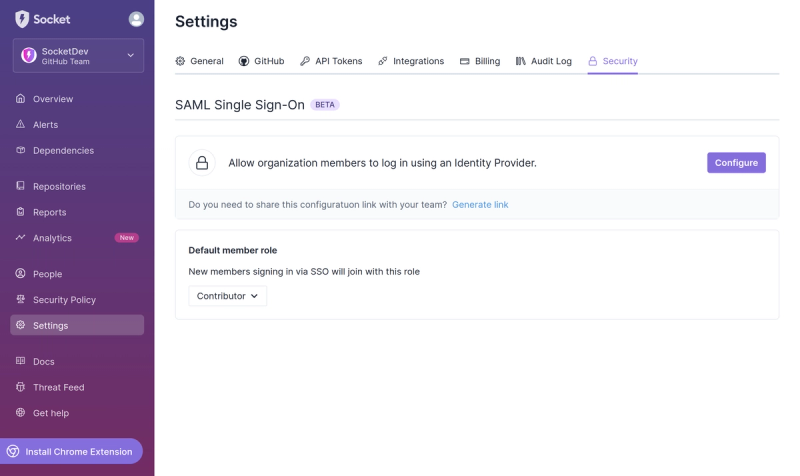
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@akcybex/jstr
Advanced tools
Readme
@akcybex/jstr
is a JavaScript library inspired by Laravel 'Strings' offering a chainable API for streamlined string manipulation and facilitating common string operations in javascript with enhanced expressiveness.
You can install @akcybex/jstr
using npm:
npm i @akcybex/jstr -S
Alternatively, you can use Yarn to add the package:
yarn add @akcybex/jstr
After installing, you can use @akcybex/jstr
in your Node.js application:
import JStr from "@akcybex/jstr";
const result = JStr.of("hello").repeat(3).upper().toString();
console.log(result); // Outputs: 'HELLOHELLOHELLO'
For browser-based projects, you can use the CDN link:
<script src="https://unpkg.com/@akcybex/jstr@x.x.x/dist/jstr.umd.js"></script>
After including the script, jstr
will be available globally:
<script>
const result = JStr.of("hello").repeat(3).upper().toString();
console.log(result); // Outputs: 'HELLOHELLOHELLO'
</script>
[!NOTE]
Methods with ✔✔ mark are included in the library and tested as well.
A string is a fundamental data type in programming used to represent a sequence of characters. It stores and manipulates text data, and string operations are typically performed using methods or functions provided by the programming language or relevant libraries. Common operations include concatenation, searching, replacing, and formatting strings.
JSTR String Methods | Description | Documentation Link |
---|---|---|
✔✔ JStr.after | Returns the portion of the string that comes after a specified substring. | Documentation |
✔✔ JStr.afterLast | Returns the portion of the string that comes after the last occurrence of a specified substring. | Documentation |
✔✔ JStr.ascii | Returns the ASCII representation of the string. | Documentation |
✔✔ JStr.before | Returns the portion of the string that comes before a specified substring. | Documentation |
✔✔ JStr.beforeLast | Returns the portion of the string that comes before the last occurrence of a specified substring. | Documentation |
✔✔ JStr.between | Returns the portion of the string that is between two specified substrings. | Documentation |
✔✔ JStr.betweenFirst | Returns the portion of the string that is between the first occurrence of two specified substrings. | Documentation |
✔✔ JStr.camel | Converts the string to camel case. | Documentation |
✔✔ JStr.charAt | Returns the character at the specified position in the string. | Documentation |
✔✔ JStr.contains | Checks if the string contains a specified substring. | Documentation |
✔✔ JStr.containsAll | Checks if the string contains all of the specified substrings. | Documentation |
✔✔ JStr.endsWith | Checks if the string ends with a specified suffix. | Documentation |
✔ JStr.excerpt | Returns an excerpt from the string with a specified length. | Documentation |
✔✔ JStr.finish | Ensures that the string ends with a specified suffix. | Documentation |
✔✔ JStr.headline | Converts the string to a headline format. | Documentation |
✔✔ JStr.capitalize | Converts the string to a capitalize format. | Documentation |
JStr.inlineMarkdown | Converts the string to inline Markdown format. | Documentation |
✔✔ JStr.is | Checks if the string is equal to a specified value. | Documentation |
✔✔ JStr.isAscii | Checks if the string contains only ASCII characters. | Documentation |
✔✔ JStr.isJson | Checks if the string is a valid JSON format. | Documentation |
✔ JStr.isUlid | Checks if the string is a valid ULID (Universally Unique Lexicographically Sortable Identifier). | Documentation |
✔✔ JStr.isUrl | Checks if the string is a valid URL. | Documentation |
✔ JStr.isUuid | Checks if the string is a valid UUID (Universally Unique Identifier). | Documentation |
✔✔ JStr.kebab | Converts the string to kebab case. | Documentation |
✔✔ JStr.lcfirst | Converts the first character of the string to lowercase. | Documentation |
✔✔ JStr.length | Returns the length of the string. | Documentation |
✔✔ JStr.limit | Limits the length of the string to a specified number of characters. | Documentation |
✔✔ JStr.lower | Converts the string to lowercase. | Documentation |
✔✔ JStr.ltrim | Removes whitespace from the beginning of the string. | Documentation |
JStr.markdown | Converts the string to Markdown format. | Documentation |
✔✔ JStr.mask | Masks part of the string with a specified character. | Documentation |
