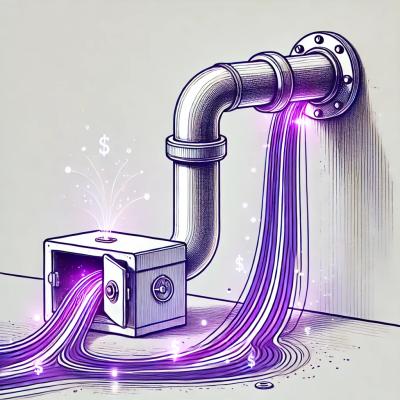
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@alitajs/cordova-native
Advanced tools
由于这个包不常用,又和
native
冲突,因此将这个包改名为@alitajs/cordova-native
,相应的配置和命令也修改为cordovanative
。
camera
, device
, dialogs
, file
, geolocation
, inappbrowser
, media
, media-capture
, keyboard
, secure-storage
, network
, screen-orientation
, statusbar
, vibration
, document-viewer
, file-opener
, fingerprint-aio
, native-storage
, qr-scanner
, sqlite-storage
,
config/config.ts
export default {
appType: 'cordova',
cordovanative: ['file', 'device', 'camera', 'qr-scanner'], // 数组里的名字只能用上面的插件名
};
在项目根目录运行
alita cordovanative
相机组件,可用来拍照等
对应的 cordova
插件: cordova-plugin-camera
config.xml
配置
<edit-config target="NSCameraUsageDescription" file="*-Info.plist" mode="merge">
<string>need camera access to take pictures</string>
</edit-config>
<edit-config target="NSPhotoLibraryUsageDescription" file="*-Info.plist" mode="merge">
<string>need photo library access to get pictures from there</string>
</edit-config>
<edit-config target="NSLocationWhenInUseUsageDescription" file="*-Info.plist" mode="merge">
<string>need location access to find things nearby</string>
</edit-config>
<edit-config target="NSPhotoLibraryAddUsageDescription" file="*-Info.plist" mode="merge">
<string>need photo library access to save pictures there</string>
</edit-config>
用法
import { Camera, CameraOptions } from '@ionic-native/camera';
const options: CameraOptions = {
quality: 100,
destinationType: Camera.DestinationType.FILE_URI,
encodingType: Camera.EncodingType.JPEG,
mediaType: Camera.MediaType.PICTURE,
};
Camera.getPicture(options).then(
(imageData) => {
// imageData is either a base64 encoded string or a file URI
// If it's base64 (DATA_URL):
let base64Image = 'data:image/jpeg;base64,' + imageData;
},
(err) => {
// Handle error
},
);
用于获取设备信息,比如 platform, uuid, version 等
对应的 cordova
插件: cordova-plugin-device
用法
import { Device } from '@ionic-native/device';
...
console.log('Device UUID is: ' + Device.uuid);
弹窗组件
对应的 cordova
插件: cordova-plugin-dialogs
用法
import { Dialogs } from '@ionic-native/dialogs';
Dialogs.alert('Hello world')
.then(() => console.log('Dialog dismissed'))
.catch((e) => console.log('Error displaying dialog', e));
文件组件,用于读写原生文件系统
对应的 cordova
插件: cordova-plugin-file
用法
import { File } from '@ionic-native/file';
File.checkDir(File.dataDirectory, 'mydir').then(_ => console.log('Directory exists')).catch(err =>
console.log('Directory doesn't exist'));
调原生方法加密保存数据
对应的 cordova
插件: cordova-plugin-secure-storage
config.xml
配置
<platform name="ios">
<preference name="KeychainAccessibility" value="WhenUnlocked"/>
</platform>
支持的配置:
AfterFirstUnlock
AfterFirstUnlockThisDeviceOnly
WhenUnlocked (default)
WhenUnlockedThisDeviceOnly
WhenPasscodeSetThisDeviceOnly (this option is available only on iOS8 and later)
用法
import {
SecureStorage,
SecureStorageObject,
} from '@ionic-native/secure-storage';
SecureStorage.create('my_store_name').then((storage: SecureStorageObject) => {
storage.get('key').then(
(data) => console.log(data),
(error) => console.log(error),
);
storage.set('key', 'value').then(
(data) => console.log(data),
(error) => console.log(error),
);
storage.remove('key').then(
(data) => console.log(data),
(error) => console.log(error),
);
});
获取当前位置组件
对应的 cordova
插件: cordova-plugin-geolocation
<edit-config target="NSLocationWhenInUseUsageDescription" file="*-Info.plist" mode="merge">
<string>need location access to find things nearby</string>
</edit-config>
用法
import { Geolocation } from '@ionic-native/geolocation';
Geolocation.getCurrentPosition()
.then((resp) => {
// resp.coords.latitude
// resp.coords.longitude
})
.catch((error) => {
console.log('Error getting location', error);
});
let watch = Geolocation.watchPosition();
watch.subscribe((data) => {
// data can be a set of coordinates, or an error (if an error occurred).
