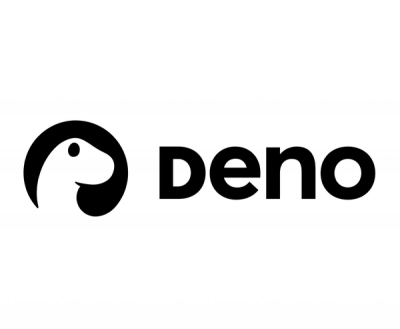
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@100mslive/react-native-video-plugin
Advanced tools
HMSSDK now provides support for Virtual Background using @100mslive/react-native-video-plugin. It allows users to change their background during a call. Users can choose from a variety of backgrounds or upload their own custom background. It also provides
Integrate virtual backgrounds, blur backgrounds effects in your React Native app with the 100ms Video Plugin. 100ms video plugin enabled adding virtual backgrounds, blur backgrounds, and other video filters to your 100ms video conferencing app. The plugin is built on top of the 100ms React Native SDK.
📖 Read the Complete Documentation here: https://www.100ms.live/docs/react-native/v2/quickstart/quickstart
📲 Download the Example iOS app here: https://testflight.apple.com/join/v4bSIPad
🤖 Download the Example Android app here: https://appdistribution.firebase.dev/i/7b7ab3b30e627c35
Virtual Background plugin helps in customising one's background that replacing the background with a static image or blurring the background. This guide provides an overview of usage of the Virtual Background plugin of 100ms.
Package | Version |
---|---|
@100mslive/react-native-room-kit | |
@100mslive/react-native-hms | |
@100mslive/react-native-video-plugin |
@100mslive/react-native-hms
SDK version is ^1.10.6
iOS 15
Install @100mslive/react-native-video-plugin
library
npm install @100mslive/react-native-video-plugin
// Import from `@100mslive/react-native-video-plugin` library
import { HMSVirtualBackgroundPlugin } from '@100mslive/react-native-video-plugin';
...
const virtualBackgroundPlugin = new HMSVirtualBackgroundPlugin();
let videoSettings = new HMSVideoTrackSettings({
initialState: HMSTrackSettingsInitState.MUTED
// The virtual background plugin to use for the video track. @type {HMSVirtualBackgroundPlugin}
videoPlugin: virtualBackgroundPlugin,
});
let trackSettings = new HMSTrackSettings({
video: videoSettings,
});
const hmsInstance = await HMSSDK.build({
trackSettings,
});
Hold on to a reference to the instance of HMSVirtualBackgroundPlugin and use enable
and disable
methods on it to enable/disable the virtual background.
const virtualBackgroundPlugin = new HMSVirtualBackgroundPlugin();
...
let isVBEnabled = false;
// Enable VB
await virtualBackgroundPlugin.enable();
isVBEnabled = true;
// Disable VB
await virtualBackgroundPlugin.disable();
isVBEnabled = false;
Always call
enable
method afterON_JOIN
andON_PREVIEW
event
Enabling Virtual Background and applying effect can take some time, you should add a loader in UI.
Enable the blur background using the setBlur
method. You should pass blur percentage ranging from 0-100
const virtualBackgroundPlugin = new HMSVirtualBackgroundPlugin();
...
// state for tracking if VB is enabled
let isVBEnabled = false;
// If VB is disabled, first enable it before calling `setBlur` method
if (isVBEnabled === false) {
await virtualBackgroundPlugin.enable();
isVBEnabled = true;
}
await virtualBackgroundPlugin.setBlur(100);
You should only call
setBlur
method only after enabling the virtual background
Enable the background image using the setBackground
method. It accepts image source (either a object with height, width and uri properties or a static image file).
Here is how to use a static image file -
const virtualBackgroundPlugin = new HMSVirtualBackgroundPlugin();
...
// state for tracking if VB is enabled
let isVBEnabled = false;
// If VB is disabled, first enable it before calling `setBlur` method
if (isVBEnabled === false) {
await virtualBackgroundPlugin.enable();
isVBEnabled = true;
}
const image = require('<PATH_TO_IMAGE_HERE>'); // ex: require('../assets/VB-1.jpg')
await virtualBackgroundPlugin.setBackground(image);
Here is how to use remote image file, setBackground
method accepts object of following type -
export interface ImageURISource {
width: number;
height: number;
/**
* `uri` is a string representing the resource identifier for the image, which
* could be an http address, a local file path, or the name of a static image
* resource (which should be wrapped in the `require('./path/to/image.png')`
* function).
*/
uri:
}
...
await virtualBackgroundPlugin.setBackground({
width,
height,
uri: 'file://...', // path of image stored in device
});
Using library like react-native-image-picker -
import { launchImageLibrary } from 'react-native-image-picker';
...
// You can use result from library like `react-native-image-picker` to use images from photo library
const result = await launchImageLibrary({ mediaType: 'photo', selectionLimit: 1 });
// getting first image
const imageObject = response.assets?.[0];
// If image is selected, use it as background
if (imageObject) {
await virtualBackgroundPlugin.setBackground(imageObject);
}
You should only call
setBackground
method only after enabling the virtual background
FAQs
HMSSDK now provides support for Virtual Background using @100mslive/react-native-video-plugin. It allows users to change their background during a call. Users can choose from a variety of backgrounds or upload their own custom background. It also provides
The npm package @100mslive/react-native-video-plugin receives a total of 9 weekly downloads. As such, @100mslive/react-native-video-plugin popularity was classified as not popular.
We found that @100mslive/react-native-video-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.