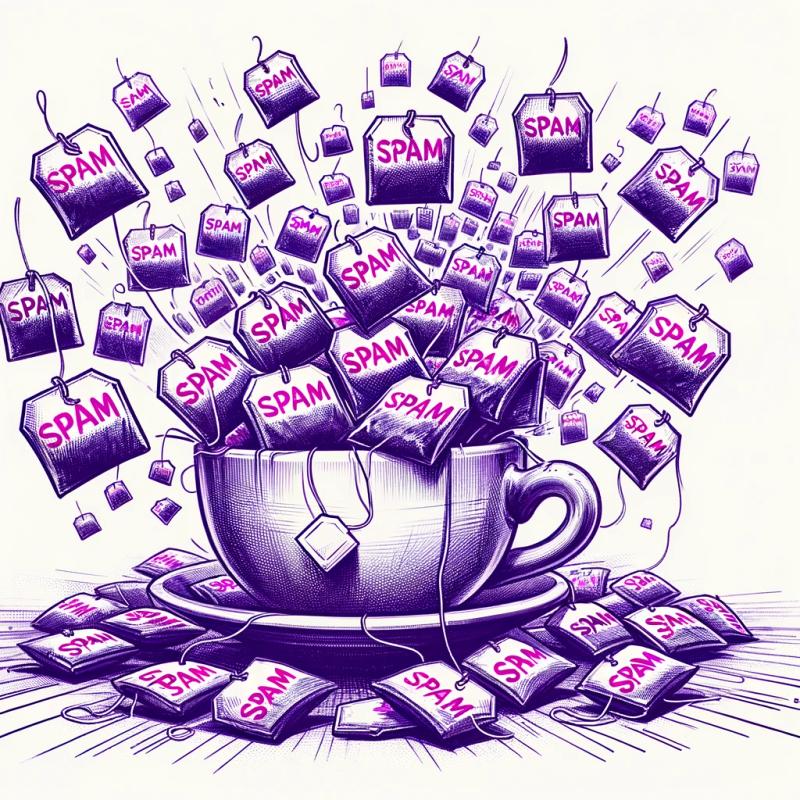
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@allianceblock/abdex-sdk-v2
Advanced tools
This SDK is used to provide all needed functionality and comunication with the ABDEX Smart contrcts. > The main export from this SDK is the `async function initSDK`.
Readme
This SDK is used to provide all needed functionality and comunication with the ABDEX Smart contrcts.
The main export from this SDK is the
async function initSDK
.
Wallet
object with attached JsonRpcProvider
Pool,
DEX,
UserContribution
const sdk = await initSDK(signerWithProvider)
async function initSDK
const pool = new sdk.Pool(poolTokenA, poolTokenB, weight)
BigNumber
and is the weight in the virtual pairs inside the ABDEX LP usually like so: const weight = BigNumber.from('750000000000000000')
public contract: Contract;
public token1: Token;
public token0: Token;
public weight: BigNumber;
async getLiquidity(address: string): Promise<TokenAmount>
- returns the balance of the ALBT LP token for the given addressasync getTotalSupply(): Promise<TokenAmount>
- returns the total supply of ALBT LP tokensasync getReserves(): Promise<TokenAmount[] | any[]>
returns the reserve amoutns for both of the tokens inside the LPpublic involvesToken(token: Token): boolean
- a util function to check if the Pool consist of the given token token.public oppositeToken(token: Token): Token
- returns the other token in the Poolpublic getLiquidityValue(reserve: TokenAmount, totalSupply: TokenAmount,liquidity: TokenAmount): TokenAmount
- returns the liquidity for a given token amount. This method is ment to be used only by the class UserContribution
async function initSDK
const userPool = new sdk.UserContribution(pool);
public pool: Pool;
public userAddress: string;
tokenAisWrappedNativeToken(): Boolean
- checks if tokenA in the Pool
is nateivetokenBisWrappedNativeToken(): Boolean
- checks if tokenB in the Pool
is nateiveasync getUserDeposit(): Promise<TokenAmount[]>
- returns the user deposited token amountsasync getUserShare(): Promise<Percent | undefined>
- returns the user % in the LPasync liquidity(): Promise<TokenAmount>
- returns the balance of the ALBT LP token for the userAddress
async function initSDK
const treasury = new sdk.Treasury(treasuryAddress,token0,token1);
function getTreasuryAddress(
dex.orchestratorTreasuryAddress, // dex is a DEX object
token0,
token1
)
public contract: Contract;
public token0: Token;
public token1: Token;
public signer: Wallet;
async approveTokenAmount(signer: Wallet, tokenAmount: TokenAmount)
- utility method that approves token to be used in treasury.invest(...)
