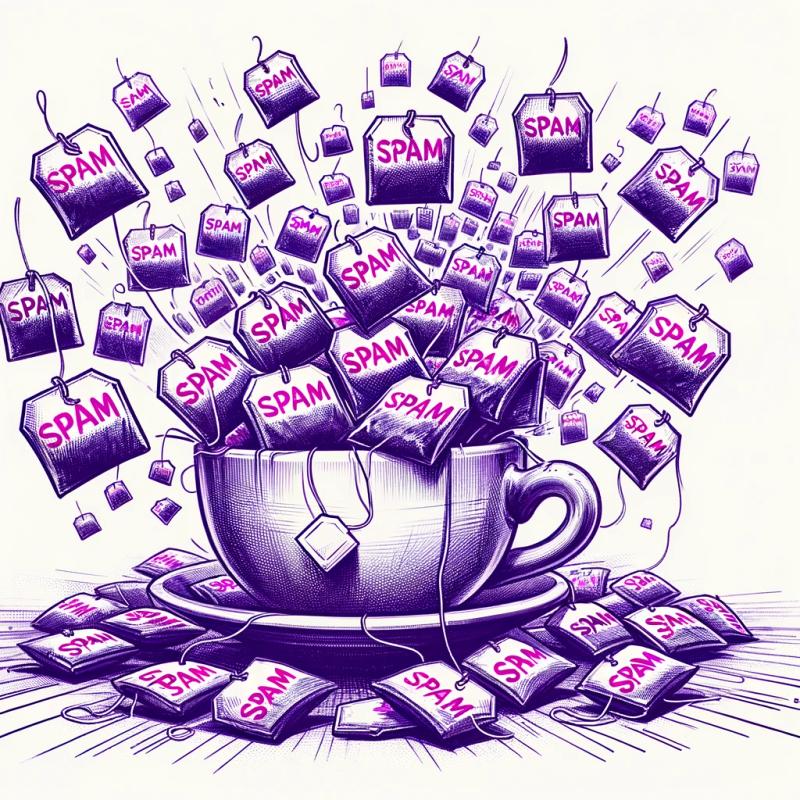
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@ajoelp/toast
Advanced tools
Readme
npm install @ajoelp/toast
Install the toast wrapper in your root app file.
import React from 'react';
import { ToastWrapper } from '@ajoelp/toast';
function App(){
return <ToastWrapper />
}
Trigger toast notifications from anywhere in your application
import { toast } from '@ajoelp/toast';
toast("This is the toast message", {
type: 'success' | 'error' | 'info' | 'warning',
isHtml: true,
delay: 1500
})
// Or use the typed helpers
toast.info("This is the toast message")
toast.success("This is the toast message")
toast.error("This is the toast message")
toast.warning("This is the toast message")
Override the toast message component
import React from 'react';
import { ToastWrapper } from '@ajoelp/toast';
function CustomContainer({ close, message, active, options}){
return (
<p>{message}</p>
)
}
function App(){
return <ToastWrapper toastContainer={CustomContainer} />
}
FAQs
Customizable toast notifications for react
The npm package @ajoelp/toast receives a total of 11 weekly downloads. As such, @ajoelp/toast popularity was classified as not popular.
We found that @ajoelp/toast demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.