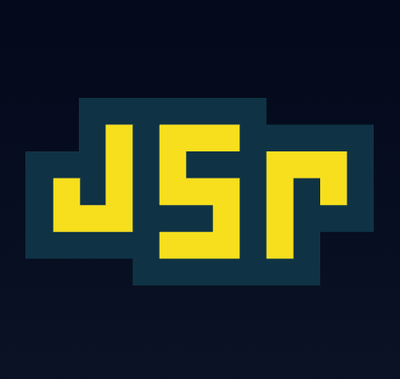
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@angular/compiler
Advanced tools
The @angular/compiler package is a core part of the Angular framework that is responsible for compiling Angular templates into JavaScript code that can be executed by the browser. It takes the templates and annotations provided in Angular components and generates code that represents the structure and behavior of the application.
Template Compilation
This feature allows you to compile Angular components and modules dynamically at runtime. The code sample demonstrates how to use the JIT compiler to compile a module and its components asynchronously.
import { Compiler, COMPILER_OPTIONS, CompilerFactory } from '@angular/core';
import { JitCompilerFactory } from '@angular/platform-browser-dynamic';
// Get a reference to the JIT compiler factory
const compilerFactory: CompilerFactory = platformBrowserDynamic().injector.get(CompilerFactory);
const compiler = compilerFactory.createCompiler();
// Compile a component dynamically
compiler.compileModuleAndAllComponentsAsync(DynamicModule)
.then(compiled => {
const moduleRef = compiled.ngModuleFactory.create(this.injector);
const componentFactory = compiled.componentFactories[0];
this.container.createComponent(componentFactory);
});
AOT Compilation
Ahead-of-Time (AOT) compilation converts Angular HTML and TypeScript code into efficient JavaScript code during the build process, before the browser downloads and runs the code. This code sample shows how to bootstrap an Angular application using the AOT-compiled module factory.
import { enableProdMode } from '@angular/core';
import { platformBrowser } from '@angular/platform-browser';
import { AppModuleNgFactory } from './app/app.module.ngfactory';
enableProdMode();
platformBrowser().bootstrapModuleFactory(AppModuleNgFactory);
Babel is a JavaScript compiler that converts ECMAScript 2015+ code into a backwards-compatible version of JavaScript that can be run by older JavaScript engines. Babel is similar to @angular/compiler in that it performs code transformation, but it is not specific to Angular and does not compile Angular templates.
TypeScript is a superset of JavaScript that compiles to plain JavaScript. It is similar to @angular/compiler in that it performs a compilation step, but TypeScript focuses on type-checking and converting TypeScript code to JavaScript, rather than compiling framework-specific templates.
The vue-template-compiler package is used to compile Vue.js templates into render functions. It is similar to @angular/compiler as both are used for compiling framework-specific templates, but they target different frameworks (Vue.js vs Angular).
The sources for this package are in the main Angular repo. Please file issues and pull requests against that repo.
License: MIT
14.1.0 (2022-07-20)
createNgModuleRef
is deprecated in favor of newly added createNgModule
one.inject()
has been deprecated, in favor of the
new options object. Correspondingly, InjectFlags
is deprecated as well.| Commit | Type | Description |
| -- | -- | -- |
| 55308f2df5 | feat | add provideAnimations()
and provideNoopAnimations()
functions (#46793) |
| Commit | Type | Description |
| -- | -- | -- |
| 4a2e7335b1 | feat | make the CommonModule
pipes standalone (#46401) |
| a7597dd080 | feat | make the CommonModule directives standalone (#46469) |
| Commit | Type | Description | | -- | -- | -- | | 33ce3883a5 | feat | Add extended diagnostic to warn when missing let on ngForOf (#46683) | | 6f11a58040 | feat | Add extended diagnostic to warn when text attributes are intended to be bindings (#46161) | | 9e836c232f | feat | warn when style suffixes are used with attribute bindings (#46651) |
| Commit | Type | Description | | -- | -- | -- | | 93c65e7b14 | feat | add extended diagnostic for non-nullable optional chains (#46686) | | 131d029da1 | feat | detect missing control flow directive imports in standalone components (#46146) | | 6b8e60c06a | fix | improve the missingControlFlowDirective message (#46846) |
| Commit | Type | Description |
| -- | -- | -- |
| e8e8e5f171 | feat | add createComponent
function |
| b5153814af | feat | add reflectComponentType
function |
| 96c6139c9a | feat | add ability to set inputs on ComponentRef (#46641) |
| a6d5fe202c | feat | alias createNgModuleRef
as createNgModule
(#46789) |
| 71e606d3c3 | feat | expose EnvironmentInjector on ApplicationRef (#46665) |
| 19e6d9ccd3 | feat | import AsyncStackTaggingZone if available (#46693) |
| a7a14df5f8 | feat | introduce EnvironmentInjector.runInContext
API (#46653) |
| fa52b6e906 | feat | options object to supersede bit flags for inject()
(#46649) |
| af20112222 | feat | support the descendants option for ContentChild queries (#46638) |
| 945a3ad359 | fix | Fix runInContext
for NgModuleRef
injector (#46877) |
| bb7c80477b | fix | make parent injector argument required in createEnvironmentInjector
(#46397) |
| Commit | Type | Description | | -- | -- | -- | | 82acbf919b | feat | improve error message for nullish header (#46059) |
| Commit | Type | Description |
| -- | -- | -- |
| 53ca936366 | feat | Add ability to create UrlTree
from any ActivatedRouteSnapshot
(#45877) |
| de058bba99 | feat | Add CanMatch guard to control whether a Route should match (#46021) |
| 6c1357dd7d | feat | Add stable cancelation code to NavigationCancel
event (#46675) |
| a4ce273e50 | feat | Add the target RouterStateSnapshot
to NavigationError
(#46731) |
| abe3759e24 | fix | allow to return UrlTree
from CanMatchFn
(#46455) |
| e8c7dd10e9 | fix | Ensure APP_INITIALIZER
of enabledBlocking
option completes (#46026) |
| ce20ed067f | fix | Ensure Route injector is created before running CanMatch guards (#46394) |
| 6a7b818d94 | fix | Ensure target RouterStateSnapshot
is defined in NavigationError
(#46842) |
| f94c6f433d | fix | Expose CanMatchFn as public API (#46394) |
| e8ae0fe3e9 | fix | Fix cancelation code for canLoad rejections (#46752) |
| Commit | Type | Description |
| -- | -- | -- |
| e9cb0454dc | feat | more closely align UpgradeModule#bootstrap()
with angular.bootstrap()
(#46214) |
AleksanderBodurri, Alex Rickabaugh, Andrew Kushnir, Andrew Scott, Cédric Exbrayat, Dmitrij Kuba, Dylan Hunn, George Kalpakas, Jessica Janiuk, JiaLiPassion, Joey Perrott, John Vandenberg, JoostK, Keith Li, Or'el Ben-Ya'ir, Paul Gschwendtner, Pawel Kozlowski, SyedAhm3r, arturovt, mariu, markostanimirovic and mgechev
<!-- CHANGELOG SPLIT MARKER --><a name="14.0.7"></a>
FAQs
Angular - the compiler library
The npm package @angular/compiler receives a total of 1,841,578 weekly downloads. As such, @angular/compiler popularity was classified as popular.
We found that @angular/compiler demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.