methodasync invest(tokenAmount: TokenAmount, signerWithProvider: Wallet)
- used to invest tokens in the treasuryasync investWithPermit(tokenAmount: TokenAmount, onBehalf: Wallet, deadline: number, v, r, s)
- same as invest
but with permitasync approve(spenderAddress: string)
- approves addres in the treasury contractasync allowance(owner: Wallet, spender: string)
- inspects if the address is approvedsync balanceOf(investor: Wallet, token: Token)
- returns the user investment of the given tokenasync totalInvestments(token: Token)
- returns the total investment of the given tokeninstantiated from the async function initSDK
constriction: const dex = new sdk.DEX();
DEX methods:
async getQuote(pool: Pool, tokenAmount: TokenAmount): Promise<TokenAmount>
- returns a quote amount of the oposite token, used when adding liquidity to LP.
async addLiquidity(tokenAmountA: TokenAmount, tokenAmountB: TokenAmount, pool: Pool, slippageTolerance: number = 50)
- provide liquidity to existing LP or create LP with the given tokens. Returns a populated transaction.
async addNativeLiquidity(currencyAmount: CurrencyAmount, tokenAmount: TokenAmount, pool: Pool)
- Provide liquidity to existing LP or create LP with the given nativeCurrency (like ETH, MATIC) - Token. Returns a populated transaction.
async removeLiquidity(percentToRemove: Percent, userPool: UserPoolContribution, slippage: number)
- Builds a transaction to remove a percentage of the user liquidity from the LP. Returns a populated transaction.
async removeLiquidityWithPermit( percentToRemove: Percent, userPool: UserPoolContribution, slippage: number, deadline: number, v: number, r: string, s: string)
- Same as removeLiquidity
but uses a permit
in stead of approve
on the ERC20. Only usable if the ERC20 supports permit
. Parameters "deadline, v, r s" are all part of the EIP-2612: permit
. Returns a populated transaction.
async removeNativeLiquidity(percentToRemove: Percent, userPool: UserPoolContribution, slippage: number)
- Same as removeLiquidity
but for nativeCurrency - Token. Returns a populated transaction.
async removeNativeLiquidityWithPermit(percentToRemove: Percent, userPool: UserPoolContribution, slippage: number, deadline: number, v: number, r: string, s: string)
- Same as removeNativeLiquidity
but uses a permit
in stead of approve
on the ERC20. Only usable if the ERC20 supports permit
. Parameters "deadline, v, r s" are all part of the EIP-2612: permit
. Returns a populated transaction.
async getAmountsOut(tokenAmount: TokenAmount, path: string[])
- Get a quote for a ExactIn swaps. Represents the amount of token that needs to be outputted in order to get the exact input. Parameter path
consists of special hashes that are build by the convertToDexPath
function.
Example:
import { convertToDexPath } from '@stichting-allianceblock-foundation/abdex-sdk-v2';
const path = convertToDexPath(
[tokenA, tokenB],
weight
);
// A quote for ExactIn swaps!
const amountsOutCall: BigNumber[] = await dex.getAmountsOut(
tokenAmountA,
path
);
async getAmountsIn(tokenAmount: TokenAmount, path: string[])
- Get a quote for a ExactOut swaps. Represents the amount of token that needs to be inputed in order to get the exact output. Parameter path
consists of special hashes that are build by the convertToDexPath
function.Example:
import { convertToDexPath } from '@stichting-allianceblock-foundation/abdex-sdk-v2';
const path = convertToDexPath(
[tokenA, tokenB],
weight
);
// A quote for ExactOut swaps!
