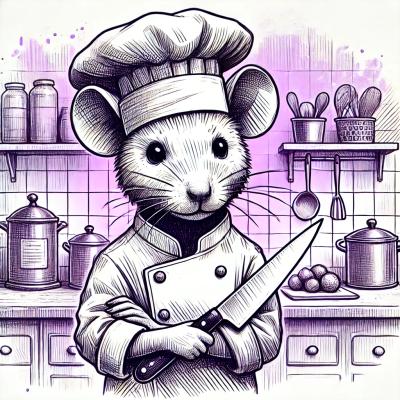
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@alexlafroscia/postcss-color-mod-function
Advanced tools
Modify colors using the color-mod() function in CSS
PostCSS color-mod() Function lets you modify colors using the color-mod()
function in CSS, following the outdated version of CSS Color Module Level 4 specification (05 July 2016).
⚠️ color-mod()
has been removed from Color Module Level 4 specification. (Here's why)
:root {
--brand-red: color-mod(yellow blend(red 50%));
--brand-red-hsl: color-mod(yellow blend(red 50% hsl));
--brand-red-hwb: color-mod(yellow blend(red 50% hwb));
--brand-red-dark: color-mod(red blackness(20%));
}
/* becomes */
:root {
--brand-red: rgb(255, 127.5, 0);
--brand-red-hsl: rgb(255, 127.5, 255);
--brand-red-hwb: rgb(255, 127.5, 0);
--brand-red-dark: rgb(204, 0, 0);
}
/* or, using stringifier(color) { return color.toString() } */
:root {
--brand-red: rgb(100% 50% 0% / 100%);
--brand-red-hsl: hsl(30 100% 50% / 100%);
--brand-red-hwb: hwb(30 0% 0% / 100%);
--brand-red-dark: hwb(0 0% 20% / 100%);
}
The color-mod()
function accepts rgb()
, legacy comma-separated rgb()
,
rgba()
, hsl()
, legacy comma-separated hsl()
, hsla()
, hwb()
, and
color-mod()
colors, as well as 3, 4, 6, and 8 digit hex colors, and named
colors without the need for additional plugins.
Implemention details are available in the specification.
The color-mod()
function accepts red()
, green()
, blue()
, a()
/
alpha()
, rgb()
, h()
/ hue()
, s()
/ saturation()
, l()
/
lightness()
, w()
/ whiteness()
, b()
/ blackness()
, tint()
,
shade()
, blend()
, blenda()
, and contrast()
color adjusters.
Implemention details are available in the specification.
By default, var()
variables will be used if their corresponding Custom
Properties are found in a :root
rule, or if a fallback value is specified.
Add PostCSS color-mod() Function to your project:
npm install postcss postcss-color-mod-function --save-dev
Use PostCSS color-mod() Function to process your CSS:
const postcssColorMod = require('postcss-color-mod-function');
postcssColorMod.process(YOUR_CSS /*, processOptions, pluginOptions */);
Or use it as a PostCSS plugin:
const postcss = require('postcss');
const postcssColorMod = require('postcss-color-mod-function');
postcss([
postcssColorMod(/* pluginOptions */)
]).process(YOUR_CSS /*, processOptions */);
PostCSS color-mod() Function runs in all Node environments, with special instructions for:
Node | PostCSS CLI | Webpack | Create React App | Gulp | Grunt |
---|
The stringifier
option defines how transformed colors will be produced in CSS.
By default, legacy rgb()
and rgba()
colors are produced, but this can be
easily updated to support [CSS Color Module Level 4 colors] colors.
import postcssColorMod from 'postcss-color-mod-function';
postcssColorMod({
stringifier(color) {
return color.toString(); // use CSS Color Module Level 4 colors (rgb, hsl, hwb)
}
});
Future major releases of PostCSS color-mod() Function may reverse this functionality so that CSS Color Module Level 4 colors are produced by default.
The unresolved
option defines how unresolved functions and arguments should
be handled. The available options are throw
, warn
, and ignore
. The
default option is to throw
.
If ignore
is used, the color-mod()
function will remain unchanged.
import postcssColorMod from 'postcss-color-mod-function';
postcssColorMod({
unresolved: 'ignore' // ignore unresolved color-mod() functions
});
The transformVars
option defines whether var()
variables used within
color-mod()
should be transformed into their corresponding Custom Properties
available in :root
, or their fallback value if it is specified. By default,
var()
variables will be transformed.
However, because these transformations occur at build time, they cannot be considered accurate. Accurately resolving cascading variables relies on knowledge of the living DOM tree.
The importFrom
option allows you to import variables from other sources,
which might be CSS, JS, and JSON files, and directly passed objects.
postcssColorMod({
importFrom: 'path/to/file.css' // :root { --brand-dark: blue; --brand-main: var(--brand-dark); }
});
.brand-faded {
color: color-mod(var(--brand-main) a(50%));
}
/* becomes */
.brand-faded {
color: rgba(0, 0, 255, .5);
}
Multiple files can be passed into this option, and they will be parsed in the
order they were received. JavaScript files, JSON files, and objects will need
to namespace custom properties under a customProperties
or
custom-properties
key.
postcssColorMod({
importFrom: [
'path/to/file.css', // :root { --brand-dark: blue; --brand-main: var(--brand-dark); }
'and/then/this.js', // module.exports = { customProperties: { '--brand-dark': 'blue', '--brand-main': 'var(--brand-dark)' } }
'and/then/that.json', // { "custom-properties": { "--brand-dark": "blue", "--brand-main": "var(--brand-dark)" } }
{
customProperties: {
'--brand-dark': 'blue',
'--brand-main': 'var(--brand-dark)'
}
}
]
});
Variables may reference other variables, and this plugin will attempt to
resolve them. If transformVars
is set to false
then importFrom
will not
be used.
4.0.0 (October 18, 2024)
FAQs
Modify colors using the color-mod() function in CSS
The npm package @alexlafroscia/postcss-color-mod-function receives a total of 128 weekly downloads. As such, @alexlafroscia/postcss-color-mod-function popularity was classified as not popular.
We found that @alexlafroscia/postcss-color-mod-function demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.