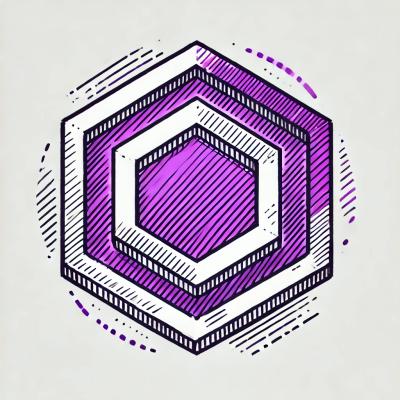
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
@adobe/react-native-acpcore
Advanced tools
@adobe/react-native-acpcore
is a wrapper around the iOS and Android AEP Core SDK to allow for integration with React Native applications. Functionality to enable the Core extension is provided entirely through JavaScript documented below.
You need to install the SDK with npm and configure the native Android/iOS project in your react native project.
Note: If you are new to React Native we suggest you follow the React Native Getting Started page before continuing.
First create a React Native project:
react-native init MyReactApp
Install and link the @adobe/react-native-acpcore
package:
cd MyReactApp
npm install @adobe/react-native-acpcore
react-native link @adobe/react-native-acpcore
Navigate to MainApplication.java
under android/app/src/main/java/com/<project-name>/MainApplication.java
and add a call to MobileCore.setApplication(this)
inside of onCreate()
.
import com.adobe.marketing.mobile.MobileCore; // import MobileCore
@Override
public void onCreate() {
super.onCreate();
//...
MobileCore.setApplication(this); // add this line
}
In the Link Binary With Libraries section, click the + link and add the following frameworks and libraries:
UIKit.framework
SystemConfiguration.framework
WebKit.framework
UserNotifications.framework
libsqlite3.0.tbd
libc++.tbd
libz.tbd
Note: If you plan to use the AEP SDK in your native iOS code you will need to import the appropriate headers with the following format: #import <RCTACPCore/ACPCore.h>
This project contains jest unit tests which are contained in the __tests__
directory, to run the tests locally:
make run-tests-locally
It is recommended to initialize the SDK in your native code inside your AppDelegate and MainApplication in iOS and Android respectively, however you can still initialize the SDK in Javascript.
iOS:
// Import the SDK
#import <RCTACPCore/ACPCore.h>
#import <RCTACPCore/ACPLifecycle.h>
#import <RCTACPCore/ACPIdentity.h>
#import <RCTACPCore/ACPSignal.h>
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
//...
[ACPCore configureWithAppId:@"yourAppId"];
[ACPCore setWrapperType:ACPMobileWrapperTypeReactNative];
[ACPIdentity registerExtension];
[ACPLifecycle registerExtension];
[ACPSignal registerExtension];
[ACPCore start:nil];
}
Android:
@Override
public void onCreate() {
//...
MobileCore.setApplication(this);
MobileCore.configureWithAppID("yourAppId");
MobileCore.setWrapperType(WrapperType.REACT_NATIVE);
try {
Identity.registerExtension();
Lifecycle.registerExtension();
Signal.registerExtension();
} catch (Exception e) {
// handle exception
}
MobileCore.start(null);
}
Javascript:
import {ACPCore, ACPLifecycle, ACPIdentity, ACPSignal, ACPMobileLogLevel} from '@adobe/react-native-acpcore';
initSDK() {
ACPCore.setLogLevel(ACPMobileLogLevel.VERBOSE);
ACPCore.configureWithAppId("yourAppId");
ACPLifecycle.registerExtension();
ACPIdentity.registerExtension();
ACPSignal.registerExtension();
ACPCore.start();
}
ACPCore.updateConfiguration({"yourConfigKey": "yourConfigValue"});
ACPCore.extensionVersion().then(version => console.log("AdobeExperienceSDK: ACPCore version: " + version));
ACPCore.getLogLevel().then(level => console.log("AdobeExperienceSDK: Log Level = " + level));
import {ACPMobileLogLevel} from '@adobe/react-native-acpcore';
ACPCore.setLogLevel(ACPMobileLogLevel.VERBOSE);
import {ACPMobileLogLevel} from '@adobe/react-native-acpcore';
ACPCore.log(ACPMobileLogLevel.ERROR, "React Native Tag", "React Native Message");
Note: ACPMobileLogLevel
contains the following getters:
const ERROR = "ACP_LOG_LEVEL_ERROR";
const WARNING = "ACP_LOG_LEVEL_WARNING";
