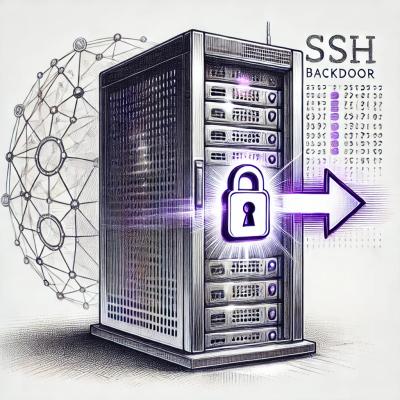
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
github.com/yehaozz/go-secure-api
This project focuses on building a small, cloud-native API service using Go, deployed on Kubernetes, with OAuth2 for authorization. The goal is to practice developing a scalable, secure API in a distributed cloud environment with modern security protocols and infrastructure automation.
curl -X POST localhost:8080/songs -i -H "Content-Type: application/json" -H "Authorization: Bearer <access_token>" -d '{"title": "Monday","artist": "Leah Dou","rating": 4.5}'
# Install metrics-server, and then add "- --kubelet-insecure-tls" to `.containers.args`
kubectl apply -f https://github.com/kubernetes-sigs/metrics-server/releases/latest/download/components.yaml
# To run the handlers tests
go test -v ./handlers
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.