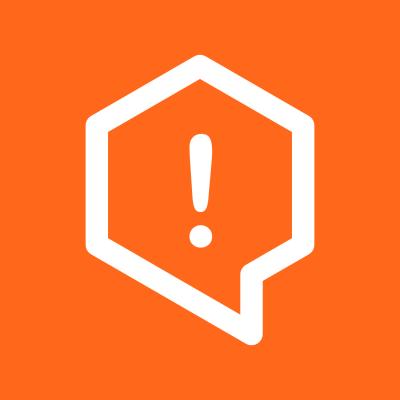
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@aofl/form-validate
Advanced tools
@aofl/aofl-validate comes with a handful of form validation functions and an aofl-element mixin to simplify form validation for webcomponents. It even bundles some basic validators.
npm i -S @aofl/aofl-validate
...
import {validationMixin, isRequired, minLength} from '@aofl/aofl-validate';
class Login extends validationMixin(AoflElement) {
get validators() {
return {
username: {
isRequired
},
password: {
isRequired,
min: minLength(8)
}
};
}
}
// results in
login-form: {
form: {
valid: true,
pending: false,
observed: false,
username: {
valid: true,
pending: false,
observed: false,
isRequired: {
valid: true,
pending: false,
observed: false,
}
},
password: {
valid: true,
pending: false,
observed: false,
isRequired: {
valid: true,
pending: false,
observed: false,
},
min: {
valid: true,
pending: false,
observed: false,
}
}
}
}
All properties of element.form
are instances of the same interface and therefore all properties and methods are available on each level.
this.form.validate();
this.form.username.validate();
this.form.username.inRequired.validate();
// and respectively
this.form.valid;
this.form.username.valid;
this.form.username.isRequired.valid;
reset()
Reset the form validation object to it's initial state.
validate()
When validate is invoked it call validate on it's properties resulting in the preperties' validation functions to be invoked.
this.form.validate(); // validates every property
this.form.username.validate(); // validates username
valid
Checks the .valid
property of it's properties and return false if any of them are invalid.
this.form.valid; // return the validity of the entire form
this.form.username.valid; // return the validity of username
pending
Checks the .pending
property of it's properties and return true if any of them are pending.
this.form.pending; // return true if any property is pending
this.form.username.pending; // returns true if username is pending
observed
Boolean property that signifies if a form proprety was validated.
this.form.observed; // return true if all properties have been observed
this.form.username.pending; // returns true if username has been observed
validateComplete
Returns a promise that resolves when the latest async operation has completed.
Test whether value is empty or not.
import {isRequired} from '@aofl/aofl-validate';
class MyElement extends validationMixin(AoflElement) {
...
get validators() {
return {
password: {
isRequired
},
...
};
}
...
}
Test whether the values of two fields are equal. E.g. password & verify password fields.
import {isEqual} from '@aofl/aofl-validate';
class MyElement extends validationMixin(AoflElement) {
...
get validators() {
return {
password: {
...
},
verifyPassword: {
isEqual: isEqual('password')
}
};
}
...
}
Test whether the value meets a minimum length requirement.
import {minLength} from '@aofl/aofl-validate';
class MyElement extends validationMixin(AoflElement) {
...
get validators() {
return {
password: {
minLength: minLength(8)
},
...
};
}
...
}
Test whether the value meets a maximum length requirement.
import {maxLength} from '@aofl/aofl-validate';
class MyElement extends validationMixin(AoflElement) {
...
get validators() {
return {
password: {
maxLength: maxLength(8)
},
...
};
}
...
}
Test whether the value consists of only digits.
import {isAllDigits} from '@aofl/aofl-validate';
class MyElement extends validationMixin(AoflElement) {
...
get validators() {
return {
password: {
isAllDigits
},
...
};
}
...
}
Test whether the value matches a pattern.
import {pattern} from '@aofl/aofl-validate';
class MyElement extends validationMixin(AoflElement) {
...
get validators() {
return {
email: {
validEmail: pattern(/[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2, 4}$/)
},
...
};
}
...
}
Compare values of 2 form fields based on a comparator function
import {compare, isRequired} from '@aofl/aofl-validate';
class MyElement extends validationMixin(AoflElement) {
...
get validators() {
return {
questions: {
question1: {
isRequired
},
question2: {
isRequired,
unique: compare('questions.question1', (value, otherValue) => value !== otherValue)
},
question3: {
isRequired,
unique: compare('questions.question2', (value, otherValue) => value !== otherValue)
}
},
...
};
}
...
}
FAQs
Form validation mixin for AoflElement
The npm package @aofl/form-validate receives a total of 389 weekly downloads. As such, @aofl/form-validate popularity was classified as not popular.
We found that @aofl/form-validate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.