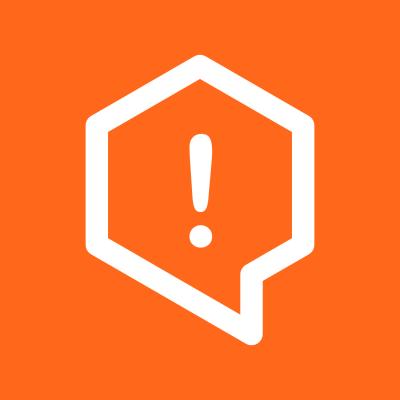
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@abtasty/pulsar-common-ui
Advanced tools
The React based library implementing AB Tasty design system. For complete information refer to the design system knowledge base.
All components are showed and documented in Storybook: https://storybook.abtasty.io.
All components are listed in the design system dashboard along with its state of usage.
This library is based on the Figma specification owned by designer team.
[[TOC]]
All components are showed and documented in Storybook: https://storybook.abtasty.io.
All components are listed in the design system dashboard along with its state of usage.
Install common-ui as dependency from the registry
yarn add @abtasty/pulsar-common-ui
Import global css styles
import '@abtasty/pulsar-common-ui/static/fonts.css';
import '@abtasty/pulsar-common-ui/static/reset.css';
import '@abtasty/pulsar-common-ui/dist/commonui.css';
Import providers (for client side application)
import { StyledProvider } from '@abtasty/pulsar-common-ui';
export const App: FC = () => (
<StyledProvider>
<YourApp />
</StyledProvider>;
);
You need to add a registry (See https://styled-components.com/docs/advanced#next.js)
import { StyledProvider } from '@abtasty/pulsar-common-ui';
import { StyledComponentsRegistry } from './registry.ts';
// ...
export default async function RootLayout({ children }: { children: React.ReactNode }) {
return (
<StyledComponentsRegistry>
<StyledThemeProvider>{children}</StyledThemeProvider>
</StyledComponentsRegistry>
);
}
// registry.ts
'use client';
import React, { FC, useState } from 'react';
import { useServerInsertedHTML } from 'next/navigation';
import { ServerStyleSheet, StyleSheetManager as StyleSheetManagerOriginal } from '@abtasty/pulsar-common-ui';
const StyleSheetManager = StyleSheetManagerOriginal as any;
export const StyledComponentsRegistry: FC<{ children: React.ReactNode }> = ({ children }) => {
// Only create stylesheet once with lazy initial state
// x-ref: https://reactjs.org/docs/hooks-reference.html#lazy-initial-state
const [styledComponentsStyleSheet] = useState(() => new ServerStyleSheet());
useServerInsertedHTML(() => {
const styles = styledComponentsStyleSheet.getStyleElement();
(styledComponentsStyleSheet.instance as any).clearTag();
return <>{styles}</>;
});
if (typeof window !== 'undefined') return <>{children}</>;
return <StyleSheetManager sheet={styledComponentsStyleSheet.instance}>{children}</StyleSheetManager>;
};
Optionally add global styles
// App.tsx
import { StyledProvider, GlobalStyles } from '@abtasty/pulsar-common-ui';
export const App: FC = () => (
//...
<GlobalStyles />
<YourApp />
//...
);
git clone git@gitlab.com:abtasty/turfu/pulsar.git
yarn workspace @abtasty/pulsar-common-ui build
cd ~/workspace/my-repo-using-common-ui
yarn link ~/workspace/pulsar/modules/common-ui/
You can specify a version when installing the library, or change the version in your package.json and install dependencies again.
yarn add @abtasty/pulsar-common-ui@1.2.0-beta-1
Default styling solution of common-ui is styled-component.
Because your application is wrapped with common-ui <StyledProvider>
any styled component will inherit the theme
property. You can also import use theme though the hook useTheme()
.
When you need to customize a component beyond of what it is currently possible through its properties or composition pattern, always trigger reflection hands in hands with designer if the customization is really needed as it might bend current design system capabilities. If it is then continue the reflection with designer to understand how to bring this new capability to the design system without breaking our rules below. If you need to modify the component checkout our best practices in the contributing guide.
Do not rely on the component internal html tag composition. Internal html composition is subject to change and will not be guaranteed by versioning and retro compatibility.
