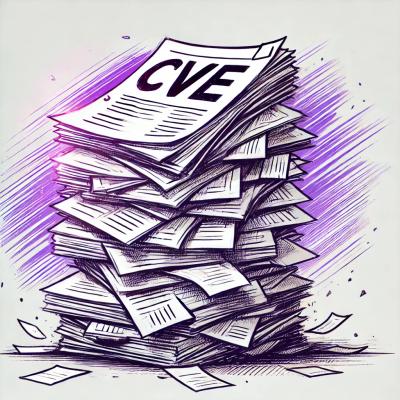
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
github.com/veliovgroup/meteor-internationalization
File based, fast, lightweight (325 lines with comments) and reactive internationalization isomorphic driver for Meteor with support of placeholders.
meteor add ostrio:i18n
/* Isomorphic (Both server and client) */
i18nConfig = {
settings: { //--> Config object
defaultLocale: "en",
ru: {
code: "ru",
isoCode: "ru-RU",
name: "Русский"
},
en: {
code: "en",
isoCode: "en-US",
name: "English"
}
},
ru:{ //--> Localization folder with name of country two-letter code
property: "value",
property2: {
nestedProp: "value"
}
},
en:{ //--> Localization folder with name of country two-letter code
property: "value",
property2: {
nestedProp: "value"
}
}
...
};
this.i18n = new I18N({driver: 'Object', i18n: i18nConfig});
private/
└─ i18n/ //--> Driver's dir
|
├── en/ //--> Localization folder with name of country two-letter code
| ├── file.json
| └── subFolder/
| └── anotherFile.json
|
├── de/ //--> Localization folder with name of country two-letter code
| ├── file.json
| └── subFolder/
| └── anotherFile.json
|
└── i18n.json //--> Config file
this.i18n = new I18N(config);
// `this` is used to create application wide variable
Configuration object:
config.driver
{String: Mongo|Object} - Driver type. Use Mongo
for file-based and Object
object-basedconfig.i18n
{Object} - Internalization objectconfig.path
{String} - Path to i18n
folder, default: /assets/app/i18n
(which points to: private/i18n
in dev environment)config.returnKey
{Boolean} - Return key if l10n value not found, default: true
config.collectionName
{String} - i18n Collection name, default: internalization
config.helperName
{String} - Template helper name, default: i18n
config.helperSettingsName
{String} - Settings helper name, default: i18nSettings
config.allowPublishAll
{Boolean} - Allow publish full i18n set to client, default: true
get([locale,] key, [replacements...])
locale
{String} - [Optional] Two-letter locale string, used to force locale, if not set current locale is usedkey
{String} - l10n key like: folder.file.object.key
replacements..
{String|[String]|Object} - [Optional] Replacements for placeholders in l10n stringi18n.get('file.obj.key'); // Current locale, no replacements
i18n.get(locale, param); // Force locale, no replacements
i18n.get('en', 'file.obj.key');
i18n.get(param, replacements); // Current locale, with replacements
i18n.get('file.obj.key', 'User Name'); // Hello {{username}} -> Hello User Name
i18n.get(locale, param, replacements); // Force locale, with replacements
i18n.get('en', 'file.obj.key', 'User Name'); // Hello {{username}} -> Hello User Name
setLocale(locale)
locale
{String} - Two-letter locale codei18n.setLocale(locale);
i18n.currentLocale.get(); // Reactive on Client
i18n.defaultLocale;
i18n.langugeSet();
/* Returns:
{
current: 'en', // Current locale
locales: ['ru', en], // List of locales
// All locales
all: [{
code: "ru",
isoCode: "ru-RU",
name: "Русский",
path: "i18n/ru/"
},{
code: "en",
isoCode: "en-US",
name: "English",
path: "i18n/en/"
}],
// All locales except current
other: [{
code: "ru",
isoCode: "ru-RU",
name: "Русский",
path: "i18n/ru/"
}],
}
*/
key
{String} - One of the keys: current
, all
, other
, locales
, currentISO
, currentName
, currentPath
i18n.getSetting('current'); // en
i18n.userLocale; // en-US
subscribeToAll(callback)
callback
{Function} - Callback function triggered right after subscription is ready
Send full i18n and l10n set to client, might be used within iron-router
and fastrender
packages, example:i18n = new I18N();
Router.route('index', {
path: '/',
fastRender: true,
waitOn: function() {
return i18n.subscribeToAll();
}
});
i18n
- accepts locale
, key
and replacements
:
You may change name of the helper via config object: config.helperName
<p>{{i18n 'sample.hello'}}</p>
<p>{{{i18n 'sample.html'}}}</p>
<p>{{i18n 'sample.fullName'}}</p>
<p>{{i18n 'sample.fullName' 'Michael' 'A.' 'Macht'}}</p>
<p>{{i18n 'en' 'sample.fullName' 'Michael' 'A.' 'Macht'}}</p>
<p>{{i18n 'de' 'sample.fullName' first='Michael' middle='A.' last='Macht'}}</p>
<p>{{i18n 'sample.fullName' first='Michael' middle='A.' last='Macht'}}</p>
<p>{{i18n 'sample.fullName' first='Michael' middle='A.' third='Macht'}}</p>
i18nSettings
- accepts configuration object key, one of current
, all
, other
, locales
You may change name of the helper via config object: config.helperSettingsName
{{#each i18nSettings 'all'}}
...
{{/each}}
<template name="langSwitch">
{{#each i18nSettings 'all'}}
{{#if compare code '==' currentLocale}}
<span title="Current language">{{name}}</span>
{{else}}
<a href="#" data-code="{{code}}" class="switch-language">{{name}}</a>
{{/if}}
{{/each}}
</template>
Template.langSwitch.helpers({
currentLocale: function(){
return i18n.currentLocale.get()
}
});
Template.langSwitch.events({
'click .switch-language': function(e, template) {
e.preventDefault();
i18n.setLocale(e.currentTarget.dataset.code);
return false;
}
});
Template helpers compare
, ==
, Session
and many more comes from: ostrio:templatehelpers package
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.