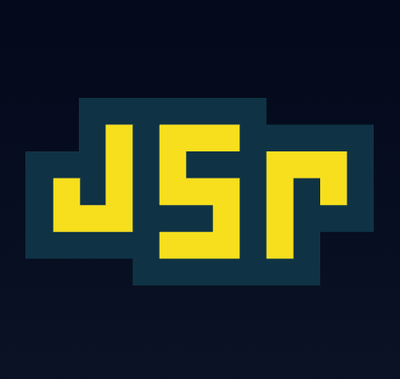
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@angular-devkit/build-optimizer
Advanced tools
@angular-devkit/build-optimizer is a tool designed to optimize Angular applications by performing various transformations and optimizations on the build output. It helps in reducing the size of the final bundle and improving the performance of the application.
Tree Shaking
Tree shaking is a feature that removes unused code from the final bundle. The code sample demonstrates how to use the buildOptimizer function to perform tree shaking on a given input code.
const { buildOptimizer } = require('@angular-devkit/build-optimizer');
const inputCode = 'import { Component } from "@angular/core";';
const result = buildOptimizer({ content: inputCode, inputFilePath: 'app.component.ts' });
console.log(result.content);
Inlining
Inlining is a feature that replaces external resources (like templates and styles) with inline content. The code sample shows how to use the buildOptimizer function to inline a template string in the input code.
const { buildOptimizer } = require('@angular-devkit/build-optimizer');
const inputCode = 'const template = `<div>{{title}}</div>`;';
const result = buildOptimizer({ content: inputCode, inputFilePath: 'app.component.ts' });
console.log(result.content);
Dead Code Elimination
Dead code elimination is a feature that removes code that is never used or executed. The code sample demonstrates how to use the buildOptimizer function to eliminate dead code from the input code.
const { buildOptimizer } = require('@angular-devkit/build-optimizer');
const inputCode = 'function unusedFunction() { return "I am not used"; }';
const result = buildOptimizer({ content: inputCode, inputFilePath: 'app.component.ts' });
console.log(result.content);
Webpack is a popular module bundler for JavaScript applications. It offers a wide range of optimization features, including tree shaking, code splitting, and minification. Compared to @angular-devkit/build-optimizer, Webpack provides a more comprehensive and flexible set of tools for optimizing and bundling applications.
Rollup is a module bundler that focuses on producing smaller and more efficient bundles. It supports tree shaking and other optimizations similar to @angular-devkit/build-optimizer. Rollup is often used for libraries and smaller projects, whereas @angular-devkit/build-optimizer is specifically tailored for Angular applications.
Terser is a JavaScript parser and mangler/compressor toolkit for ES6+. It is used to minify JavaScript code, reducing the size of the final bundle. While @angular-devkit/build-optimizer includes some minification capabilities, Terser is a dedicated tool for this purpose and can be used in conjunction with other bundlers like Webpack or Rollup.
Angular Build Optimizer contains Angular optimizations applicable to JavaScript code as a TypeScript transform pipeline.
This package is deprecated and should not be used. It has always been experimental (never hit
1.0.0
) and was an internal package for the Angular CLI. All the relevant functionality has been
moved into
@angular-devkit/build-angular
.
Transformations applied depend on file content:
Some of these optimizations add /*@__PURE__*/
comments.
These are used by JS optimization tools to identify pure functions that can potentially be dropped.
Static properties are folded into ES5 classes:
// input
var Clazz = (function () {
function Clazz() {}
return Clazz;
})();
Clazz.prop = 1;
// output
var Clazz = (function () {
function Clazz() {}
Clazz.prop = 1;
return Clazz;
})();
Angular decorators, property decorators and constructor parameters are removed, while leaving non-Angular ones intact.