const amountsInCall: BigNumber[] = await dex.getAmountsIn(
tokenAmountA,
path
);
DEX methods for swaping tokens:
async swapExactTokensForTokens
Takes in: tokenAmountIn: TokenAmount, // Exact amount of token to be swaped
tokenAmountOutMin: TokenAmount, // get the quote from getAmountsOut function
to: string,
path: string[], // get the path from convertToDexPath function
deadline: number
returns a populated transaction
Example:
const path = convertToDexPath(
[tokenAmountA.token, tokenHop, tokenB],
weight
);
const amountsOutCall: BigNumber[] = await dex.getAmountsOut(
tokenAmountA,
path
);
const amountsOut = amountsOutCall[amountsOutCall.length - 1];
tokenAmountB = new TokenAmount(tokenB, amountsOut.toBigInt());
const tx = await dex.swapExactTokensForTokens(
tokenAmountA,
tokenAmountB,
signerWithProvider.address,
path,
getDeadline()
);
const signedTransaction = await signerWithProvider.sendTransaction(tx);
async swapTokensForExactTokens
Takes in: tokenAmountOut: TokenAmount, // Exact amount of token to be swaped
tokenAmountInMax: TokenAmount, // get the quote from getAmountsIn function
to: string,
path: string[], // get the path from convertToDexPath function
deadline: number
returns a populated transaction
Example:
const path = convertToDexPath(
[tokenB, tokenHop, tokenAmountA.token],
weight
);
let amountsInCall: BigNumber[] = await dex.getAmountsIn(
tokenAmountA,
path
);
const amountsIn = amountsInCall[0];
tokenAmountB = new TokenAmount(tokenB, amountsIn.toBigInt());
const tx = await dex.swapTokensForExactTokens(
tokenAmountA,
tokenAmountB,
signerWithProvider.address,
path,
getDeadline()
);
const signedTransaction = await signerWithProvider.sendTransaction(tx);
async swapExactTokensForNative
Takes in: tokenAmountIn: TokenAmount, // Exact amount of token to be swaped
nativeAmountOutMin: TokenAmount, // get the quote from getAmountsOut function
to: string,
path: string[], // get the path from convertToDexPath function
deadline: number
returns a populated transaction
Example:
const path = convertToDexPath(
[tokenAmountB.token, tokenHop, native], // native is the wraped Coin
weight
);
const amountsOutCall: BigNumber[] = await dex.getAmountsOut(
tokenAmountB,
path
);
const amountsOut = amountsOutCall[amountsOutCall.length - 1];
const nativeOut = new TokenAmount(native, amountsOut.toBigInt());
const tx = await dex.swapExactTokensForNative(
tokenAmountB,
nativeOut,
signerWithProvider.address,
path,
getDeadline()
);
const signedTransaction = await signerWithProvider.sendTransaction(tx);
async swapTokensForExactNative
Takes in: nativeAmountOut: TokenAmount, // Exact amount of token to be swaped
tokenAmountInMax: TokenAmount, // get the quote from getAmountsIn function
to: string,
path: string[], // get the path from convertToDexPath function
deadline: number
returns a populated transaction
Example:
const path = convertToDexPath(
[tokenB, tokenHop, exactNativeOut.token], // native is the wraped Coin
weight
);
let amountsInCall: BigNumber[] = await dex.getAmountsIn(
exactNativeOut,
path
);
const amountsIn = amountsInCall[0];
tokenAmountB = new TokenAmount(tokenB, amountsIn.toBigInt());
const tx = await dex.swapTokensForExactNative(
exactNativeOut,
tokenAmountB,
signerWithProvider.address,
path,
getDeadline()
);
const signedTransaction = await signerWithProvider.sendTransaction(tx);
async swapNativeForExactTokens
Takes in: tokenAmountOut: TokenAmount, // Exact amount of token to be swaped
nativeAmountInMax: TokenAmount, // get the quote from getAmountsIn function
to: string,
path: string[], // get the path from convertToDexPath function
deadline: number
returns a populated transaction
Example:
const path = convertToDexPath(
[native, tokenHop, tokenAmountB.token], // native is the wraped Coin
weight
);
const amountsInCall: BigNumber[] = await dex.getAmountsIn(
tokenAmountB,
path
);
const amountsIn = amountsInCall[0];
const nativeIn = new TokenAmount(native, amountsIn.toBigInt());
const tx = await dex.swapNativeForExactTokens(
tokenAmountB,
nativeIn,
signerWithProvider.address,
path,
getDeadline()
);
const signedTransaction = await signerWithProvider.sendTransaction(tx);
async swapExactNativeForTokens
Takes in:nativeAmountIn: TokenAmount, // Exact amount of token to be swaped
tokenAmountOutMin: TokenAmount, // get the quote from getAmountsOut function
to: string,
path: string[], // get the path from convertToDexPath function
deadline: number
returns a populated transaction
Example:
const path = convertToDexPath(
[nativeExactIn.token, tokenHop, tokenB], // native is the wraped Coin
weight
);
const amountsOutCall: BigNumber[] = await dex.getAmountsOut(
nativeExactIn,
path
);
const amountsOut = amountsOutCall[amountsOutCall.length - 1];
tokenAmountB = new TokenAmount(tokenB, amountsOut.toBigInt());
const tx = await dex.swapExactNativeForTokens(
nativeExactIn,
tokenAmountB,
signerWithProvider.address,
path,
getDeadline()
);
const signedTransaction = await signerWithProvider.sendTransaction(tx);
DEX treasury methods:
async addLiquidityTreasuryRouter(tokenAmountA: TokenAmount,tokenAmountB: TokenAmount,amountATreasury: TokenAmount,amountBTreasury: TokenAmount,pool: Pool,signedAllowance: string,slippageTolerance: number = 500)
- provide liquidity to existing LP or create LP with the given tokens. Invests amountATreasury and amountBTreasury to the treasury. Returns a populated transaction.