// data.coords.latitude
// data.coords.longitude
});
应用内浏览器组件
对应的 cordova
插件: cordova-plugin-inappbrowser
import { InAppBrowser } from '@ionic-native/in-app-browser';
const browser = InAppBrowser.create('https://ionicframework.com/');
browser.executeScript(...);
browser.insertCSS(...);
browser.on('loadstop').subscribe(event => {
browser.insertCSS({ code: "body{color: red;" });
});
browser.close();
音频播放、录制组件
对应的 cordova
插件: cordova-plugin-media
用法
import { Media, MediaObject } from '@ionic-native/media';
// Create a Media instance. Expects path to file or url as argument
// We can optionally pass a second argument to track the status of the media
const file: MediaObject = Media.create('file.mp3');
// to listen to plugin events:
file.onStatusUpdate.subscribe((status) => console.log(status)); // fires when file status changes
file.onSuccess.subscribe(() => console.log('Action is successful'));
file.onError.subscribe((error) => console.log('Error!', error));
// play the file
file.play();
// pause the file
file.pause();
// get current playback position
file.getCurrentPosition().then((position) => {
console.log(position);
});
// get file duration
let duration = file.getDuration();
console.log(duration);
// skip to 10 seconds (expects int value in ms)
file.seekTo(10000);
// stop playing the file
file.stop();
// release the native audio resource
// Platform Quirks:
// iOS simply create a new instance and the old one will be overwritten
// Android you must call release() to destroy instances of media when you are done
file.release();
// Recording to a file
const file: MediaObject = Media.create('path/to/file.mp3');
file.startRecord();
file.stopRecord();
照片、音频、视频录制组件
对应的 cordova
插件: cordova-plugin-media-capture
<edit-config target="NSCameraUsageDescription" file="*-Info.plist" mode="merge">
<string>need camera access to take pictures</string>
</edit-config>
<edit-config target="NSMicrophoneUsageDescription" file="*-Info.plist" mode="merge">
<string>need microphone access to record sounds</string>
</edit-config>
<edit-config target="NSPhotoLibraryUsageDescription" file="*-Info.plist" mode="merge">
<string>need to photo library access to get pictures from there</string>
</edit-config>
用法
import {
MediaCapture,
MediaFile,
CaptureError,
CaptureImageOptions,
} from '@ionic-native/media-capture';
let options: CaptureImageOptions = { limit: 3 };
MediaCapture.captureImage(options).then(
(data: MediaFile[]) => console.log(data),
(err: CaptureError) => console.error(err),
);
键盘插件,支持键盘弹起或隐藏,需要安装 ionic webview
对应的 cordova
插件: cordova-plugin-ionic-keyboard
用法
import { Keyboard } from '@ionic-native/keyboard';
Keyboard.show();
Keyboard.hide();
网络连接监听插件,可以获取网络连接状态,连接变化
对应的 cordova
插件: cordova-plugin-network-information
用法
import { Network } from '@ionic-native/network';
// watch network for a disconnection
let disconnectSubscription = Network.onDisconnect().subscribe(() => {
console.log('network was disconnected :-(');
});
// stop disconnect watch
disconnectSubscription.unsubscribe();
// watch network for a connection
let connectSubscription = Network.onConnect().subscribe(() => {
console.log('network connected!');
// We just got a connection but we need to wait briefly
// before we determine the connection type. Might need to wait.
// prior to doing any api requests as well.
setTimeout(() => {
if (Network.type === 'wifi') {
console.log('we got a wifi connection, woohoo!');
}
}, 3000);
});
// stop connect watch
connectSubscription.unsubscribe();
控制屏幕旋转
对应的 cordova
插件: cordova-plugin-screen-orientation
用法
import { ScreenOrientation } from '@ionic-native/screen-orientation';
// get current
console.log(ScreenOrientation.type); // logs the current orientation, example: 'landscape'
// set to landscape
ScreenOrientation.lock(ScreenOrientation.ORIENTATIONS.LANDSCAPE);
// allow user rotate
ScreenOrientation.unlock();
// detect orientation changes
ScreenOrientation.onChange().subscribe(() => {
console.log('Orientation Changed');
});
状态栏组件
对应的 cordova
插件: cordova-plugin-statusbar
import { StatusBar } from '@ionic-native/status-bar';
// let status bar overlay webview
StatusBar.overlaysWebView(true);
// set status bar to white
StatusBar.backgroundColorByHexString('#ffffff');
震动控制
对应的 cordova
插件: cordova-plugin-vibration
用法
import { Vibration } from '@ionic-native/vibration';
// Vibrate the device for a second
// Duration is ignored on iOS.
