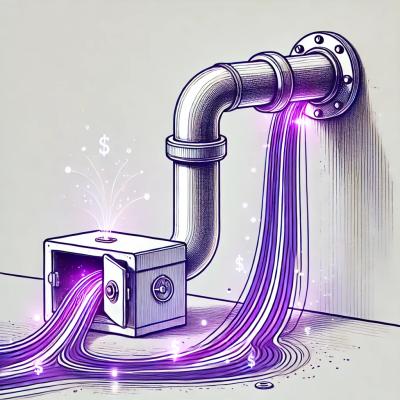
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@astro-utils/forms
Advanced tools
Server component for Astro (call server functions from client side with validation and state management)
Server component for Astro (validation and state management)
This package is a framework for Astro.js that allows you to create forms and manage their state without any JavaScript.
It also allows you to validate the form on the client side and server side, and protect against CSRF attacks.
zod
---
import { Bind, BindForm, BButton, BInput } from "@astro-utils/forms/forms.js";
import Layout from "../layouts/Layout.astro";
const form = Bind();
let showSubmitText: string;
function formSubmit(){
showSubmitText = `You name is ${form.name}, you are ${form.age} years old. `;
}
---
<Layout>
<BindForm bind={form}>
{showSubmitText}
<h4>What you name*</h4>
<BInput type="text" name="name" maxlength={20} required/>
<h4>Enter age*</h4>
<BInput type="int" name="age" required/>
<BButton onClick={formSubmit} whenFormOK>Submit</BButton>
</BindForm>
</Layout>
npm install @astro-utils/forms
Add the middleware to your server
src/middleware.ts
import astroForms from "@astro-utils/forms";
import {sequence} from "astro/middleware";
export const onRequest = sequence(astroForms());
Add the WebForms
component in the layout
layouts/Layout.astro
---
import {WebForms} from '@astro-utils/forms/forms.js';
---
<WebForms>
<slot/>
</WebForms>
This changes astro behavior to allow the form to work, it ensure the components render by the order they are in the file.
astro.config.mjs
import { defineConfig } from 'astro/config';
import astroForms from "@astro-utils/forms/dist/integration.js";
export default defineConfig({
output: 'server',
integrations: [astroForms]
});
pages/index.astro
---
import { Bind, BindForm, FormErrors, BButton, BInput, BOption, BSelect, BTextarea } from "@astro-utils/forms/forms.js";
import Layout from "../layouts/Layout.astro";
type formType = {
name: string,
age: number,
about?: string
favoriteFood?: 'Pizaa' | 'Salad' | 'Lasagna'
}
const form = Bind<formType>();
let showSubmitText: string;
function formSubmit(){
showSubmitText = `You name is ${form.name}, you are ${form.age} years old. `;
if(form.about){
showSubmitText += `\n\n${form.about}\n\n`;
}
if(form.favoriteFood){
showSubmitText += `Your favorite food is ${form.favoriteFood}`;
}
}
---
<Layout>
<BindForm bind={form}>
<FormErrors title="Form Errors"/>
<h4>What you name*</h4>
<BInput type={'text'} name="name" maxlength={20} required/>
<h4>Enter age*</h4>
<BInput type={'int'} name="age" required/>
<h4>Tell about yourself</h4>
<BTextarea name="about" maxlength={300}></BTextarea>
<h4>What you favorite food?</h4>
<BSelect name="favoriteFood" required={false}>
<BOption disabled selected>Idk</BOption>
<BOption>Pizaa</BOption>
<BOption>Salad</BOption>
<BOption>Lasagna</BOption>
</BSelect>
<BButton onClick={formSubmit} whenFormOK>Submit</BButton>
{showSubmitText && <>
<h3>You submitted the form:</h3>
<div style="white-space: pre;">{showSubmitText}</div>
</>}
</BindForm>
</Layout>
You can also use this as a simple on click hook
---
import { BButton } from "@astro-utils/forms/forms.js";
import { Button } from 'reactstrap';
const { session } = Astro.locals;
function increaseCounter() {
session.counter ??= 0
session.counter++
}
---
<Layout>
<BButton as={Button} props={{color: 'info'}} onClick={increaseCounter}>++</BButton>
{session.counter}
<Layout/>
The session.counter
will show the last value and not the update value.
This is because the output is not reactive. You can use it inside BindForm
to make it reactive.
FAQs
Server component for Astro (call server functions from client side with validation and state management)
We found that @astro-utils/forms demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.