✔✔ JStr.match | Performs a regular expression match on the string. | Documentation |
✔✔ JStr.matchAll | Performs a global regular expression match on the string. | Documentation |
✔✔ JStr.isMatch | Checks if the string matches a specified pattern. | Documentation |
JStr.orderedUuid | Generates an ordered UUID (Universally Unique Identifier). | Documentation |
✔✔ JStr.padBoth | Pads the string on both sides with a specified character to a specified length. | Documentation |
✔✔ JStr.padLeft | Pads the string on the left side with a specified character to a specified length. | Documentation |
✔✔ JStr.padRight | Pads the string on the right side with a specified character to a specified length. | Documentation |
✔✔ JStr.password | Generates a password string. | Documentation |
✔ JStr.plural | Converts the string to plural form. | Documentation |
✔ JStr.pluralStudly | Converts the string to plural studly form. | Documentation |
✔✔ JStr.position | Finds the position of the first occurrence of a substring in the string. | Documentation |
✔✔ JStr.random | Generates a random string. | Documentation |
✔✔ JStr.remove | Removes a specified substring from the string. | Documentation |
✔✔ JStr.repeat | Repeats the string a specified number of times. | Documentation |
✔✔ JStr.replace | Replaces occurrences of a specified substring with another substring. | Documentation |
✔✔ JStr.replaceArray | Replaces occurrences of specified substrings with corresponding replacements. | Documentation |
✔✔ JStr.replaceFirst | Replaces the first occurrence of a specified substring with another substring. | Documentation |
✔✔ JStr.replaceLast | Replaces the last occurrence of a specified substring with another substring. | Documentation |
✔✔ JStr.rtrim | Removes whitespace from the end of the string. | Documentation |
✔✔ JStr.reverse | Reverses the characters of the string. | Documentation |
✔ JStr.singular | Converts the string to singular form. | Documentation |
✔ JStr.slug | Converts the string to a URL-friendly slug. | Documentation |
✔✔ JStr.snake | Converts the string to snake case. | Documentation |
✔ JStr.squish | Reduces multiple consecutive whitespace characters to a single space. | Documentation |
✔✔ JStr.start | Adds a specified prefix to the beginning of the string. | Documentation |
✔ JStr.startsWith | Checks if the string starts with a specified prefix. | Documentation |
✔✔ JStr.studly | Converts the string to studly case. | Documentation |
✔ JStr.substr | Returns a substring of the string starting from a specified position. | Documentation |
✔ JStr.substrCount | Counts the number of occurrences of a substring in the string. | Documentation |
✔ JStr.substrReplace | Replaces a portion of the string with a specified substring. | Documentation |
✔ JStr.swap | Swaps the case of each character in the string. | Documentation |
✔ JStr.take | Returns the first n characters from the string. | Documentation |
✔ JStr.title | Converts the first character of each word in the string to uppercase. | Documentation |
✔✔ JStr.trim | Removes whitespace from the beginning and end of the string. | Documentation |
JStr.toHtmlString | Converts the string to its HTML-encoded representation. | Documentation |
✔✔ JStr.ucfirst | Converts the first character of the string to uppercase. | Documentation |
✔ JStr.ucsplit | Splits the string into an array of words. | Documentation |
✔✔ JStr.upper | Converts the string to uppercase. | Documentation |
JStr.ulid | Generates a ULID (Universally Unique Lexicographically Sortable Identifier). | Documentation |
✔✔ JStr.uuid | Generates a UUID (Universally Unique Identifier). | Documentation |
✔ JStr.wordCount | Counts the number of words in the string. | Documentation |
✔ JStr.wordWrap | Wraps the string at a specified length with a specified line ending. | Documentation |
✔ JStr.words | Splits the string into an array of words. | Documentation |
✔ JStr.wrap | Wraps the string at a specified length with a specified string. | Documentation |
Fluent strings provide a more fluent, object-oriented interface for working with string values. This paradigm allows you to chain multiple string operations together, creating a more readable syntax compared to traditional string operations. Each method call returns a new fluent string object, enabling you to seamlessly chain operations while maintaining clarity and conciseness in your code.
