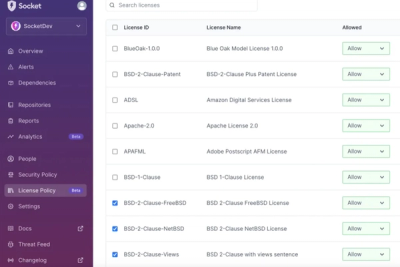
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
com.qifun:stateless-future-akka_2.10
Advanced tools
stateless-future-akka allows you to build control flow for Akka actor in the native Scala syntax.
FutureFactory
with your Actor
import com.qifun.statelessFuture.akka.FutureFactory
import akka.actor._
class YourActor extends Actor with FutureFactory {
}
receive
method from a Future
blockimport com.qifun.statelessFuture.akka.FutureFactory
import akka.actor._
class YourActor extends Actor with FutureFactory {
override def receive = Future {
???
}
}
Future
blockThere is a magic method nextMessage.await
that receive the next message from the actor's mail box. Unlike in a Actor.Receive
, you can receive multiple message sequentially:
import com.qifun.statelessFuture.akka.FutureFactory
import akka.actor._
class YourActor extends Actor with FutureFactory {
override def receive = Future {
while (true) {
val message1 = nextMessage.await
val message1 = nextMessage.await
sender ! s"You have sent me $message1 and $message2"
}
throw new IllegalStateException("Unreachable code!")
}
}
Note that the Future
block for receive
must receive all the message until the actor stops. In fact, the def receive = Future { ??? }
is a shortcut of def receive = FutureFactory.receiveForever(Future[Nothing] { ??? })
.
This example creates an actor that concantenates arbitrary number of strings.
import com.qifun.statelessFuture.akka.FutureFactory
import akka.actor._
import scala.concurrent.duration._
class ConcatenationActor extends Actor with FutureFactory {
def nextInt: Future[Int] = Future {
nextMessage.await.toString.toInt
}
override def receive = Future {
while (true) {
// Should behave the same as:
// val numberOfSubstrings = nextMessage.await.toString.toInt
val numberOfSubstrings = nextInt.await
var i = 0
val sb = new StringBuilder
while (i < numberOfSubstrings) {
sb ++= nextMessage.await.toString
i += 1
}
val result = sb.toString
println(result)
sender ! result
}
throw new IllegalStateException("Unreachable code!")
}
}
object ConcatenationActor {
def main(arguments: Array[String]) {
val system = ActorSystem("helloworld")
val concatenationActor = system.actorOf(Props[ConcatenationActor], "concatenationActor")
val inbox = Inbox.create(system)
inbox.send(concatenationActor, "4")
inbox.send(concatenationActor, "Hello")
inbox.send(concatenationActor, ", ")
inbox.send(concatenationActor, "world")
inbox.send(concatenationActor, "!")
assert(inbox.receive(5.seconds) == "Hello, world!")
inbox.send(concatenationActor, "2")
inbox.send(concatenationActor, "Hello, ")
inbox.send(concatenationActor, "world, again!")
assert(inbox.receive(5.seconds) == "Hello, world, again!")
}
}
Put these lines in your build.sbt
if you use Sbt:
libraryDependencies += "com.qifun" %% "stateless-future-akka" % "0.1.1"
stateless-future-akka
should work with Scala 2.10.3, 2.10.4, or 2.11.0.
FAQs
The ultimate control flow solution for Akka.
We found that com.qifun:stateless-future-akka_2.10 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.