfmm/cryptocurrency-price-api
Service for track the latest price of cryptocurrency asset
Create .env
file
NAME="Cryptocurrency Price API" # The service name
PORT=PORT # The service port. The default is 8080
DB_PATH=DB_PATH # The location of Sqlite database
DB_MAX_IDLE_CONNECTION=DB_MAX_IDLE_CONNECTION # The max idle connection of the db. The default is 10
DB_MAX_OPEN_CONNECTION=DB_MAX_OPEN_CONNECTION # The max open connection of the db. The default is 10
DB_CONNECTION_MAX_LIFE_TIME=DB_CONNECTION_MAX_LIFE_TIME # The connection maximum lifetime. The default is 60s
DB_LOG_MODE=DB_LOG_MODE # Log mode of the DB. If true, the query is printed. The default is false
DB_LOG_SLOW_THRESHOLD=DB_LOG_SLOW_THRESHOLD # Threshold of the query printed as slow. The default is 1s
DB_RETRY=DB_RETRY # The number of connection retrying. The default is 3
DB_WAIT_SLEEP=DB_WAIT_SLEEP # The sleep time between retry. The default is 1s
LOG_MODE=LOG_MODE # The log mode. If true, log is printed
BCRYPT_COST=BCRYPT_COST # The password bcrypt cost. The default is 5
JWT_PUBLIC_KEY=JWT_PUBLIC_KEY
JWT_ALG=JWT_ALG # The default is HS256
JWT_EXPIRES=JWT_EXPIRES # The default is 1h
COINCAP_BASE_PATH=COINCAP_BASE_PATH # The base path of coincap API
COINCAP_PRICE_SYNC_MODE=COINCAP_PRICE_SYNC_MODE # The mode of asset price sync via websocket. The default is false
Migrate the database by
make db DOCKER= SQLPATH= DBPATH= DBFILENAME= ENVFILENAME=
# Note:
# DOCKER= // If the migrating process use docker, please set as true.
# SQLPATH= // Set the value if the DOCKER parameter is true
# DBPATH= // Set the value if the DOCKER parameter is true
# DBFILENAME= // Set the value if the DOCKER parameter is true
# ENVFILENAME= // Set the value if the DOCKER parameter is true
Note:
The command make db
automatically create the docker image (make db_init
) and run the migration (make db_migrate
) if the DOCKER
parameter is true
Run the service by
make app DOCKER= PORT= DBPATH= DBFILENAME= ENVFILENAME=
# Note:
# DOCKER= // If the migrating process use docker, please set as true.
# PORT= // Port the service is running. Please set is as stated in the .env file.
# DBPATH= // Set the value if the DOCKER parameter is true
# DBFILENAME= // Set the value if the DOCKER parameter is true
# ENVFILENAME= // Set the value if the DOCKER parameter is true
Note:
The command make app
automatically create the docker image (make app_init
) and run the migration (make app_run
) if the DOCKER
parameter is true
If you want to test the code, run
go test ./... -count=1 -failfast
The command create the docker image of migration naufalfmm/cryptocurrency-price-api-migration
