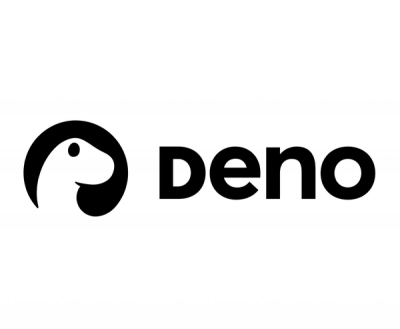
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@atek-cloud/adb-api
Advanced tools
atek.cloud/adb-api
npm install @atek-cloud/adb-api
import adb from '@atek-cloud/adb-api'
import cats from 'example-cats-table'
// get or create a database under the 'mydb' alias
const db = adb.db('mydb')
// use the cats table
await cats(db).create({id: 'kit', name: 'Kit'})
await cats(db).list() // => {records: [{key: 'kit', value: {id: 'kit', name: 'Kit', createdAt: '2021-09-07T01:06:07.487Z'}}]}
await cats(db).get('kit') // => {key: 'kit', value: {id: 'kit', name: 'Kit', createdAt: '2021-09-07T01:06:07.487Z'}}
await cats(db).put('kit', {id: 'kit', name: 'Kitty'})
await cats(db).delete('kit')
See The Atek DB Guide to learn how to use ADB.
Name | Type |
---|---|
api | AdbApi & AtekRpcClient |
db | (dbId : string | DbConfig , opts? : DbConfig ) => AdbDatabase |
Use the .db()
method to create AdbDatabase
instances. You may pass a Hypercore 64-character hex-string key or an arbitrary string (which will be a local alias).
import adb from '@atek-cloud/adb-api'
// get or create a database under the 'mydb' alias
// - the 'mydb' alias will be stored under this application for easy future access
const db = adb.db('mydb')
// get a database under its Hypercore key
// - if the database does not exist locally, its content will be fetched from the p2p network
const db2 = adb.db('97396e81e407e5ae7a64b375cc54c1fc1a0d417a5a72e2169b5377506e1e3163')
The .api
is the RPC interface which will be used by .db()
.
▸ defineSchema<T
>(path
, opts?
): (db
: AdbDatabase
) => any
Name | Type |
---|---|
path | string | string [] |
opts? | AdbSchemaOpts |
fn
▸ (db
): any
Use this function to create reusable record schemas.
import adb, { defineSchema } from '@atek-cloud/adb-api`
interface CatRecord {
id: string
name: string
createdAt: string
}
const cats = defineSchema<CatRecord>('example.com/cats', {
pkey: '/id',
jsonSchema: {
type: 'object',
required: ['id', 'name']
properties: {
id: {type: 'string'},
name: {type: 'string'},
createdAt: {type: 'string', format: 'date-time'}
}
}
})
await cats(adb.db('mydb')).create({
id: 'kit',
name: 'Kit the Cat'
})
▸ createClient(): AdbApi
& AtekRpcClient
AdbApi
& AtekRpcClient
Creates an AdbApi
instance. You can typically use the .api
exported on the default object, but if you need to configure a separate API instance you can use this function.
▸ createServer(handlers
): AtekRpcServer
Name | Type |
---|---|
handlers | any |
AtekRpcServer
Creates an AtekRpcServer
server. You would only ever need this if creating your own ADB server (perhaps for test mocking).
• new AdbDatabase(api
, dbId
, opts?
)
Name | Type |
---|---|
api | AdbApi |
dbId | string |
opts? | DbConfig |
• isReady: Promise
<any
>
• api: AdbApi
• dbId: string
▸ describe(): Promise
<DbInfo
>
desc
Get metadata and information about the database.
Promise
<DbInfo
>
▸ list(path
, opts?
): Promise
<Object
>
desc
List records in a table.
Name | Type |
---|---|
path | string | string [] |
opts? | ListOpts |
Promise
<Object
>
▸ get(path
): Promise
<Record
<object
>>
desc
Get a record in a table.
Name | Type |
---|---|
path | string | string [] |
Promise
<Record
<object
>>
▸ put(path
, value
): Promise
<Record
<object
>>
desc
Write a record to a table.
Name | Type |
---|---|
path | string | string [] |
value | object |
Promise
<Record
<object
>>
▸ delete(path
): Promise
<void
>
desc
Delete a record from a table.
Name | Type |
---|---|
path | string | string [] |
Promise
<void
>
Name | Type |
---|---|
T | extends object |
• new AdbSchema<T
>(db
, path
, opts?
)
Name | Type |
---|---|
T | extends object |
Name | Type |
---|---|
db | AdbDatabase |
path | string | string [] |
opts? | AdbSchemaOpts |
• path: string
[]
• isReady: Promise
<any
>
• Optional
pkey: string
| string
[]
• pkeyFn: PkeyFunction
• Optional
jsonSchema: object
• Optional
validator: Validator
• db: AdbDatabase
▸ list(opts?
): Promise
<Object
>
desc
List records in the schema.
Name | Type |
---|---|
opts? | ListOpts |
Promise
<Object
>
▸ get(key
, opts?
): Promise
<undefined
| Record
<T
>>
desc
Get a record in the schema space.
Name | Type |
---|---|
key | string |
opts? | ValidationOpts |
Promise
<undefined
| Record
<T
>>
▸ create(value
, opts?
): Promise
<undefined
| Record
<T
>>
desc
Add a record to the schema space.
Name | Type |
---|---|
value | T |
opts? | ValidationOpts |
Promise
<undefined
| Record
<T
>>
▸ put(key
, value
, opts?
): Promise
<undefined
| Record
<T
>>
desc
Write a record to the schema space.
Name | Type |
---|---|
key | string |
value | T |
opts? | ValidationOpts |
Promise
<undefined
| Record
<T
>>
▸ delete(key
): Promise
<void
>
desc
Delete a record from the schema space.
Name | Type |
---|---|
key | string |
Promise
<void
>
• Optional
pkey: string
| string
[]
• Optional
jsonSchema: object
FAQs
Atek DB API
We found that @atek-cloud/adb-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.