@adactive/arc-clock
Advanced tools
Comparing version 1.0.0-0 to 1.0.0
import AdsumClock from './src/AdsumClock'; | ||
export default AdsumClock; | ||
{ | ||
"name": "@adactive/arc-clock", | ||
"version": "1.0.0-0", | ||
"version": "1.0.0", | ||
"description": "Adsum Clock Component", | ||
@@ -20,2 +20,6 @@ "main": "index.js", | ||
}, | ||
"dependencies": { | ||
"chalk": "^1.1.3", | ||
"commander": "^2.9.0" | ||
}, | ||
"devDependencies": { | ||
@@ -25,2 +29,6 @@ "prop-types": "^15.6.1", | ||
}, | ||
"peerDependencies": { | ||
"prop-types": "^15.6.1", | ||
"react": "^16.3.2" | ||
}, | ||
"keywords": [ | ||
@@ -35,4 +43,9 @@ "adsum", | ||
"CHANGELOG.md", | ||
"README.md" | ||
] | ||
"README.md", | ||
"cli.js" | ||
], | ||
"bin": { | ||
"arc-clock": "./cli.js" | ||
}, | ||
"gitHead": "d36adcc832216a277301eb795e2b58f30778ce16" | ||
} |
# Clock component | ||
The clock component is, in reality, a wrapper function, which wraps the component, which you supply and provides all the props you need to create your own look and feel for the clock :) | ||
## Demo | ||
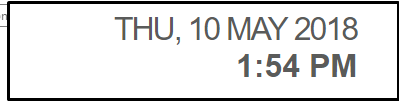 | ||
[Example here](https://adactivesas.github.io/adsum-react-components/packages/adsum-clock/examples/index.html) | ||
[Live examples here](https://adactivesas.github.io/adsum-react-components/packages/adsum-clock/examples/index.html) | ||
@@ -21,3 +22,19 @@ ## Getting started | ||
... | ||
<AdsumClock lang="en" timeFormat="12hrs" /> | ||
// Your own stateless UI component for the clock | ||
// You will be provided with props, which are described below | ||
const ClockUi = (props) => ( | ||
<div role="presentation" className="adsum-clock-wrapper"> | ||
<div className="adsum-clock"> | ||
<div className="day-date">{props.dateStr}</div> | ||
<div className="time">{props.timeStr}</div> | ||
</div> | ||
</div> | ||
); | ||
// The actual wrapping of your component with AdsumClock wrapper | ||
const Clock = AdsumClock(ClockUi); | ||
// Usage of the wrapped component | ||
<Clock lang="en" timeFormat="12hrs" /> | ||
``` | ||
@@ -28,19 +45,45 @@ | ||
```javascript | ||
AdsumClock.propTypes = { | ||
lang: PropTypes.string.isRequired, | ||
timeFormat: PropTypes.string.isRequired, | ||
style: PropTypes.objectOf(PropTypes.string) | ||
}; | ||
AdsumClock.defaultProps = { | ||
static defaultProps = { | ||
lang: 'en', | ||
timeFormat: '24hrs', | ||
style: null | ||
}; | ||
type AdsumClockPropsType = { | ||
lang: LangType, | ||
timeFormat: TimeFormatType | ||
}; | ||
``` | ||
### Additional props, which will be passed to the provided ClockUi component: | ||
```javascript | ||
lang : "en" | "fr" | "zh" | ||
timeFormat : "24hrs" | "12hrs" | ||
style : Css react object | ||
``` | ||
{ | ||
+year: string, | ||
+month: string, | ||
+day: string, | ||
+hours: string, | ||
+minutes: string, | ||
+dateStr: string, | ||
+timeStr: string | ||
}; | ||
``` | ||
```javascript | ||
type LangType = 'en' | 'zh' | 'fr'; | ||
type TimeFormatType = '24hrs' | '12hrs'; | ||
type AdsumClockPropsType = { | ||
lang: LangType, | ||
timeFormat: TimeFormatType | ||
}; | ||
``` | ||
## Copy component inside your project src folder | ||
### Less only | ||
`npx @adactive/arc-clock copy --less-only` | ||
### Full copy | ||
`npx @adactive/arc-clock copy` |
@@ -1,112 +0,133 @@ | ||
import React, { Component } from 'react'; | ||
import PropTypes from 'prop-types'; | ||
// @flow | ||
import * as React from 'react'; | ||
import type { ComponentType } from 'react'; | ||
import translate from './adsumClock.lang.json'; | ||
import './adsumClock.css'; | ||
class AdsumClock extends Component { | ||
constructor(props) { | ||
super(props); | ||
type LangType = 'en' | 'zh' | 'fr'; | ||
