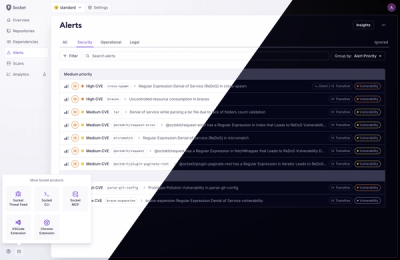
Product
A Fresh Look for the Socket Dashboard
We’ve redesigned the Socket dashboard with simpler navigation, less visual clutter, and a cleaner UI that highlights what really matters.
@3dweb/360javascriptviewer
Advanced tools
A flexible light responsive 360 image viewer with zoom functions. You can use it with npm (typescript support) or include the script on your page. Autorotate, batch loading of images, inertia and speed control. Events for making custom progress bars, intro animations and custom zoom functions. Several methods for navigation from outside which return promises for easy chaining of actions. Use all kind of images from any location with flexible start numbering and filenames.
Check for examples on 360-javascriptviewer.com
using npm with typescript declaration file:
npm i @3dweb/360javascriptviewer
import {JavascriptViewer} from "@3dweb/360javascriptviewer";
or use the CDN to get the latest version
<script src="https://cdn.jsdelivr.net/npm/@3dweb/360javascriptviewer@1/lib/JavascriptViewer.min.js"></script>
First create a div where the 360 presentation will be created. We use the size of this div to calculate zoom positions. The events for dragging and zooming are also added to this div (default #jsv-holder). Insert a image in it which is often the first image of the 360 image serie. This image will be replaced by the 360 presentation when it's fully loaded. If you don't specify otherwise this image is used to determine the other image filenames. If the main image is product.jpg, the other images in the same folder must be called product_1.jpg, product_2.jpg and so on. But if you have other filenames/locations for your 360 images, you can change this naming format behaviour with the imageUrlFormat parameter.
<script defer src="https://cdn.jsdelivr.net/npm/@3dweb/360javascriptviewer/lib/JavascriptViewer.min.js"></script>
<style>
#jsv-holder {
width:500px;
}
#jsv-holder img {
width: 100%;
}
</style>
<div id="jsv-holder">
<img id="jsv-image" alt="example" src="https://360-javascriptviewer.gumlet.io/images/phone/iphone-gold-01.png">
</div>
<script>
window.addEventListener('load', () => {
const viewer = new JavascriptViewer({
mainHolderId: 'jsv-holder',
mainImageId: 'jsv-image', // will hide after loading
imageUrlFormat: 'iphone-gold-xx.png',
totalFrames: 72,
speed: 70,
defaultProgressBar: true
});
// use events for example
viewer.events().loadImage.on((progress) => {
// use this for your own progress bar
console.log(`loading ${progress.percentage}%`)
})
viewer.events().started.on((result) => {
// use a promise or a start event to trigger things
})
viewer.start().then(() => {
viewer.rotateDegrees(180).then(() => {
// continue with your amazing intro
})
});
});
</script>
property | type | description | default |
---|---|---|---|
mainImageId | string | ID of the image which is the base for all the frames. You can also use mainImageUrl if the path of the other files are different. | jsv-image |
mainHolderId | string | ID of the div where the presentation runs in. | jsv-holder |
mainImageUrl | string | URl of the first image, doesn't have to be in the document. Not needed when using imageUrls | |
totalFrames | number | Amount of frames in the presentation, more images means smoother rotations. | 72 |
imageUrls | string[] | Array of image urls, overrides totalFrames and imageUrlFormat | [] |
firstImageNumber | number | After loading the presentation this is the first frame visible. | 1 |
reverse | boolean | Invert the rotation direction when dragging. | false |
speed | number | Speed of rotating. -199 to 100 | 80 |
inertia | number | Delay when stop dragging. | 20 |
defaultProgressBar | boolean | Disable the build in progress bar, use this when you have implemented your own loader or don't like this one. | true |
defaultProgressBarColor | string | Change the color of the progress bar, can be hex of rgb. | rgb(0, 0, 0) |
defaultProgressBarBackgroundColor | string | Change the background color of the progress bar, can be hex of rgb. | rgba(255, 255, 255, 0.5) |
defaultProgressBarHeight | string | Change the height of the progress bar, can be any css value for height. | 5px |
imageUrlFormat | string | Format for determining the filenames of the frames {filename}_xx.{extension} is the default ipod_x.jpg => ipod_1.jpg ipod_xx.jpg => ipod_01.jpg https://other.cdn/images/ipod_xx.jpg => https://other.cdn/images/ipod_01.jpg | {filename}_xx.{extension} |
extraImageClass | string | Add a class to all the images used in the presentation, separate multiple classes with a space. | empty |
