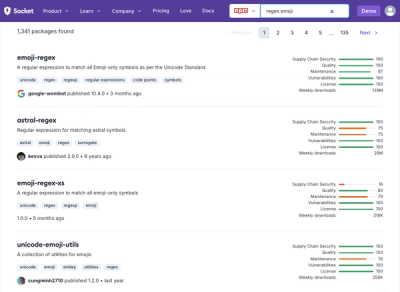
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
zvelo.io/go-signalfx
Provides a simple way to send DataPoints to SignalFx
Fully documented via godoc.
This release greatly changes the API. It cleanly separates metrics and their data points; this change also extends to ownership semantics and goroutine cleanliness.
Added Reporter.Inc
, Reporter.Sample
and Reporter.Record
for
one-shot counter, cumulative-counter and gauge values.
Metrics are a new concept: they represent metric time series, which have internal state and may be converted to a data point at a particular point in time.
Client code may define its own metrics, however, convenient-to-use Counter, WrappedCounter, CumulativeCounter, WrappedCumulativeCounter, Gauge and WrappedGauge types are provided.
To track metrics over time, use Reporter.Track
to start tracking
them and Reporter.Untrack
to stop tracking them.
Client code should no longer need to know about the sfxproto
library, which is used internally by signalfx
.
Function arguments should go from most general to most specific, e.g. from metric name, to dimensions, to value.
Create a Config
object. If $SFX_API_TOKEN
is set in the
environment, it will be used within the Config
. Other default
values are generally acceptable for most uses.
config := signalfx.NewConfig()
config.AuthToken = "<YOUR_SIGNALFX_API_TOKEN>" // if $SFX_API_TOKEN is set, this is unnecessary
Create a Reporter
object and set any dimensions that should be
set on every metric it sends to SignalFx. Optionally, call
Reporter.SetPrefix
to set a metric prefix which will be prepended
to every metric that reporter reports (this can be used to enforce
hard environment separation).
reporter := signalfx.NewReporter(config, map[string]string{
"dim0": "val0",
"dim1": "val1",
})
Add static DataPoints as needed, the value will be sent to SignalFx
later when reporter.Report
is called. All operations on the
DataPoint are goroutine safe.
reporter.Record(
"SomeGauge",
sfxproto.Dimensions{
"gauge_dim0": "gauge_val0",
"gauge_dim1": "gauge_val1",
},
5,
)
// will be reported on Metric "SomeGauge" with integer value 5
To track a metric over time, use a Metric:
counter := NewCounter("counter-metric-name", nil, 0)
reporter.Track(counter)
⋮
counter.Inc(1)
⋮
counter.Inc(3)
Reporter.Report(…) // will report a counter value of 4
Bucket
is also provided to help with reporting multiple aspects of a Metric simultaneously. All operations on Bucket
are goroutine safe.
bucket := reporter.NewBucket("SomeBucket", nil)
bucket.Add(3)
bucket.Add(5)
// 5 DataPoints will be sent.
// * Metric "SomeBucket" value of 2 with appended dimension "rollup" = "count"
// * Metric "SomeBucket" value of 8 with appended dimension "rollup" = "sum"
// * Metric "SomeBucket" value of 34 with appended dimension "rollup" = "sumsquare"
// * Metric "SomeBucket.min" value of 3 with appended dimension "rollup" = "min"
// * Metric "SomeBucket.max" value of 5 with appended dimension "rollup" = "max"
// Min and Max are reset each time bucket is reported
When ready to send the DataPoints to SignalFx, just Report
them.
reporter.Report(context.Background())
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.