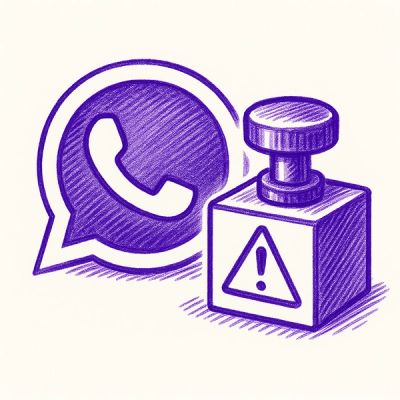
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
@apihero/js
Advanced tools
npm install @apihero/js
Automatically proxy all 3rd-party requests through API Hero in Node.js
import { setupProxy } from "@apihero/js/node";
const proxy = setupProxy({
projectKey: "hero_123abc",
url: "https://proxy.apihero.run",
});
// Start intercepting requests and sending them to https://proxy.apihero.run
proxy.start();
// You can always stop intercepting requests
proxy.stop();
You can also setup matchers for only capturing some traffic:
import { setupProxy } from "@apihero/js/node";
const proxy = setupProxy({
allow: [
"https://api.github.com/*",
"https://api.twitter.com/users/*",
{ url: "https://httpbin.org/*", method: "GET" },
],
projectKey: "hero_123abc",
url: "https://proxy.apihero.run",
});
// Start intercepting requests and sending them to https://proxy.apihero.run
proxy.start();
Capture all traffic except the matchers in the deny option:
import { setupProxy } from "@apihero/js/node";
const proxy = setupProxy({
deny: ["https://myprivateapi.dev/*"]
projectKey: "hero_123abc",
url: "https://proxy.apihero.run",
});
// Start intercepting requests and sending them to https://proxy.apihero.run
proxy.start();
If you would like to use API Hero in a Cloudflare Worker environment, simply change the import above from @apihero/js/node
to @apihero/js
, like so:
import { setupProxy } from "@apihero/js";
// This will now intercept requests made to `globalThis.fetch` and proxy them through https://proxy.apihero.run
const proxy = setupProxy({
projectKey: "hero_123abc",
url: "https://proxy.apihero.run",
});
// Start intercepting requests and sending them to https://proxy.apihero.run
proxy.start();
// You can always stop intercepting requests
proxy.stop();
This library uses a Service Worker approach to capture and proxy API requests performed in the browser, so the setup is slightly different from Node.
You'll first need to add the Service Worker code to your public directly, using the @apihero/js
cli:
npm exec apihero-js init public/ --save
Next, create a file to setup the worker, for example in src/apihero.js
:
// src/apihero.js
import { setupWorker } from "@apihero/js";
export const worker = setupWorker({
// You MUST supply the allow option for setupWorker, to ensure too many requests are not sent to the API Hero proxy
allow: ["api.github.com", "api.twitter.com/users/*"],
deny: ["myprivateapi.dev/*"],
projectKey: "hero_123abc",
url: "https://proxy.apihero.run",
});
Finally, will need to start the worker in your application code. For example, in a React app
// src/index.js
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
import { worker } from "./apihero";
worker.start();
ReactDOM.render(<App />, document.getElementById("root"));
You could also restrict proxying to only local dev like so:
// src/index.js
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
if (process.env.NODE_ENV === "development") {
const { worker } = require("./apihero");
worker.start();
}
ReactDOM.render(<App />, document.getElementById("root"));
FAQs
## Installation
The npm package @apihero/js receives a total of 0 weekly downloads. As such, @apihero/js popularity was classified as not popular.
We found that @apihero/js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.