// ❌ Incorrect
const StyledButton = styled(Button)`
button > svg.icon {
margin-right: 8px;
}
`;
// ...
<StyledButton />;
// ✅ Correct
// This will highly depend on your case, but such deep customization can generally be handled by
// - leveraging composition (see other point below)
// - adding capabilities to the component itself in agreement with design team through new composition or properties
// - getting back in track with the design system state (do we really need this inner customization ???)
Prioritize usage of existing properties over custom css. Properties are meant to carry more semantic of the component, apply on the whole component directly and will be maintain through versioning.
// ❌ Incorrect
const StyledButton = styled(Button)`
color: ${({ theme }) => theme.dec.color.primary.default.fg.positive};
`;
// ✅ Correct
<Button color="success" />;
Apply style to inner component by leveraging the component composition.
// ❌ Incorrect
const StyledDropdown = styled(Dropdown)`
.option {
background: ${({ theme }) => theme.dec.color.brand.default.bg.norm};
}
`;
// ...
<StyledDropdown />;
// ✅ Correct
const StyledOption = styled(Option)`
background: ${({ theme }) => theme.dec.color.brand.default.bg.norm};
`;
// ...
<Dropdown OptionComponent={StyledOption} />;
Avoid (heavy) customization. Always double check with designer if the customization is meant to be global (aka should be directly in library) or specific to the use case.
Always rely on theme and design tokens. Do not use direct hexadecimals for colors nor magic numbers for any styles. Theme is providing already decided values with semantic meanings, use them so you'll directly inherit any changes to those values.
// ❌ Incorrect
const PositiveFeedback = styled.div`
color: #00806c;
`;
// ✅ Correct
const PositiveFeedback = styled.div`
color: ${({ theme }) => theme.dec.color.primary.default.fg.positive};
`;
We use styled-component theme
to expose design tokens (aka variables representing a design decision). This way all repeated design decisions are centralized and easy to maintain.
⚠️ Never import theme directly! Only use theme from styled-components theme provider.
These same design tokens are used in designer mockups so you need to use the same token in implementation. To map the Figma token name to our theme, simply take the last part and replace dashes -
by pointed notation .
.
Example of mapping Figma token name to styled component theme:
Surface/Brand/Default/dec-color-brand-default-bg-norm
➡️ theme.dec.color.brand.default.bg.norm
Example of getting token name in Figma:
We select the button surface we can retrieve the token used in the Selection colors section or in the style section in the form of CSS variables.
This a basic explanation of design token structure, please refer to designer documentation for a full description..
Design tokens follow a general structure type.property.variant[.state][.uiElement].extension
.
Where
dec
(decision), data
, spe
(specific), opt
(option)color
spe
), primary
, brand
, negative
, brandStrong
, ...default
, hovered
, pressed
, disabled
, ...bg
(background), fg
(foreground), sk
(stroke), sh
(shadows)norm
, tone
, subbtle
, minimal
, ...🚧 Work in progress section
- Add missing link to board
📌 Design system board
Each component has its own version mentioned as a suffix in its name (ex <DropdownV2 />
). If no version is added it is implicitly in version V0. All component usage state and version are managed in the design system board.
When a component is marked as deprecated it indicates the alternative to use.
Moving from one version to another for a specific component is documented in the Migration guide, if not please contact on #front_squad for help.
⚠️ For now we are not managing semantic versions and moving from one version of the lib to another it not straightforward. We are trying to limit breaking changes the most we can to be able to adopt changes easily. Future work might help to have semantic versions and clean changelog.
This project works in pair with common-ui-usage
package. Any consumer should implement reporting of usage using common-ui-usage
as explained here.
The monitoring helps to identify where components and props are used to better understand impact of changes, better workload assignment, faster retirement of deprecated features.
Make sure to read contribution guidelines before submitting changes.
Check out troubleshooting section in the knowledge base.
For any question related to design system use Slack channel #ask-design-system.
FAQs
Unknown package
The npm package @abtasty/pulsar-common-ui receives a total of 3,534 weekly downloads. As such, @abtasty/pulsar-common-ui popularity was classified as popular.
We found that @abtasty/pulsar-common-ui demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.