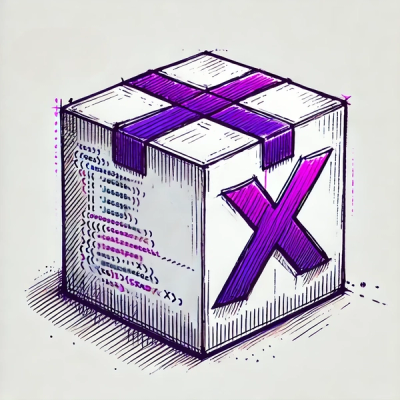
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@antoniovdlc/range
Advanced tools
Implement ranges in JavaScript.
This package is distributed via npm:
npm install @antoniovdlc/range
Ranges are natively supported by a few (popular) programming languages. They allow for iteration over a defined space, while not having a linear increase in their memory footprint (all ranges always store a similar amount of data).
You can use this library either as an ES module or a CommonJS package:
import range from "@antoniovdlc/range";
- or -
const range = require("@antoniovdlc/range");
To create a range:
const start = 0;
const stop = 10;
const step = 2; // Defaults to `1` if not passed
const inclusive = true; // Defaults to `false` if not passed
const r = range(start, stop, step, inclusive);
You can also pass an options object for convenience:
const start = 0;
const stop = 10;
const opts = {
step: 2, // Defaults to `1` if not passed
inclusive: true, // Defaults to `false` if not passed
};
const r = range(start, stop, opts);
You can use the following getters on ranges:
Returns the start value of a range:
range(0, 10).start; // 0
Returns the stop value of a range:
range(0, 10).stop; // 10
Returns the step value of a range:
range(0, 10).step; // 1 (by default)
Returns true
if the range is inclusive of its last value:
range(0, 10).isInclusive; // false (by default)
Returns the first value of a range:
range(0, 10).first; // 0
Returns the last value of a range:
range(0, 10).last; // 9
range(0, 10, 1, true).last; // 10
You can use the following methods on ranges:
Returns a string representation of a range:
range(0, 10).toString(); // 0..10
range(0, 10, 2).toString(); // 0..10{2}
range(0, 10, 2, true).toString(); // 0..=10{2}
Returns an array representation of a range:
range(0, 10).toArray(); // [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
range(0, 10, 2).toArray(); // [0, 2, 4, 6, 8]
range(0, 10, 1, true).toArray(); // [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
You can also use the follow syntax as
ranges
are iterable:[...range(0, 10)]
Checks if 2 ranges are equal (same start, stop, and step):
range(0, 10).equal(range(0, 10)); // true
range(0, 10, 2).equal(range(0, 10)); // false
range(0, 10, 2, true).equal(range(0, 10, 2, false)); // false
Checks if one range includes another (irrespective of step values):
range(0, 10).includes(range(1, 5)); // true
range(0, 10).includes(range(1, 11)); // false
Adds ranges together (only if step values are equal):
range(0, 5).add(range(1, 10)); // range(0, 10)
Iterate over a range and apply fn
function to every element of the range:
range(0, 10).forEach((i) => console.log(i));
// 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
range(0, 10, 1, true).forEach((i) => console.log(i));
// 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
range(0, 10, 2).forEach((i) => console.log(i));
// 0, 2, 4, 6, 8
Iterate over a range and apply fn
function to every element of the range asynchronously:
await range(0, 10).forEachAsync((i) => console.log(i));
// 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
await range(0, 10, 1, true).forEachAsync((i) => console.log(i));
// 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
await range(0, 10, 2).forEachAsync((i) => console.log(i));
// 0, 2, 4, 6, 8
Map over a range and apply fn
function to every element of the range:
const result = range(0, 5).map((i) => i ** 2);
// [0, 1, 4, 9, 16]
const result = range(0, 5, 1, true).map((i) => i ** 2);
// [0, 1, 4, 9, 16, 25]
const result = range(0, 10, 2).map((i) => i ** 2);
// [0, 4, 16, 36, 64]
Map over a range and apply fn
function to every element of the range asynchronously:
const result = await range(0, 5).mapAsync(
(i) => new Promise((resolve) => resolve(i ** 2))
);
// [0, 1, 4, 9, 16]
const result = await range(0, 5, 1, true).mapAsync(
(i) => new Promise((resolve) => resolve(i ** 2))
);
// [0, 1, 4, 9, 16, 25]
const result = await range(0, 10, 2).mapAsync(
(i) => new Promise((resolve) => resolve(i ** 2))
);
// [0, 4, 16, 36, 64]
Ranges implement both the iterable and the iterator protocols. This allows iteration over ranges as follow:
for (let i of range(0, 10)) {
console.log(i);
}
// 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
for (let i of range(0, 10, 1, true)) {
console.log(i);
}
// 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
for (let i of range(0, 10, 2)) {
console.log(i);
}
// 0, 2, 4, 6, 8
const r = range(0, 10);
console.log(r.next()); // { value: 0, done: false }
console.log(r.next()); // { value: 1, done: false }
console.log(r.next()); // { value: 2, done: false }
...
console.log(r.next()); // { value: 8, done: false }
console.log(r.next()); // { value: 9, done: false }
console.log(r.next()); // { value: undefined, done: true }
Note that ranges are not consumed after a single iteration. They can be reused in consecutive iterations!
MIT
FAQs
Implement ranges in JavaScript
We found that @antoniovdlc/range demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.