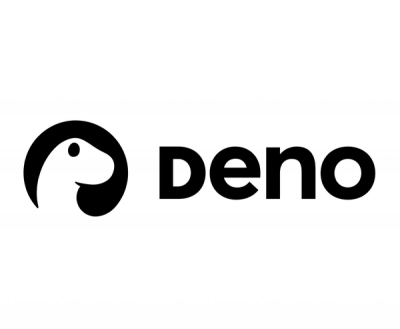
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
github.com/titanlien/asynchronous-events
In this milestone:
I was able to test all of the code created in this milestone on my local machine. The instructions below assume you are running on your local machine. I implemented this on a Mac, so references to the command-line will show as a UNIX shell.
milestone5/code
folder)
~/.bash_profile
, make sure you have the following ENV var set: export GO111MODULE=on
and make sure the file is sourced.$ go mod init
$ go mod tidy
$ go run order/main.go
milestone5/code
folder)
~/.bash_profile
, make sure you have the following ENV var set: export GO111MODULE=on
and make sure the file is sourced.$ go mod init
$ go mod tidy
$ go run inventory/main.go
milestone5/code
folder)
~/.bash_profile
, make sure you have the following ENV var set: export GO111MODULE=on
and make sure the file is sourced.$ go mod init
$ go mod tidy
$ go run warehouse/main.go
milestone5/code
folder)
~/.bash_profile
, make sure you have the following ENV var set: export GO111MODULE=on
and make sure the file is sourced.$ go mod init
$ go mod tidy
$ go run notification/main.go
milestone5/code
folder)
~/.bash_profile
, make sure you have the following ENV var set: export GO111MODULE=on
and make sure the file is sourced.$ go mod init
$ go mod tidy
$ go run shipper/main.go
$ curl -v -H "Content-Type: application/json" -d '{"id":"6e042f29-350b-4d51-8849-5e36456dfa48","products":[{"productCode":"12345","quantity":2}],"customer":{"firstName":"Tom","lastName":"Hardy","emailAddress":"tom.hardy@email.com","shippingAddress":{"line1":"123 Anywhere St","city":"Anytown","state":"AL","postalCode":"12345"}}}' http://localhost:8080/orders
$ $KAFKA_HOME/bin/kafka-console-consumer.sh --bootstrap-server localhost:9092 --topic OrderReceived --from-beginning
$ $KAFKA_HOME/bin/kafka-console-consumer.sh --bootstrap-server localhost:9092 --topic Notification --from-beginning
$ $KAFKA_HOMEbin/kafka-console-producer.sh --bootstrap-server localhost:9092 --topic OrderPickedAndPacked
Then you can past a payload like this
{"EventBase":{"EventID":"4a651ef8-a851-4d77-a58b-3d8af748a570","EventTimestamp":"2020-08-16T16:03:05.258542-04:00"},"EventBody":{"id":"c6b37316-b4da-4b25-94c8-14c08bad95e6","products":[{"productCode":"12345","quantity":2}],"customer":{"firstName":"Tom","lastName":"Hardy","emailAddress":"tom.hardy@email.com","shippingAddress":{"line1":"123 Anywhere St","city":"Anytown","state":"AL","postalCode":"12345"}}}}
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.