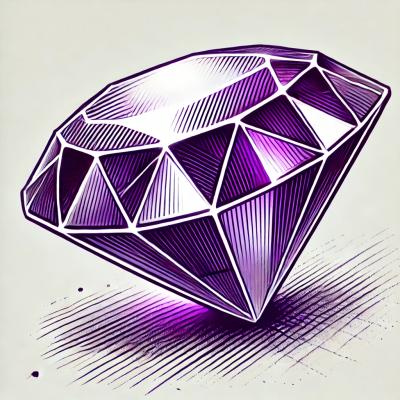
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@20i/eslint-config
Advanced tools
import baseConfig from "@20i/eslint-config"
import reactConfig from "@20i/eslint-config/react"
import pluginQuery from "@tanstack/eslint-plugin-query"
import tsEslint from "typescript-eslint"
export default tsEslint.config(
{
// global ignores
ignores: ["**/generated/**/*"],
},
{
files: ["**/*.{js,jsx,ts,tsx}"],
extends: [
// 20i configs
...baseConfig,
...reactConfig,
// other configs
...pluginQuery.configs["flat/recommended"],
],
// for type aware linting
languageOptions: {
parserOptions: {
projectService: true,
},
},
// custom rules
rules: {
"react/prop-types": "off",
"no-debugger": "warn",
},
},
// scoped rules
{
files: ["./packages/app/src/**/*.{js,jsx,ts,tsx}"],
rules: {
"@typescript-eslint/switch-exhaustiveness-check": "error",
},
}
)
We can share prettier configs now! 🎉
import sharedConfig from "@20i/eslint-config/prettier.config"
/**
* @see https://prettier.io/docs/en/configuration.html
* @type {import("prettier").Config}
*/
const config = {
...sharedConfig,
// override any shared config here
arrowParens: "avoid",
}
export default config
Make sure these extensions are installed:
code --install-extension dbaeumer.vscode-eslint && \
code --install-extension esbenp.prettier-vscode
Add the following to your global ~/.vscode/settings.json
file:
{
"editor.codeActionsOnSave": {
"source.fixAll": true,
},
// format on save for everything, but what prettier will handle through eslint
"editor.formatOnSave": true,
"[javascriptreact]": {
"editor.formatOnSave": false,
},
"[javascript]": {
"editor.formatOnSave": false,
},
"[typescript]": {
"editor.formatOnSave": false,
},
"[typescriptreact]": {
"editor.formatOnSave": false,
},
}
Restart VS Code
Inspired heavily by eslint-config-wesbos
Use mrm
to install all dependencies and add config files.
npx mrm eslint --preset @20i/mrm-preset
Install dev dependencies:
yarn add -D eslint prettier typescript @20i/eslint-config
# or
npm i -D eslint prettier typescript @20i/eslint-config
Create a new file, .eslintrc.js
, in the directory of your project.
Add the following code to the file.
module.exports = {
extends: ["@20i/eslint-config"],
parserOptions: {
project: ["./tsconfig.eslint.json"],
},
ignorePatterns: [],
}
⚠️ For React projects, set @20i/eslint-config/react
instead.
Add a special tsconfig.json
file to your project: tsconfig.eslint.json
{
// extend your base config to share compilerOptions, etc
"extends": "./tsconfig.json",
"compilerOptions": {
// ensure that nobody can accidentally use this config for a build
"noEmit": true,
},
"include": ["**/*", "**/.*"],
}
Add the following to your .prettierrc
file:
{
"endOfLine": "auto",
"semi": false,
"trailingComma": "es5",
"plugins": ["prettier-plugin-organize-imports"]
}
FAQs
ESLint and Prettier Config for Twenty Ideas
We found that @20i/eslint-config demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.