async addNativeLiquidityTreasuryRouter(currencyAmount: CurrencyAmount, tokenAmount: TokenAmount, amountATreasury: TokenAmount, amountNativeTreasury: CurrencyAmount, pool: Pool, slippageTolerance: number = 500)
- Provide liquidity to existing LP or create LP with the given nativeCurrency (like ETH, MATIC) - Token. Invests amountATreasury and amountNativeTreasury to the treasury. Returns a populated transaction.
async removeLiquidityTreasuryRouter(percentToRemove: Percent, userPool: UserPoolContribution, amountATreasury: TokenAmount, amountBTreasury: TokenAmount, slippage: number)
- Builds a transaction to remove a percentage of the user liquidity from the LP. Withdraws amountATreasury and amountBTreasury to the treasury Returns a populated transaction.
async removeLiquidityWithPermitTreasuryRouter( percentToRemove: Percent, userPool: UserPoolContribution, amountATreasury: TokenAmount, amountBTreasury: TokenAmount, slippage: number, deadline: number, v: number, r: string, s: string)
- Same as removeLiquidityTreasuryRouter
but uses a permit
in stead of approve
on the ERC20. Only usable if the ERC20 supports permit
. Parameters "deadline, v, r s" are all part of the EIP-2612: permit
. Returns a populated transaction.
async removeNativeLiquidityTreasuryRouter(percentToRemove: Percent, userPool: UserPoolContribution, amountATreasury: TokenAmount, amountNativeTreasury: CurrencyAmount, slippage: number)
- Same as removeLiquidityTreasuryRouter
but for nativeCurrency - Token. Returns a populated transaction.
async removeNativeLiquidityWithPermitTreasuryRouter(percentToRemove: Percent, userPool: UserPoolContribution, amountATreasury: TokenAmount, amountNativeTreasury: CurrencyAmount, slippage: number, deadline: number, v: number, r: string, s: string)
- Same as removeNativeLiquidityTreasuryRouter
but uses a permit
in stead of approve
on the ERC20. Only usable if the ERC20 supports permit
. Parameters "deadline, v, r s" are all part of the EIP-2612: permit
. Returns a populated transaction.
async migrateTreasury(pool: Pool, amountADesired: TokenAmount, amountBDesired: TokenAmount, slippageTolerance: number = 500, deadline: Number)
- migrates tokens from a the treasury to the given pool. Returns a populated transaction.
async migrateTreasuryNative(pool: Pool, amountADesired: TokenAmount, amountBDesired: TokenAmount, slippageTolerance: number = 500, deadline: Number)
- migrates tokens from a the treasury to the given pool. Used when the pool has a native wrapped token. Returns a populated transaction.
async migratePool(percentToRemove: Percent, userPool: UserPoolContribution, signature: string, slippage: number, deadline: number)
- removes liquidity from the given pool and invests the tokens in the treasury. Returns a populated transaction. When migratePool()
and not migratePoolWithPermit()
is used it is verry important to approve the spending of the Pool Token like so:
const txAppr = await pool.contract.approve(
dex.treasuryRouterAddress, // dex is a DEX object
await pool.contract.balanceOf(signer.address) // pool is a Pool object
);
await txAppr.wait();
async migratePoolWithPermit(percentToRemove: Percent, userPool: UserPoolContribution, signature: string, slippage: number, deadline: number, v: number, r: string, s: string)
- removes liquidity from the given pool and invests the tokens in the treasury. Uses permit. Parameters "deadline, v, r s" are all part of the EIP-2612: permit
. Returns a populated transaction.
async migratePoolNative(percentToRemove: Percent, userPool: UserPoolContribution, signature: string, slippage: number, deadline: number)
- removes liquidity from the given pool and invests the tokens in the treasury. Used when the pool has a native wrapped token. When migratePoolNative()
and not migratePoolNativeWithPermit()
is used it is verry important to approve the spending of the Pool Token like so:
const txAppr = await pool.contract.approve(
dex.treasuryRouterAddress, // dex is a DEX object
await pool.contract.balanceOf(signer.address) // pool is a Pool object
);
await txAppr.wait();
async migratePoolNativeWithPermit(percentToRemove: Percent, userPool: UserPoolContribution, signature: string, slippage: number, deadline: number, v: number,r: string,s: string)
- removes liquidity from the given pool and invests the tokens in the treasury. Used when the pool has a native wrapped token. Uses permit. Parameters "deadline, v, r s" are all part of the EIP-2612: permit
. Returns a populated transaction.FAQs
This SDK is used to provide all needed functionality and comunication with the ABDEX Smart contrcts. > The main export from this SDK is the `async function initSDK`.
The npm package @allianceblock/abdex-sdk-v2 receives a total of 0 weekly downloads. As such, @allianceblock/abdex-sdk-v2 popularity was classified as not popular.
We found that @allianceblock/abdex-sdk-v2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.