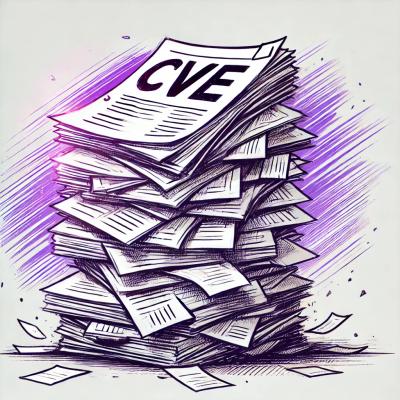
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@abubu/kaos
Advanced tools
Kaos is a simple but efficient (and hopefully hard to break) data encrypter.
Stream.Transform encrypt (key, offset = undefined)
The encrypt
API accepts two parameters and returns a Stream.Transform instance.
The first parameter is the encryption key, it can be :
If an unsupported type is used an Error with the message Unsupported key type
is thrown.
The second parameter must be used only when the encryption is done by chunks (see example).
// Simple encryption
const { promisify } = require('util')
const pipeline = promisify(require('stream').pipeline)
const { createReadStream, createWriteStream } = require('fs')
const { encrypt } = require('@abubu/kaos')
pipeline(
createReadStream('file to encrypt'),
encrypt('my secret key'),
createWriteStream('encrypted file')
)
.then(() => console.log('Encryption succeeded.'))
.catch(() => console.log('Encryption failed.'))
If the data to encrypt is available by chunks (for instance : uploaded by packets), the same salted key must be used for all parts. The start offset of each chunk must be specified for all but the first one.
// Chunks encryption
const { promisify } = require('util')
const pipeline = promisify(require('stream').pipeline)
const { createReadStream, createWriteStream } = require('fs')
const { key, encrypt } = require('@abubu/kaos')
const myKey = key('my secret key')
myKey.salt()
// mySaltedKey must be reused for each chunks
.then(mySaltedKey => pipeline(
createReadStream('file to encrypt', { start: 0, end: 50 }),
encrypt(mySaltedKey), // /!\ No offset fo the first chunk
createWriteStream('encrypted file')
))
.then(() => pipeline(
createReadStream('file to encrypt', { start: 51 }),
encrypt(mySaltedKey, 51), // Start offset of this chunk
createWriteStream('encrypted file', { flags: 'r+', start: 51 })
))
.then(() => console.log('Encryption succeeded.'))
.catch(() => console.log('Encryption failed.'))
Stream.Transform decrypt (key, range = undefined)
The decrypt
API accepts two parameters and returns a Stream.Transform instance.
The first parameter is the encryption key, similar to the encrypt
API.
The second parameter must be used only when the decryption is done by chunks (see example).
NOTE : the decryption algorithm does not verify if the data is correctly decrypted.
// Simple decryption
const { promisify } = require('util')
const pipeline = promisify(require('stream').pipeline)
const { createReadStream, createWriteStream } = require('fs')
const { decrypt } = require('@abubu/kaos')
pipeline(
createReadStream('file to decrypt'),
decrypt('my secret key'),
createWriteStream('decrypted file')
)
.then(() => console.log('Decryption succeeded.'))
.catch(() => console.log('Decryption failed.'))
When only a specific part of the message must be decoded, the key
API provides information about the ranges to load from the encrypted data.
The first step consists in reading the key salt. Once salted, the key can be reused to decode other ranges of the same encrypted data.
// Chunks decryption
const { promisify } = require('util')
const pipeline = promisify(require('stream').pipeline)
const { createReadStream, createWriteStream } = require('fs')
const { key, decrypt } = require('@abubu/kaos')
const myKey = key('my secret key')
myKey.saltRange()
.then(range => myKey.salt(createReadStream('file to decrypt', range)))
// To decode bytes between 1000 and 1100 (inclusively)
.then(saltedKey => saltedKey.byteRange(1000, 1100))
.then(range => pipeline(
createReadStream('file to decrypt', range),
decrypt(saltedKey, range),
createWriteStream('decrypted byte range')
))
.then(() => console.log('Decryption succeeded.'))
.catch(() => console.log('Decryption failed.'))
FAQs
A simple stream encrypter
The npm package @abubu/kaos receives a total of 1 weekly downloads. As such, @abubu/kaos popularity was classified as not popular.
We found that @abubu/kaos demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.