JSTR Fluent String Methods | Description | Documentation Link |
---|---|---|
✔✔ after | Returns the portion of the string that comes after a specified substring. | Documentation |
✔✔ afterLast | Returns the portion of the string that comes after the last occurrence of a specified substring. | Documentation |
✔ append | Appends a string or an array of strings to the end of the current string. | Documentation |
✔✔ ascii | Returns the ASCII representation of the string. | Documentation |
basename | Returns the trailing name component of a path. | Documentation |
✔✔ before | Returns the portion of the string that comes before a specified substring. | Documentation |
✔✔ beforeLast | Returns the portion of the string that comes before the last occurrence of a specified substring. | Documentation |
✔✔ between | Returns the portion of the string that is between two specified substrings. | Documentation |
✔✔ betweenFirst | Returns the portion of the string that is between the first occurrence of two specified substrings. | Documentation |
✔✔ camel | Converts the string to camel case. | Documentation |
✔✔ charAt | Returns the character at the specified position in the string. | Documentation |
classBasename | Returns the class basename of a fully qualified class name. | Documentation |
✔✔ contains | Checks if the string contains a specified substring. | Documentation |
✔✔ containsAll | Checks if the string contains all of the specified substrings. | Documentation |
dirname | Returns the directory name component of a path. | Documentation |
✔✔ endsWith | Checks if the string ends with a specified suffix. | Documentation |
✔ excerpt | Returns an excerpt from the string with a specified length. | Documentation |
✔ exactly | Checks if the string is exactly equal to a specified value. | Documentation |
✔ explode | Splits the string by a specified delimiter and returns an array of the parts. | Documentation |
✔✔ finish | Ensures that the string ends with a specified suffix. | Documentation |
✔✔ headline | Converts the string to a headline format. | Documentation |
✔✔ capitalize | Converts the string to a capitalize format. | Documentation |
inlineMarkdown | Converts the string to inline Markdown format. | Documentation |
✔✔ is | Checks if the string is equal to a specified value. | Documentation |
✔✔ isAscii | Checks if the string contains only ASCII characters. | Documentation |
✔✔ isEmpty | Checks if the string is empty. | Documentation |
✔ isNotEmpty | Checks if the string is not empty. | Documentation |
✔✔ isJson | Checks if the string is a valid JSON format. | Documentation |
✔ isUlid | Checks if the string is a valid ULID (Universally Unique Lexicographically Sortable Identifier). | Documentation |
✔✔ isUrl | Checks if the string is a valid URL. | Documentation |
✔ isUuid | Checks if the string is a valid UUID (Universally Unique Identifier). | Documentation |
✔✔ kebab | Converts the string to kebab case. | Documentation |
✔✔ lcfirst | Converts the first character of the string to lowercase. | Documentation |
✔✔ length | Returns the length of the string. | Documentation |
✔✔ limit | Limits the length of the string to a specified number of characters. | Documentation |
✔✔ lower | Converts the string to lowercase. | Documentation |
✔✔ ltrim | Removes whitespace from the beginning of the string. | Documentation |
markdown | Converts the string to Markdown format. | Documentation |
✔✔ mask | Masks part of the string with a specified character. | Documentation |
✔✔ match | Performs a regular expression match on the string. | Documentation |
✔✔ matchAll | Performs a global regular expression match on the string. | Documentation |
✔✔ isMatch | Checks if the string matches a specified pattern. | Documentation |
✔ newLine | Replaces each occurrence of a newline character with a specified string. | Documentation |
✔✔ padBoth | Pads the string on both sides with a specified character to a specified length. | Documentation |
✔✔ padLeft | Pads the string on the left side with a specified character to a specified length. | Documentation |
✔✔ padRight | Pads the string on the right side with a specified character to a specified length. | Documentation |
✔ pipe | Passes the string to a callback and returns the result. | Documentation |
✔ plural | Converts the string to plural form. | Documentation |
✔✔ position | Finds the position of the first occurrence of a substring in the string. | Documentation |
✔ prepend | Prepends a string or an array of strings to the beginning of the current string. | Documentation |
✔✔ remove | Removes a specified substring from the string. | Documentation |
✔✔ repeat | Repeats the string a specified number of times. | Documentation |
