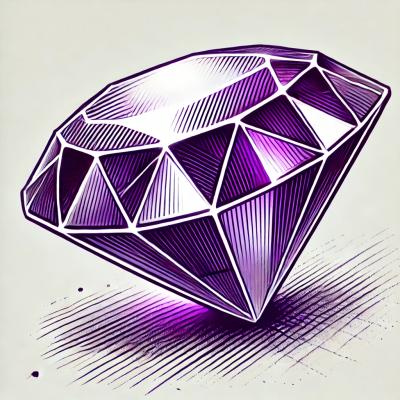
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@aplus-frontend/oss
Advanced tools
Rebuild ali-oss javascript sdk that provide private sts server methods
Repackaged ali-oss sdk to make it easier to upload files to Alibaba Cloud oss
//add -w in you root folder
pnpm install @aplus-frontend/oss -S -w
//register client instance
import { client } from '@aplus-frontend/oss'
//some code in you project
import { you_STS_server } from '@/api/sts'
//init oss access token
const ossIntsance = await client.initOssClient({
you_STS_server,
onFailure: err => {
console.warn('get oss accessSecret fail', err)
}
})
//after init
//you can use ossIntsance do something
ossIntsance.put(..)
ossIntsance.getSignatureUrl(..)
ossIntsance.downloadFile(..)
ossIntsance.deleteFile(..)
ossIntsance.pauseUpload(...)
// upload image or video with oss
// your can use put method to upload
const result = await client.put({
fileName: file.name,
dirName: 'video',
data: file,
progressCallBack: (p: number) => {
percentVideo.value = p
},
//the number of parts to be uploaded in parallel
parallel: 6,
//the suggested size for each part, default 1024 * 1024(1MB), minimum 100 * 1024(100KB)
partSize: 1024 * 1024
})
if (result.status === 200) {
const { status, previewUrl, saveUrl } = result
console.log('status---', status)
console.log('previewUrl---', previewUrl)
console.log('saveUrl---', saveUrl)
} else {
//failure
console.log(result.message)
}
//you can use download method to download
let res = client.downloadFile(fileName)
console.log(res.message)
console.log(res.status)
//you can get signature url to preview with getSignatureUrl method
let signatureUrl = client.getSignatureUrl(pathName)
//get signature url
//after expires seconds, the url will become invalid, default is 3600s
console.log(signatureUrl)
//delete file
let res = await client.deleteFile(pathName)
console.log(res)
//pauseUpload
let res = = await client.pauseUpload()
console.log(res)
type: initOssClient(options: RequestOssOptions): Promise<Oss | null>
tips: register client instance with you sts server
ResquestOssOptions
props | type | default | directions |
---|---|---|---|
getOssAccess | () => Promise<accessCreate> | - | init oss access token |
onFailure | (err: any) => void | - | get oss accessSerct fail |
locale | zh_CN | en_US | zh_CN | language |
bucket | string | - | (not required)choose bucket where to upload in ali-yun oss |
type: put({ fileName, dirName, data, progressCallBack, parallel, partSize }: uploadParams): Promise<actionResponse>;
tips: you can put image video with put core method
put options
Props | Type | default | explanation |
---|---|---|---|
fileName | string | - | file Name |
dirName | string | - | upload folder name |
data | File | - | upload data |
progressCallBack | (p:number) => void | - | upload progress |
parallel | number | 6 | the number of parts to be uploaded in parallel |
partSize | number | 1024*1024(1M) | the suggested size for each part, default 1024 _ 1024(1MB), minimum 100 _ 1024(100KB) |
expires | number | 1800 | after expires seconds, the url will become invalid, default is 1800 |
needHash | boolean | true | hash upload file name |
type: extractZip(file: File): Promise<extractedFile[]>;
tips: The zip file will be decompressed with extractZip
methods ,After that you want to upload to Ali oss one by one through the put
method by yourself.
extractZip options
Props | Type | default | explanation |
---|---|---|---|
file | File | - | file data |
//upload zip file demo
//template
<input type="file" @change="onFileChange" />
//logical
const onFileChange = async (e) => {
let res = await client.extractZip(e.target.files[0]);
console.log(res)
};
type: downloadFile(savePathName: Array<string> | string | Array<{path: string;fileName: string;}>): Promise<actionResponse>
tips: download file with downloadFile method (support multiple download files)
downloadFile options
Props | Type | default | explanation |
---|---|---|---|
savePathName | Array<string> | string | Array<{path: string;fileName: string;}> | - | if multipart download will be array,single file download will be string |
type: getSignatureUrl(name: string, expires?: number, rename?: string): Promise<string | undefined>;
tips: get signature url to preview
getSignatureUrl options
Props | Type | default | explanation |
---|---|---|---|
name | string | - | file path name |
expires | number | 3600(s) | expires time |
rename | string | - | customized file name |
type: deleteFile(savePathName: string, isRealDelete?: boolean): Promise<actionResponse>;
tips: delete oss file
deleteFile options
Props | Type | default | explanation |
---|---|---|---|
savePathName | string | - | file path name |
isRealDelete | boolean | false | In order to avoid accidentally deleting oss files, developers must manually turn on isRealDelete=true when deleting files. The default is false. |
type: pauseUpload(): Promise<actionResponse>;
tips: pause upload
type: destroy(): Promise<actionResponse>
tips: destroy oss instance
Props | Type | default | explanation |
---|---|---|---|
status | number | - | status code(success 200 ,other will be fail) |
previewUrl | string | - | preview file url,you can preview image or video after uploaded |
saveUrl | string | - | your backend server will be save this path |
message | string | - | success message or fail message |
originalFileName | string | - | upload file original name |
You can write useOss.ts
global Hooks like below
import { client } from '@aplus-frontend/oss';
import type { Oss } from '@aplus-frontend/oss';
import { getOssAccess } from '@/api/sys/uploadOss';
import { onMounted } from 'vue';
import { useMessage } from '@/hooks/web/useMessage';
const { createMessage } = useMessage();
let ossInstance: Oss;
// 获取client实例对象
export function useOss() {
return {
client: ossInstance
};
}
// 初始化client实例对象
export function useOssInit() {
onMounted(async () => {
ossInstance = await client.initOssClient({
getOssAccess,
onFailure: (err) => {
console.log(err)
}
});
});
return useOss();
}
you need to download or upload functionality in your application you can use like this
import { useOssInit, useOss } from '@/hooks/web/useOss';
//oss not init
//your can use useOssInit to initial
useOssInit();
//after initial you can use useOss to get client instance
const { client } = useOss();
const result = await client.put(...)
support multipart upload with exports createOssInstance
method to create multipart upload instance
import { createOssInstance } from '@aplus-frontend/oss';
const client1 = createOssInstance()
client1.put(...)
const client2 = createOssInstance()
client2.put(...)
//stop client1 upload
client1.pauseUpload()
//stop client2 upload
client2.pauseUpload()
FAQs
Rebuild ali-oss javascript sdk that provide private sts server methods
We found that @aplus-frontend/oss demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.