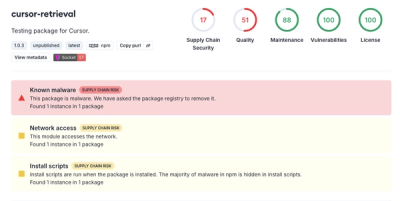
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
github.com/obrhoff/spotify/v2
This is a Go wrapper for working with Spotify's Web API.
It aims to support every task listed in the Web API Endpoint Reference, located here.
By using this library you agree to Spotify's Developer Terms of Use.
To install the library, simply
go get github.com/zmb3/spotify/v2
Spotify uses OAuth2 for authentication and authorization.
As of May 29, 2017 all Web API endpoints require an access token.
You can authenticate using a client credentials flow, but this does not provide
any authorization to access a user's private data. For most use cases, you'll
want to use the authorization code flow. This package includes an Authenticator
type to handle the details for you.
Start by registering your application at the following page:
https://developer.spotify.com/my-applications/.
You'll get a client ID and secret key for your application. An easy way to provide this data to your application is to set the SPOTIFY_ID and SPOTIFY_SECRET environment variables. If you choose not to use environment variables, you can provide this data manually.
// the redirect URL must be an exact match of a URL you've registered for your application
// scopes determine which permissions the user is prompted to authorize
auth := spotifyauth.New(spotifyauth.WithRedirectURL(redirectURL), spotifyauth.WithScopes(spotifyauth.ScopeUserReadPrivate))
// get the user to this URL - how you do that is up to you
// you should specify a unique state string to identify the session
url := auth.AuthURL(state)
// the user will eventually be redirected back to your redirect URL
// typically you'll have a handler set up like the following:
func redirectHandler(w http.ResponseWriter, r *http.Request) {
// use the same state string here that you used to generate the URL
token, err := auth.Token(r.Context(), state, r)
if err != nil {
http.Error(w, "Couldn't get token", http.StatusNotFound)
return
}
// create a client using the specified token
client := spotify.New(auth.Client(r.Context(), token))
// the client can now be used to make authenticated requests
}
You may find the following resources useful:
Spotify's Web API Authorization Guide: https://developer.spotify.com/web-api/authorization-guide/
Go's OAuth2 package: https://godoc.org/golang.org/x/oauth2/google
The API will throttle your requests if you are sending them too rapidly.
The client can be configured to wait and re-attempt the request.
To enable this, set the AutoRetry
field on the Client
struct to true
.
For more information, see Spotify rate-limits.
Examples of the API can be found in the examples directory.
You may find tools such as Spotify's Web API Console or Rapid API valuable for experimenting with the API.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.