✔✔ replace | Replaces occurrences of a specified substring with another substring. | Documentation |
✔✔ replaceArray | Replaces occurrences of specified substrings with corresponding replacements. | Documentation |
✔✔ replaceFirst | Replaces the first occurrence of a specified substring with another substring. | Documentation |
✔✔ replaceLast | Replaces the last occurrence of a specified substring with another substring. | Documentation |
✔ replaceMatches | Replaces occurrences of a specified pattern with a callback result. | Documentation |
✔✔ reverse | Reverses the characters of the string. | Documentation |
✔✔ rtrim | Removes whitespace from the end of the string. | Documentation |
✔ scan | Returns an array of all occurrences of a regular expression pattern in the string. | Documentation |
✔ singular | Converts the string to singular form. | Documentation |
✔ slug | Converts the string to a URL-friendly slug. | Documentation |
✔✔ snake | Converts the string to snake case. | Documentation |
✔ split | Splits the string by a specified delimiter and returns an array of the parts. | Documentation |
✔ squish | Reduces multiple consecutive whitespace characters to a single space. | Documentation |
✔✔ start | Adds a specified prefix to the beginning of the string. | Documentation |
✔ startsWith | Checks if the string starts with a specified prefix. | Documentation |
✔✔ studly | Converts the string to studly case. | Documentation |
✔ pluralStudy | Converts the string to studly case. | Documentation |
✔ substr | Returns a substring of the string starting from a specified position. | Documentation |
✔ substrCount | Counts the number of occurrences of a substring in the string. | Documentation |
✔ substrReplace | Replaces a portion of the string with a specified substring. | Documentation |
✔ swap | Swaps the case of each character in the string. | Documentation |
✔ take | Returns the first n characters from the string. | Documentation |
✔ tap | Passes the string to a callback and returns the string. | Documentation |
✔ test | Performs a regular expression match on the string and returns a boolean. | Documentation |
✔✔ title | Converts the first character of each word in the string to uppercase. | Documentation |
✔✔ trim | Removes whitespace from the beginning and end of the string. | Documentation |
✔✔ ucfirst | Converts the first character of the string to uppercase. | Documentation |
✔ ucsplit | Splits the string into an array of words. | Documentation |
✔✔ upper | Converts the string to uppercase. | Documentation |
✔ when | Executes a callback if a given condition is true. | Documentation |
whenContains | Executes a callback if the string contains a specified substring. | Documentation |
whenContainsAll | Executes a callback if the string contains all of the specified substrings. | Documentation |
whenEmpty | Executes a callback if the string is empty. | Documentation |
whenNotEmpty | Executes a callback if the string is not empty. | Documentation |
whenStartsWith | Executes a callback if the string starts with a specified prefix. | Documentation |
whenEndsWith | Executes a callback if the string ends with a specified suffix. | Documentation |
whenExactly | Executes a callback if the string is exactly equal to a specified value. | Documentation |
whenNotExactly | Executes a callback if the string is not | Documentation |
whenIs | Executes a callback if the string is equal to a specified value. | Documentation |
whenIsAscii | Executes a callback if the string contains only ASCII characters. | Documentation |
whenIsUlid | Executes a callback if the string is a valid ULID. | Documentation |
whenIsUuid | Executes a callback if the string is a valid UUID. | Documentation |
whenTest | Executes a callback if a regular expression test on the string is true. | Documentation |
✔ wordCount | Counts the number of words in the string. | Documentation |
✔ words | Splits the string into an array of words. | Documentation |
✔ wordWrap | Wraps the string at a specified length with a specified line ending. | Documentation |
Contributions to @akcybex/jstr
are welcome. Please refer to the contributing guidelines for more information.
This project is licensed under the MIT License.
FAQs
`@akcybex/jstr` is a JavaScript library inspired by [Laravel 'Strings'](https://laravel.com/docs/10.x/strings) offering a chainable API for streamlined string manipulation and facilitating common string operations in javascript with enhanced expressivenes
The npm package @akcybex/jstr receives a total of 2 weekly downloads. As such, @akcybex/jstr popularity was classified as not popular.
We found that @akcybex/jstr demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.