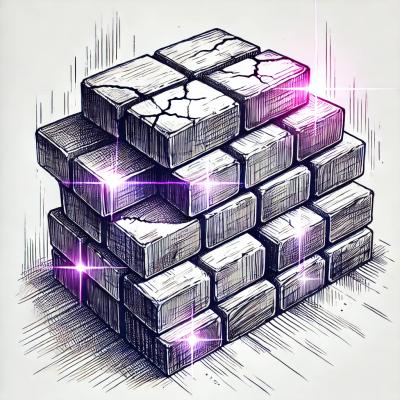
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@amplitude/analytics-browser
Advanced tools
@amplitude/analytics-browser is an npm package that allows you to integrate Amplitude's analytics capabilities into your web applications. It provides tools for tracking user events, managing user identities, and setting up custom properties to gain insights into user behavior.
Initialize Amplitude
This feature allows you to initialize the Amplitude analytics instance with your API key, which is necessary to start tracking events.
const amplitude = require('@amplitude/analytics-browser');
amplitude.init('YOUR_API_KEY');
Track Events
This feature allows you to track specific events within your application. In this example, a 'Button Clicked' event is tracked with an additional property 'buttonName'.
amplitude.track('Button Clicked', { buttonName: 'Sign Up' });
Identify Users
This feature allows you to identify users and set user properties. In this example, a user with ID 'user123' is identified and a custom property 'plan' is set to 'premium'.
amplitude.identify({ userId: 'user123', userProperties: { plan: 'premium' } });
Set User Properties
This feature allows you to set custom properties for a user. In this example, the user's age and gender are set.
amplitude.setUserProperties({ age: 30, gender: 'female' });
Log Revenue
This feature allows you to log revenue events. In this example, a revenue event is logged with an amount of 10.99 for product 'product_123' with a quantity of 1.
amplitude.logRevenue(10.99, 'product_123', 1);
Mixpanel is another popular analytics tool that provides similar functionalities to Amplitude. It allows you to track user interactions, manage user identities, and set custom properties. Mixpanel also offers advanced features like A/B testing and user segmentation.
Google Analytics is a widely-used web analytics service that tracks and reports website traffic. While it offers extensive tracking and reporting capabilities, it is more focused on web traffic analysis compared to the user-centric approach of Amplitude.
Official Amplitude SDK for Web
To get started with using Amplitude Browser SDK, install the package to your project via NPM or script loader.
This package is published on NPM registry and is available to be installed using npm and yarn.
# npm
npm install @amplitude/analytics-browser
# yarn
yarn add @amplitude/analytics-browser
Alternatively, the package is also distributed through a CDN. Copy and paste the script below to your html file.
<script type="text/javascript">
!function(){"use strict";!function(e,t){var r=e.amplitude||{_q:[]};if(r.invoked)e.console&&console.error&&console.error("Amplitude snippet has been loaded.");else{r.invoked=!0;var n=t.createElement("script");n.type="text/javascript",n.integrity="sha384-TeoEVjqxsQrwhskzwWGK6epJC7EkYjvl7k4gmwunKlRBkUK9nT01H4FL9z4VPMSm",n.crossOrigin="anonymous",n.async=!0,n.src="https://cdn.amplitude.com/libs/analytics-browser-0.3.1-min.js.gz",n.onload=function(){e.amplitude.runQueuedFunctions||console.log("[Amplitude] Error: could not load SDK")};var s=t.getElementsByTagName("script")[0];function v(e,t){e.prototype[t]=function(){return this._q.push({name:t,args:Array.prototype.slice.call(arguments,0)}),this}}s.parentNode.insertBefore(n,s);for(var o=function(){return this._q=[],this},i=["add","append","clearAll","prepend","set","setOnce","unset","preInsert","postInsert","remove","getUserProperties"],a=0;a<i.length;a++)v(o,i[a]);r.Identify=o;for(var u=function(){return this._q=[],this},c=["getEventProperties","setProductId","setQuantity","setPrice","setRevenue","setRevenueType","setEventProperties"],p=0;p<c.length;p++)v(u,c[p]);r.Revenue=u;var l=["getDeviceId","setDeviceId","getSessionId","setSessionId","getUserId","setUserId","setOptOut","setTransport"],d=["init","add","remove","track","logEvent","identify","groupIdentify","setGroup","revenue"];function m(e){function t(t,r){e[t]=function(){var n={promise:new Promise((r=>{e._q.push({name:t,args:Array.prototype.slice.call(arguments,0),resolve:r})}))};if(r)return n}}for(var r=0;r<l.length;r++)t(l[r],!1);for(var n=0;n<d.length;n++)t(d[n],!0)}m(r),e.amplitude=r}}(window,document)}();
amplitude.init("YOUR_API_KEY_HERE");
</script>
Initialization is necessary before any instrumentation is done. The API key for your Amplitude project is required.
