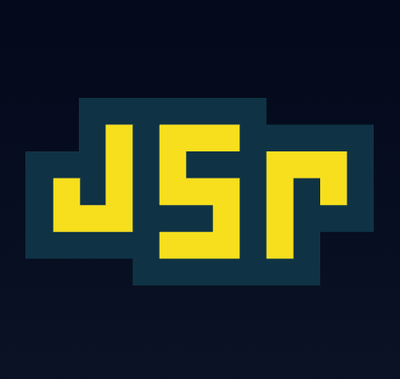
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@arg-def/mapper-js
Advanced tools
Fast, reliable and intuitive object mapping.
npm install @arg-def/mapper-js --save
#or
yarn add @arg-def/mapper-js
Before we start, it is essential that we know your data structure so we can map it accordingly.
For this demo case, let's assume that we have the following object:
const source = {
person: {
name: {
firstName: 'John',
lastName: 'Doe'
},
age: 32,
drinks: ['beer', 'whiskey'],
address: [
{
street: 'Infinite Loop',
city: 'Cupertino',
state: 'CA',
postalCode: 95014,
country: 'United States'
},
{
street: '1600 Amphitheatre',
city: 'Mountain View',
state: 'CA',
postalCode: 94043,
country: 'United States',
},
]
}
}
At this step, we need to create our mapping
against our data source
.
We will be using dot notation
to create our final structure
.
For more info about
dot notation
API, check out the documentation
With mapper
, it is possible to get
one or several values from our source
and even transform
it in the way we need.
For that, map.get()
accepts single dot notation
path or
an array of dot notation paths
. E.g.: map.get('person.name.firstName')
, map.get([person.name.firstName, person.name.lastName]);
'
Those values can be transformed by using the .transform()
method, which expects a function
as argument and provides
the selected values as array in the parameter
.
For more information about the usage, check the API Documentation.
Now let's create our mapping
!
import mapper from '@arg-def/mapper-js'
...
const mapping = mapper.mapping((map) => ({
'person.name': map
.get('person.name')
.transform(([{ firstName, lastName }]) => `${firstName} ${lastName}`)
.value,
'person.lastName': map.get('person.lastName').value,
'person.isAllowedToDrive': map.get(['person.age', 'person.drinks'])
.transform(([age, drinks]) => age > 18 && drinks.includes('soft-drink'))
.value,
address: map.get('person.address').value,
defaultAddress: map.get('person.address[0]').value,
}));
import mapper from '@arg-def/mapper-js'
...
const result = mapper(source, mapping);
/* outputs
{
person: {
name: 'John Doe',
isAllowedToDrive: false,
},
address: [
{
street: 'Infinite Loop',
city: 'Cupertino',
state: 'CA',
postalCode: 95014,
country: 'United States'
},
...
],
defaultAddress: {
street: 'Infinite Loop',
city: 'Cupertino',
state: 'CA',
postalCode: 95014,
country: 'United States'
}
}
*/
Type: function()
Parameter: source, mapping
Signature: (callback: (map: IMap) => IMapping): Function => callback
Description:
mapper()
mappes your source data against your mapping.
Example:
mapper(source, mapping);
/* outputs
{
employee: {
name: 'John',
age: 32,
address: [
{
street: 'Infinite Loop',
city: 'Cupertino',
state: 'CA',
postalCode: 95014,
country: 'United States'
},
...
],
},
}
*/
Type: function()
Parameter: map
Signature: (callback: (map: IMap) => IMapping): Function => callback
Description:
mapper.mapping()
is the method responsible for mapping the values from your source data against your object shape.
It accepts dot notation
path as key
.
Example:
// raw definition
const mapping = mapper.mapping((map) => {
...
});
// with map() query
const mapping = mapper.mapping((map) => {
'employee.name': map.get('person.name.firstName').value,
'employee.age': map.get('person.name.age').value,
'employee.address': map.get('person.address').value,
});
Type: object
get
Type: function
Parameter: string|string[]
Signature: <T>(key: string | string[]) => IMap;
Description:
.get
method retrieves values from your source data using dot notation
path, it accepts a string or array of string.
Example:
map.get('person.name.firstName');
map.get(['person.name.firstName', 'person.name.lastName']);
transform
Type: function
Parameter: any[]
Signature: <T, V>(callback: (values: T[]) => V) => IMap
Description:
.transform
method provides you the ability to transform the retrieved values from .get()
according to your needs, and for that, it expects a return value.
.transform
provides you as parameter, an array
with the retrieved values in the same order as defined in the get
method.
Example:
// single value
map.get('person.name.firstName')
.transform(([firstName]) => firstName.toLoweCase());
// multiple values
map.get(['person.name.firstName', 'person.name.lastName'])
.transform(([firstName, lastName]) => `${firstName} ${lastName]`);
value
Type: readonly
Returns: any | any[] | undefined | null
Description:
.value
returns the value of your dot notation
query. If transformed, returns the transformed value.
Example:
// single value
map.get('person.name.firstName')
.transform(([firstName]) => firstName.toLoweCase())
.value;
// multiple values
map.get(['person.name.firstName', 'person.name.lastName'])
.transform(([firstName, lastName]) => `${firstName} ${lastName]`)
.value;
FAQs
Fast, reliable and intuitive object mapping.
The npm package @arg-def/mapper-js receives a total of 4 weekly downloads. As such, @arg-def/mapper-js popularity was classified as not popular.
We found that @arg-def/mapper-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.