imageAltText | string | Add an alt text to the images, all images will have the same alt text. | empty |
startFrameNo | number | Use this setting in combination with imageUrlFormat startFrameNo: 4 => first file is ipod_04.jpg | 1 |
autoRotate | number | Use this setting for rotating the view at start. Rotation stops when user drags the model or another animation method is called. | 0 |
autoRotateSpeed | number | Speed of autorotation. -199 to 100 | 1 |
autoRotateReverse | boolean | Use this setting for changing the direction of the auto rotating. | 1 |
enableImageEvents | boolean | Use this setting for enabling click pinch and wheel events on images. | 0 |
zoom | boolean | Use this setting for enabling zoom functions. Zoom on wheel event or pinch. | 0 |
zoomWheelSpeed | number | Change the speed of zooming with the mousewheel. | 50 |
zoomMax | number | If zoom is enabled this is the max zoom factor. | 2 |
stopAtEdges | boolean | Blocks repeating images after reaching last image. | 0 |
autoCDNResizer | boolean | Set the query params height and width for use with an image resizer. | 0 |
autoCDNResizerConfig | IAutoCDNResizerConfig | Config for adding query params to image urls. | 0 |
enableChangeImageEvent | boolean | Enable changeImage event on rotation, could be CPU heavy | 0 |
cursorConfig | ICursorConfig | Change the cursors for the presentation | |
notificationConfig | InotificationConfig | Configure the default notifications |
loadImage:IProgress
Use this event to create your own progress bar
viewer.events().loadImage.on((progress) => { // use the progress object for your own loader })
started:boolean
Use this event to trigger other actions when the viewer is ready
viewer.events().started.on((boolean) => { // start intro animations or other creative ideas })
startDragging:IHandle
Use this event to capture the user drag movement.
viewer.events().startDragging.on((handle) => { // show or hide information on the viewer or send statistics })
changeImage:IStatus
Fires when a new image becomes visible, works in combination with enableChangeImageEvent:true
viewer.events().changeImage.on((handle) => { // show or hide information on the viewer or send statistics })
endAutoRotate:IStatus
Fires when autorotate stops
viewer.events().endAutoRotate.on((status) => { // show or hide information on the viewer or send statistics })
click:IClick
Fires when the user clicks on a image, works in combination with enableImageEvents: true
viewer.events().click.on((click) => { // Use this event for zoom functions })
pinch:IPinch
Fires when the user pinch on a image (zoom on mobile with 2 fingers), works in combination with enableImageEvents: true
viewer.events().pinch.on((click) => { // Use this event for zoom functions })
scroll:IScroll
Fires when the user use the mouse scroll, works in combination with enableImageEvents: true
viewer.events().pinch.on((click) => { // Use this event for zoom functions })
doubleClick:IClick
Fires when the user double clicks on a image, works in combination with enableImageEvents: true
viewer.events().pinch.on((click) => { // Use this event for zoom functions })
IResponse {
currentDegree: number;
}
IAutoCDNResizerConfig {
useWidth: boolean; //default true
useHeight: boolean;
scaleWithZoomMax: boolean; //default false loads images with the zoomMax factor for height or width
extraParams: object; //add extra params to the image urls
}
notificationConfig {
dragToRotate: {
showStartToRotateDefaultNotification: boolean,
imageUrl: string,
languages: ITranslation[],
mainColor: string //hex,rgb or rgba color
textColor: string //hex,rgb or rgba color
}
}
ITranslation { [
language: string, //ISO Language Code (en-US,nl-NL)
text: string
]}
IStatus {
currentDegree: number;
currentImage: IImage;
completed: boolean;
}
IImage {
id: string;
src: string;
sequence: number;
}
FAQs
A 360 javascript viewer working with images
The npm package @3dweb/360javascriptviewer receives a total of 471 weekly downloads. As such, @3dweb/360javascriptviewer popularity was classified as not popular.
We found that @3dweb/360javascriptviewer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We’ve redesigned the Socket dashboard with simpler navigation, less visual clutter, and a cleaner UI that highlights what really matters.
Industry Insights
Terry O’Daniel, Head of Security at Amplitude, shares insights on building high-impact security teams, aligning with engineering, and why AI gives defenders a fighting chance.
Security News
MCP spec updated with structured tool output, stronger OAuth 2.1 security, resource indicators, and protocol cleanups for safer, more reliable AI workflows.