amplitude.init(API_KEY)
Events represent how users interact with your application. For example, “Button Clicked” may be an action you want to note.
import { track } from '@amplitude/analytics-browser';
// Track a basic event
track('Button Clicked');
// Track events with additional properties
const eventProperties = {
selectedColors: ['red', 'blue'],
};
track('Button Clicked', eventProperties);
User properties help you understand your users at the time they performed some action within your app such as their device details, their preferences, or language.
import { Identify, identify } from '@amplitude/analytics-browser';
const event = new Identify();
// sets the value of a user property
event.set('key1', 'value1');
// sets the value of a user property only once
event.setOnce('key1', 'value1');
// increments a user property by some numerical value.
event.add('value1', 10);
// pre inserts a value or values to a user property
event.preInsert('ab-tests', 'new-user-test');
// post inserts a value or values to a user property
event.postInsert('ab-tests', 'new-user-test');
// removes a value or values to a user property
event.remove('ab-tests', 'new-user-test')
// sends identify event
identify(event);
import { setGroup } from '@amplitude/analytics-browser';
// set group with single group name
setGroup('orgId', '15');
// set group with multiple group names
setGroup('sport', ['soccer', 'tennis']);
This feature is only available to Growth and Enterprise customers who have purchased the Accounts add-on.
Use the Group Identify API to set or update properties of particular groups. However, these updates will only affect events going forward.
import { Identify, groupIdentify } from '@amplitude/analytics-browser';
const groupType = 'plan';
const groupName = 'enterprise';
const event = new Identify()
event.set('key1', 'value1');
groupIdentify(groupType, groupName, identify);
Revenue instances will store each revenue transaction and allow you to define several special revenue properties (such as 'revenueType', 'productIdentifier', etc.) that are used in Amplitude's Event Segmentation and Revenue LTV charts. These Revenue instance objects are then passed into revenue
to send as revenue events to Amplitude. This allows us to automatically display data relevant to revenue in the platform. You can use this to track both in-app and non-in-app purchases.
import { Revenue, revenue } from '@amplitude/analytics-browser';
const event = new Revenue()
.setProductId('com.company.productId')
.setPrice(3.99)
.setQuantity(3);
revenue(event);
All asynchronous API are optionally awaitable through a specific Promise interface. This also serves as callback interface.
// Using async/await
const results = await track('Button Clicked').promise;
result.event; // {...} (The final event object sent to Amplitude)
result.code; // 200 (The HTTP response status code of the request.
result.message; // "Event tracked successfully" (The response message)
// Using promises
track('Button Clicked').promise.then((result) => {
result.event; // {...} (The final event object sent to Amplitude)
result.code; // 200 (The HTTP response status code of the request.
result.message; // "Event tracked successfully" (The response message)
});
FAQs
Official Amplitude SDK for Web
The npm package @amplitude/analytics-browser receives a total of 324,052 weekly downloads. As such, @amplitude/analytics-browser popularity was classified as popular.
We found that @amplitude/analytics-browser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.