type TimeFormatType = '24hrs' | '12hrs'; | ||
type AdsumClockPropsType = { | ||
lang: LangType, | ||
timeFormat: TimeFormatType | ||
}; | ||
type StateType = { | ||
+year: string, | ||
+month: string, | ||
+day: string, | ||
+hours: string, | ||
+minutes: string, | ||
+dateStr: string, | ||
+timeStr: string | ||
}; | ||
type ClockUIPropsType = AdsumClockPropsType & StateType; | ||
this.state = { | ||
date: '', | ||
time: '' | ||
function ClockCreator<T: ComponentType<ClockUIPropsType>>(ClockUI: T): Element<typeof AdsumClock> { | ||
return class AdsumClock extends React.Component<AdsumClockPropsType, StateType> { | ||
static defaultProps = { | ||
lang: 'en', | ||
timeFormat: '24hrs', | ||
}; | ||
this.getTime = this.getTime.bind(this); | ||
} | ||
timerID: IntervalId = null; | ||
/** | ||
* Clock calls for update every sec | ||
*/ | ||
componentDidMount() { | ||
this.timerID = setInterval( | ||
() => this.getTime(), | ||
1000 | ||
); | ||
} | ||
state = { | ||
year: '', | ||
month: '', | ||
day: '', | ||
hours: '', | ||
minutes: '', | ||
dateStr: '', | ||
timeStr: '', | ||
}; | ||
/** | ||
* Removing interval | ||
*/ | ||
componentWillUnmount() { | ||
clearInterval(this.timerID); | ||
} | ||
/** | ||
* Clock calls for update every sec | ||
*/ | ||
componentDidMount() { | ||
this.timerID = setInterval( | ||
(): void => this.getTime(), | ||
1000, | ||
); | ||
} | ||
getTime() { | ||
const { timeFormat } = this.props; | ||
let { lang } = this.props; | ||
if(Object.keys(translate).indexOf(lang) === -1) { | ||
console.warn(`AdsumClock does not support language: ${lang}, goes to default ${AdsumClock.defaultProps.lang}`); | ||
lang = AdsumClock.defaultProps.lang; | ||
/** | ||
* Removing interval | ||
*/ | ||
componentWillUnmount() { | ||
clearInterval(this.timerID); | ||
} | ||
const time = new Date(); | ||
const day = translate[lang].days[time.getDay()]; | ||
getTime = () => { | ||
const { timeFormat } = this.props; | ||
let { lang } = this.props; | ||
const date = (time.getDate().toString().length === 1) ? `0${time.getDate()}${translate[lang].date}` : `${time.getDate()}${translate[lang].date}`; | ||
const month = (lang === 'zh') ? `${time.getMonth() + 1}${translate[lang].month}` : translate[lang].month[time.getMonth()]; | ||
const year = `${time.getFullYear()}${translate[lang].year}`; | ||
if (Object.keys(translate) | ||
.indexOf(lang) === -1) { | ||
console.warn(`AdsumClock does not support language: ${lang}, goes to default ${AdsumClock.defaultProps.lang}`); | ||
// eslint-disable-next-line prefer-destructuring | ||
lang = AdsumClock.defaultProps.lang; | ||
} | ||
let hours = ''; | ||
let minutes = ''; | ||
let timeStr = ''; | ||
const time = new Date(); | ||
const day = translate[lang].days[time.getDay()]; | ||
if (timeFormat === '12hrs') { | ||
if (time.getHours() === 12) { | ||
hours = `${time.getHours()}`; | ||
minutes = (time.getMinutes().toString().length === 1) ? `0${time.getMinutes()}` : `${time.getMinutes()}`; | ||
timeStr = `${hours}:${minutes} ${translate[lang].pm}`; | ||
} else if (time.getHours() > 12) { | ||
hours = `${time.getHours() - 12}`; | ||
minutes = (time.getMinutes().toString().length === 1) ? `0${time.getMinutes()}` : `${time.getMinutes()}`; | ||
timeStr = `${hours}:${minutes} ${translate[lang].pm}`; | ||
const dateStr = (time.getDate() | ||
.toString().length === 1) ? `0${time.getDate()}${translate[lang].date}` : `${time.getDate()}${translate[lang].date}`; | ||
const month = (lang === 'zh') ? `${time.getMonth() + 1}${translate[lang].month}` : translate[lang].month[time.getMonth()]; | ||
const year = `${time.getFullYear()}${translate[lang].year}`; | ||