const DEBUG = "ACP_LOG_LEVEL_DEBUG";
const VERBOSE = "ACP_LOG_LEVEL_VERBOSE";
ACPCore.getPrivacyStatus().then(status => console.log("AdobeExperienceSDK: Privacy Status = " + status));
import {ACPMobilePrivacyStatus} from '@adobe/react-native-acpcore';
ACPCore.setPrivacyStatus(ACPMobilePrivacyStatus.OPT_IN);
Note: ACPMobilePrivacyStatus
contains the following getters:
const OPT_IN = "ACP_PRIVACY_STATUS_OPT_IN";
const OPT_OUT = "ACP_PRIVACY_STATUS_OPT_OUT";
const UNKNOWN = "ACP_PRIVACY_STATUS_UNKNOWN";
ACPCore.getSdkIdentities().then(identities => console.log("AdobeExperienceSDK: Identities = " + identities));
import {ACPExtensionEvent} from '@adobe/react-native-acpcore';
var event = new ACPExtensionEvent("eventName", "eventType", "eventSource", {"testDataKey": "testDataValue"});
ACPCore.dispatchEvent(event);
import {ACPExtensionEvent} from '@adobe/react-native-acpcore';
var event = new ACPExtensionEvent("eventName", "eventType", "eventSource", {"testDataKey": "testDataValue"});
ACPCore.dispatchEventWithResponseCallback(event).then(responseEvent => console.log("AdobeExperienceSDK: responseEvent = " + responseEvent));
import {ACPExtensionEvent} from '@adobe/react-native-acpcore';
var responseEvent = new ACPExtensionEvent("responseEvent", "eventType", "eventSource", {"testDataKey": "testDataValue"});
var requestEvent = new ACPExtensionEvent("requestEvent", "eventType", "eventSource", {"testDataKey": "testDataValue"});
ACPCore.dispatchResponseEvent(responseEvent, requestEvent);
ACPIdentity.extensionVersion().then(version => console.log("AdobeExperienceSDK: ACPIdentity version: " + version));
ACPIdentity.registerExtension();
ACPIdentity.syncIdentifiers({"id1": "identifier1"});
import {ACPMobileVisitorAuthenticationState} from '@adobe/react-native-acpcore';
ACPIdentity.syncIdentifiersWithAuthState({"id1": "identifier1"}, ACPMobileVisitorAuthenticationState.UNKNOWN);
Note: ACPMobileVisitorAuthenticationState
contains the following getters:
const AUTHENTICATED = "ACP_VISITOR_AUTH_STATE_AUTHENTICATED";
const LOGGED_OUT = "ACP_VISITOR_AUTH_STATE_LOGGED_OUT";
const UNKNOWN = "ACP_VISITOR_AUTH_STATE_UNKNOWN";
ACPCore.setAdvertisingIdentifier("adID");
ACPIdentity.appendVisitorInfoForURL("test.com").then(urlWithVisitorData => console.log("AdobeExperienceSDK: VisitorData = " + urlWithVisitorData));
ACPIdentity.getUrlVariables().then(urlVariables => console.log("AdobeExperienceSDK: UrlVariables = " + urlVariables));
ACPIdentity.getIdentifiers().then(identifiers => console.log("AdobeExperienceSDK: Identifiers = " + identifiers));
ACPIdentity.getExperienceCloudId().then(cloudId => console.log("AdobeExperienceSDK: CloudID = " + cloudId));
ACPCore.setPushIdentifier("pushIdentifier");
import {ACPVisitorID} from '@adobe/react-native-acpcore';
var visitorId = new ACPVisitorID(idOrigin?: string, idType: string, id?: string, authenticationState?: ACPMobileVisitorAuthenticationState)
ACPLifecycle.extensionVersion().then(version => console.log("AdobeExperienceSDK: ACPLifecycle version: " + version));
ACPLifecycle.registerExtension();
ACPCore.lifecycleStart({"lifecycleStart": "myData"});
ACPCore.lifecyclePause();
ACPSignal.extensionVersion().then(version => console.log("AdobeExperienceSDK: ACPSignal version: " + version));
ACPSignal.registerExtension();
ACPCore.collectPii({"myPii": "data"});
See CONTRIBUTING
See LICENSE
FAQs
Adobe Experience Platform support for React Native apps.
The npm package @adobe/react-native-acpcore receives a total of 7,743 weekly downloads. As such, @adobe/react-native-acpcore popularity was classified as popular.
We found that @adobe/react-native-acpcore demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 21 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.