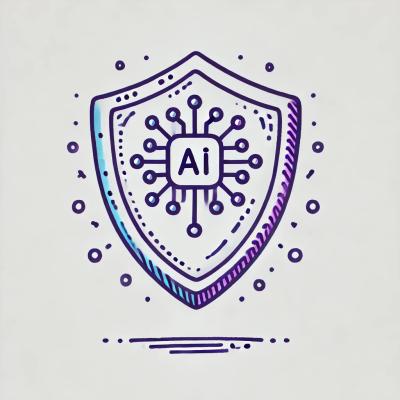
Security News
38% of CISOs Fear Theyβre Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
github.com/ners1us/trie
Module with concurrent safe trie implementation.
go get github.com/ners1us/trie
A trie, also known as a prefix tree, is a specialized tree data structure used to store a dynamic set of strings. Unlike binary trees, tries are particularly efficient for retrieval operations, especially when dealing with prefixes.
This implementation uses RWMutex
and prevents race conditions by synchronizing concurrent read and write access to the trie.
The Insert
method adds a new word to the trie. Here's how it works:
The Search
method checks if a complete word exists in the trie:
The StartsWith
method checks if any word in the trie begins with the given prefix:
Unlike Search
, this method doesn't check the isEnd
flag since it only verifies the prefix existence.
The Remove
method deletes a word from the trie if it exists:
Consider inserting the words: cat, car and dog into a trie:
root
βββ c
β βββ a
β βββ t (End of Word)
β βββ r (End of Word)
βββ d
βββ o
βββ g (End of Word)
Insert "cat"
Insert "car"
Insert "dog"
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.