make db_init DOCKER=
# Note
# DOCKER= // If the parameter is `true`, it will build the docker image
The command create the sql file
make db_create DOCKER= SQLPATH= DBPATH= DBFILENAME= ENVFILENAME=
# Note:
# DOCKER= // If the file migration creation use docker, please set as true.
# SQLPATH= // Set the value if the DOCKER parameter is true
# DBPATH= // Set the value if the DOCKER parameter is true
# DBFILENAME= // Set the value if the DOCKER parameter is true
# ENVFILENAME= // Set the value if the DOCKER parameter is true
The command migrates all sql files in ./migrates/sql
or ./sql
make db_migrate DOCKER= SQLPATH= DBPATH= DBFILENAME= ENVFILENAME=
# Note:
# DOCKER= // If the migrating process use docker, please set as true.
# SQLPATH= // Set the value if the DOCKER parameter is true
# DBPATH= // Set the value if the DOCKER parameter is true
# DBFILENAME= // Set the value if the DOCKER parameter is true
# ENVFILENAME= // Set the value if the DOCKER parameter is true
The command rollbacks all sql files in ./migrates/sql
or ./sql
make db_migrate DOCKER= SQLPATH= DBPATH= DBFILENAME= ENVFILENAME= VERSION=
# Note:
# DOCKER= // If the migrating process use docker, please set as true.
# SQLPATH= // Set the value if the DOCKER parameter is true
# DBPATH= // Set the value if the DOCKER parameter is true
# DBFILENAME= // Set the value if the DOCKER parameter is true
# ENVFILENAME= // Set the value if the DOCKER parameter is true
# VERSION= // The value is optional. If the VERSION parameter set, the sql files will be rollback-ed until the VERSION value
If the JWT inputted in Authorization
header is not valid, the endpoint return
// Response (401)
{
"ok": false,
"message": "invalid token",
"data": {
"error": "invalid token"
}
}
Endpoint to sign up new user
{
"email": "", // user's email. Requred
"password": "", // user's pasword. Required
"password_confirmation": "" // Required. The password confirmation must be same as password
}
{
"ok": true,
"message": "Success",
"data": {
"id": 0,
"email": ""
}
}
{
"ok": false,
"message": "email has been used",
"data": {
"error": "email has been used"
}
}
{
"ok": false,
"message": "internal server error",
"data": {
"error": "internal server error"
}
}
Endpoint to sign in
{
"email": "", // user's email. Requred
"password": "", // user's pasword. Required
}
{
"ok": true,
"message": "Success",
"data": {
"jwt": "xxxxx.yyyyy.zzzzz", // the JWT of user
"user": {
"id": 0,
"email": ""
}
}
}
{
"ok": false,
"message": "wrong password",
"data": {
"error": "wrong password"
}
}
{
"ok": false,
"message": "email missing",
"data": {
"error": "email missing"
}
}
{
"ok": false,
"message": "internal server error",
"data": {
"error": "internal server error"
}
}
Endpoint for add the coin to the user's tracker. Coin cannot be added to the tracker more than once.
Bearer <JWT>
{
"coin_symbol": "ETH" // the coin symbol of coincap (coincap.io)
}
{
"ok": true,
"message": "Success",
"data": {
"track_id": 0, // The track id of the user coin
"code": "ETH", // The coincap symbol of the coin
"name": "Ethereum", // The name of the coin
"latest_price": 2919.4101049055726, // The latest price of the coin
"latest_price_currency": "USD", // The currency of coin latest price
"added_at": "2024-05-12T10:14:26.5455845+07:00", // The time of coin added to the tracker
"added_by": ""
}
}
{
"ok": false,
"message": "coin has been added for the user",
"data": {
"error": "coin has been added for the user"
}
}
{
"ok": false,
"message": "internal server error",
"data": {
"error": "internal server error"
}
}
Endpoint for remove the coin to the user's tracker
Bearer <JWT>
{
"coin_symbol": "ETH" // the coin symbol of coincap (coincap.io)
}
{
"ok": true,
"message": "Success"
}
{
"ok": false,
"message": "coin is missing",
"data": {
"error": "coin is missing"
}
}
{
"ok": false,
"message": "tracking coin is missing",
"data": {
"error": "tracking coin is missing"
}
}
{
"ok": false,
"message": "internal server error",
"data": {
"error": "internal server error"
}
}
Bearer <JWT>
{
"ok": true,
"message": "Success",
"data": {
"items": [
{
"track_id": 12,
"code": "ETH",
"name": "Ethereum",
"latest_price": 2931.13,
"latest_price_currency": "USD",
"added_at": "2024-05-12T10:14:26.5455845+07:00",
"added_by": ""
}
]
}
}
{
"ok": false,
"message": "internal server error",
"data": {
"error": "internal server error"
}
}
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.