let hours = ''; | ||
let minutes = ''; | ||
let timeStr = ''; | ||
if (timeFormat === '12hrs') { | ||
if (time.getHours() === 12) { | ||
hours = `${time.getHours()}`; | ||
minutes = (time.getMinutes() | ||
.toString().length === 1) ? `0${time.getMinutes()}` : `${time.getMinutes()}`; | ||
timeStr = `${hours}:${minutes} ${translate[lang].pm}`; | ||
} else if (time.getHours() > 12) { | ||
hours = `${time.getHours() - 12}`; | ||
minutes = (time.getMinutes() | ||
.toString().length === 1) ? `0${time.getMinutes()}` : `${time.getMinutes()}`; | ||
timeStr = `${hours}:${minutes} ${translate[lang].pm}`; | ||
} else { | ||
hours = `${time.getHours()}`; | ||
minutes = (time.getMinutes() | ||
.toString().length === 1) ? `0${time.getMinutes()}` : `${time.getMinutes()}`; | ||
timeStr = `${hours}:${minutes} ${translate[lang].am}`; | ||
} | ||
} else { | ||
hours = `${time.getHours()}`; | ||
minutes = (time.getMinutes().toString().length === 1) ? `0${time.getMinutes()}` : `${time.getMinutes()}`; | ||
timeStr = `${hours}:${minutes} ${translate[lang].am}`; | ||
hours = (time.getHours() | ||
.toString().length === 1) ? `0${time.getHours()}` : `${time.getHours()}`; | ||
minutes = (time.getMinutes() | ||
.toString().length === 1) ? `0${time.getMinutes()}` : `${time.getMinutes()}`; | ||
timeStr = `${hours}:${minutes}`; | ||
} | ||
} else { | ||
hours = (time.getHours().toString().length === 1) ? `0${time.getHours()}` : `${time.getHours()}`; | ||
minutes = (time.getMinutes().toString().length === 1) ? `0${time.getMinutes()}` : `${time.getMinutes()}`; | ||
timeStr = `${hours}:${minutes}`; | ||
} | ||
if (lang === 'zh') { | ||
this.setState({ | ||
date: `${day}, ${year} ${month} ${date}`, | ||
time: timeStr | ||
}); | ||
} else { | ||
this.setState({ | ||
date: `${day}, ${date} ${month} ${year}`, | ||
time: timeStr | ||
}); | ||
} | ||
} | ||
const newState = { | ||
year, | ||
month, | ||
day, | ||
hours, | ||
minutes, | ||
timeStr, | ||
}; | ||
render() { | ||
return ( | ||
<div role="presentation" className="adsum-clock-wrapper"> | ||
<div className="adsum-clock"> | ||
<div className="day-date">{this.state.date}</div> | ||
<div className="time">{this.state.time}</div> | ||
</div> | ||
</div> | ||
); | ||
} | ||
} | ||
if (lang === 'zh') { | ||
newState.dateStr = `${day}, ${year} ${month} ${dateStr}`; | ||
} else { | ||
newState.dateStr = `${day}, ${dateStr} ${month} ${year}`; | ||
} | ||
AdsumClock.propTypes = { | ||
lang: PropTypes.string.isRequired, | ||
timeFormat: PropTypes.string.isRequired, | ||
style: PropTypes.objectOf(PropTypes.string) | ||
}; | ||
this.setState(newState); | ||
}; | ||
AdsumClock.defaultProps = { | ||
lang: 'en', | ||
timeFormat: '24hrs', | ||
style: null | ||
}; | ||
render(): T { | ||
return <ClockUI {...this.props} {...this.state} />; | ||
} | ||
}; | ||
} | ||
export default AdsumClock; | ||
export default ClockCreator; |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Filesystem access
Supply chain riskAccesses the file system, and could potentially read sensitive data.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
11287
10
210
1
88
4
1
+ Addedchalk@^1.1.3
+ Addedcommander@^2.9.0
+ Addedansi-regex@2.1.1(transitive)
+ Addedansi-styles@2.2.1(transitive)
+ Addedchalk@1.1.3(transitive)
+ Addedcommander@2.20.3(transitive)
+ Addedescape-string-regexp@1.0.5(transitive)
+ Addedhas-ansi@2.0.0(transitive)
+ Addedjs-tokens@4.0.0(transitive)
+ Addedloose-envify@1.4.0(transitive)
+ Addedobject-assign@4.1.1(transitive)
+ Addedprop-types@15.8.1(transitive)
+ Addedreact@16.14.0(transitive)
+ Addedreact-is@16.13.1(transitive)
+ Addedstrip-ansi@3.0.1(transitive)
+ Addedsupports-color@2.0.0(transitive)