// input
import { Injectable, Input, Component } from '@angular/core';
import { NotInjectable, NotComponent, NotInput } from 'another-lib';
var Clazz = (function () {
function Clazz() {}
return Clazz;
})();
Clazz.decorators = [{ type: Injectable }, { type: NotInjectable }];
Clazz.propDecorators = { 'ngIf': [{ type: Input }] };
Clazz.ctorParameters = function () {
return [{ type: Injector }];
};
var ComponentClazz = (function () {
function ComponentClazz() {}
__decorate([Input(), __metadata('design:type', Object)], Clazz.prototype, 'selected', void 0);
__decorate(
[NotInput(), __metadata('design:type', Object)],
Clazz.prototype,
'notSelected',
void 0,
);
ComponentClazz = __decorate(
[
NotComponent(),
Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
}),
],
ComponentClazz,
);
return ComponentClazz;
})();
// output
import { Injectable, Input, Component } from '@angular/core';
import { NotInjectable, NotComponent } from 'another-lib';
var Clazz = (function () {
function Clazz() {}
return Clazz;
})();
Clazz.decorators = [{ type: NotInjectable }];
var ComponentClazz = (function () {
function ComponentClazz() {}
__decorate(
[NotInput(), __metadata('design:type', Object)],
Clazz.prototype,
'notSelected',
void 0,
);
ComponentClazz = __decorate([NotComponent()], ComponentClazz);
return ComponentClazz;
})();
Adds /*@__PURE__*/
comments to top level downleveled class declarations and instantiation.
Warning: this transform assumes the file is a pure module. It should not be used with unpure modules.
// input
var Clazz = (function () {
function Clazz() {}
return Clazz;
})();
var newClazz = new Clazz();
var newClazzTwo = Clazz();
// output
var Clazz = /*@__PURE__*/ (function () {
function Clazz() {}
return Clazz;
})();
var newClazz = /*@__PURE__*/ new Clazz();
var newClazzTwo = /*@__PURE__*/ Clazz();
Adds /*@__PURE__*/
to downleveled TypeScript classes.
// input
var ReplayEvent = (function () {
function ReplayEvent(time, value) {
this.time = time;
this.value = value;
}
return ReplayEvent;
})();
// output
var ReplayEvent = /*@__PURE__*/ (function () {
function ReplayEvent(time, value) {
this.time = time;
this.value = value;
}
return ReplayEvent;
})();
TypeScript helpers (__extends/__decorate/__metadata/__param
) are replaced with tslib
imports whenever found.
// input
var __extends =
(this && this.__extends) ||
function (d, b) {
for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p];
function __() {
this.constructor = d;
}
d.prototype = b === null ? Object.create(b) : ((__.prototype = b.prototype), new __());
};
// output
import { __extends } from 'tslib';
Wrap downleveled TypeScript enums in a function, and adds /*@__PURE__*/
comment.
// input
var ChangeDetectionStrategy;
(function (ChangeDetectionStrategy) {
ChangeDetectionStrategy[(ChangeDetectionStrategy['OnPush'] = 0)] = 'OnPush';
ChangeDetectionStrategy[(ChangeDetectionStrategy['Default'] = 1)] = 'Default';
})(ChangeDetectionStrategy || (ChangeDetectionStrategy = {}));
// output
var ChangeDetectionStrategy = /*@__PURE__*/ (function () {
var ChangeDetectionStrategy = {};
ChangeDetectionStrategy[(ChangeDetectionStrategy['OnPush'] = 0)] = 'OnPush';
ChangeDetectionStrategy[(ChangeDetectionStrategy['Default'] = 1)] = 'Default';
return ChangeDetectionStrategy;
})();
import { buildOptimizer } from '@angular-devkit/build-optimizer';
const transpiledContent = buildOptimizer({ content: input }).content;
Available options:
export interface BuildOptimizerOptions {
content?: string;
inputFilePath?: string;
outputFilePath?: string;
emitSourceMap?: boolean;
strict?: boolean;
isSideEffectFree?: boolean;
}
import { BuildOptimizerWebpackPlugin } from '@angular-devkit/build-optimizer';
module.exports = {
plugins: [
new BuildOptimizerWebpackPlugin(),
]
module: {
rules: [
{
test: /\.js$/,
loader: '@angular-devkit/build-optimizer/webpack-loader',
options: {
sourceMap: false
}
}
]
}
}
build-optimizer input.js
build-optimizer input.js output.js
FAQs
Angular Build Optimizer
We found that @angular-devkit/build-optimizer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.