Vibration.vibrate(1000);
// Vibrate 2 seconds
// Pause for 1 second
// Vibrate for 2 seconds
// Patterns work on Android and Windows only
Vibration.vibrate([2000, 1000, 2000]);
// Stop any current vibrations immediately
// Works on Android and Windows only
Vibration.vibrate(0);
使用系统默认软件打开文件
对应的 cordova
插件: cordova-plugin-file-opener2
用法
import { FileOpener } from '@ionic-native/file-opener';
FileOpener.open('path/to/file.pdf', 'application/pdf')
.then(() => console.log('File is opened'))
.catch((e) => console.log('Error opening file', e));
FileOpener.showOpenWithDialog('path/to/file.pdf', 'application/pdf')
.then(() => console.log('File is opened'))
.catch((e) => console.log('Error opening file', e));
pdf 查看组件
对应的 cordova
插件: cordova-plugin-document-viewer
用法
import {
DocumentViewer,
DocumentViewerOptions,
} from '@ionic-native/document-viewer';
const options: DocumentViewerOptions = {
title: 'My PDF',
};
DocumentViewer.viewDocument('assets/myFile.pdf', 'application/pdf', options);
生物识别组件
对应的 cordova
插件: cordova-plugin-fingerprint-aio
<preference name="UseSwiftLanguageVersion" value="4.0" />
,在 config.xml 里设置 <preference name="UseSwiftLanguageVersion" value="4.0" />
用法
import { FingerprintAIO } from '@ionic-native/fingerprint-aio';
FingerprintAIO.show({
clientId: 'Fingerprint-Demo', //Android: Used for encryption. iOS: used for dialogue if no `localizedReason` is given.
clientSecret: 'o7aoOMYUbyxaD23oFAnJ' //Necessary for Android encrpytion of keys. Use random secret key.
disableBackup:true, //Only for Android(optional)
localizedFallbackTitle: 'Use Pin', //Only for iOS
localizedReason: 'Please authenticate' //Only for iOS
})
.then((result: any) => console.log(result))
.catch((error: any) => console.log(error));
使用原生存储,Sharedpreferences
in Android and NSUserDefaults
in iOS.
对应的 cordova
插件: cordova-plugin-nativestorage
用法
import { NativeStorage } from '@ionic-native/native-storage';
NativeStorage.setItem('myitem', {
property: 'value',
anotherProperty: 'anotherValue',
}).then(
() => console.log('Stored item!'),
(error) => console.error('Error storing item', error),
);
NativeStorage.getItem('myitem').then(
(data) => console.log(data),
(error) => console.error(error),
);
二维码识别组件
对应的 cordova
插件: cordova-plugin-qrscanner
用法
import { QRScanner, QRScannerStatus } from '@ionic-native/qr-scanner';
// Optionally request the permission early
QRScanner.prepare()
.then((status: QRScannerStatus) => {
if (status.authorized) {
// camera permission was granted
// start scanning
let scanSub = QRScanner.scan().subscribe((text: string) => {
console.log('Scanned something', text);
QRScanner.hide(); // hide camera preview
scanSub.unsubscribe(); // stop scanning
});
} else if (status.denied) {
// camera permission was permanently denied
// you must use QRScanner.openSettings() method to guide the user to the settings page
// then they can grant the permission from there
} else {
// permission was denied, but not permanently. You can ask for permission again at a later time.
}
})
.catch((e: any) => console.log('Error is', e));
sqlite 数据库插件
对应的 cordova
插件: cordova-sqlite-storage
用法
import { SQLite, SQLiteObject } from '@ionic-native/sqlite';
SQLite.create({
name: 'data.db',
location: 'default',
})
.then((db: SQLiteObject) => {
db.executeSql('create table danceMoves(name VARCHAR(32))', [])
.then(() => console.log('Executed SQL'))
.catch((e) => console.log(e));
})
.catch((e) => console.log(e));
FAQs
@alitajs/cordova-native
We found that @alitajs/cordova-native demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.