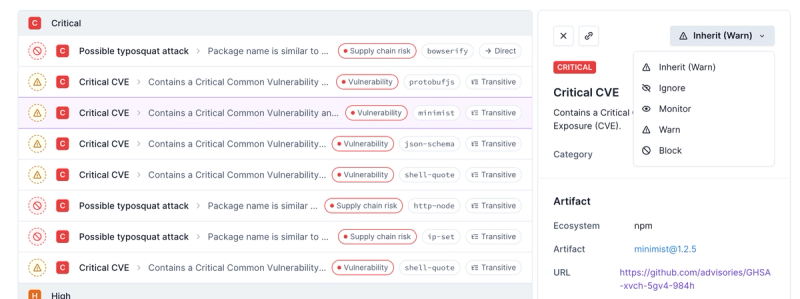
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@audius/fetch-nft
Advanced tools
Readme
@audius/fetch-nft
🖼🎑🌠
A utility to fetch and easily display Ethereum & Solana NFTs in a common format given any wallet.
built with ❤️ from the team @Audius.
# install peer dependencies if not already in your project
npm install @solana/spl-token @solana/web3.js
npm install @audius/fetch-nft
import { FetchNFTClient } from "@audius/fetch-nft";
// Initialize fetch client
const fetchClient = new FetchNFTClient();
// Fetching all collectibles for the given wallets
fetchClient
.getCollectibles({
ethWallets: ["0x5A8443f456f490dceeAD0922B0Cc89AFd598cec9"],
solWallets: ["GrWNH9qfwrvoCEoTm65hmnSh4z3CD96SfhtfQY6ZKUfY"],
})
.then((res) => console.log(res));
By default, fetch-nft uses the public Opensea API and the Solana mainnet RPC endpoint. To configure API keys and endpoints, see Usage With Configs.
FetchNFTClient is the primary interface for using the library. When initializing the client, you may optionally pass in configs for the OpenSea and Helius clients used internally.
type OpenSeaConfig = {
apiEndpoint?: string;
apiKey?: string;
assetLimit?: number;
eventLimit?: number;
};
type HeliusConfig = {
apiEndpoint?: string;
apiKey?: string;
limit?: number;
};
type FetchNFTClientProps = {
openSeaConfig?: OpenSeaConfig;
heliusConfig?: HeliusConfig;
solanaConfig?: {
rpcEndpoint?: string;
metadataProgramId?: string;
};
};
Getting Ethereum collectibles:
FetchNFTClient::getEthereumCollectibles(wallets: string[]) => Promise<CollectibleState>
Getting Solana collectibles:
FetchNFTClient::getSolanaCollectibles(wallets: string[]) => Promise<CollectibleState>
Getting all collectibles:
FetchNFTClient::getCollectibles({
ethWallets?: string[],
solWallets?: string[]
}) => Promise<{
ethCollectibles: CollectibleState
solCollectibles: CollectibleState
}>
type Collectible = {
id: string;
tokenId: string;
name: Nullable<string>;
description: Nullable<string>;
mediaType: CollectibleMediaType;
frameUrl: Nullable<string>;
imageUrl: Nullable<string>;
gifUrl: Nullable<string>;
videoUrl: Nullable<string>;
threeDUrl: Nullable<string>;
animationUrl: Nullable<string>;
hasAudio: boolean;
isOwned: boolean;
dateCreated: Nullable<string>;
dateLastTransferred: Nullable<string>;
externalLink: Nullable<string>;
permaLink: Nullable<string>;
chain: Chain;
wallet: string;
duration?: number;
// ethereum nfts
assetContractAddress: Nullable<string>;
standard: Nullable<EthTokenStandard>;
collectionSlug: Nullable<string>;
collectionName: Nullable<string>;
collectionImageUrl: Nullable<string>;
// solana nfts
solanaChainMetadata?: Nullable<Metadata>;
heliusCollection?: Nullable<HeliusCollection>;
};
type CollectibleState = {
[wallet: string]: Collectible[];
};
import { FetchNFTClient } from '@audius/fetch-nft'
// OpenSea Config
const openSeaConfig = {
apiEndpoint: '...',
apiKey: '...',
assetLimit: 10,
eventLimit: 10
}
// Helius Config
const heliusConfig = {
apiEndpoint: '...';
apiKey: '...',
limit: 10
}
const solanaConfig = {
rpcEndpoint: '...',
metadataProgramId: '...'
};
// Initialize fetch client with configs
const fetchClient = new FetchNFTClient({ openSeaConfig, heliusConfig, solanaConfig })
// Fetching Ethereum collectibles for the given wallets
fetchClient.getEthereumCollectibles([...]).then(res => console.log(res))
// Fetching Solana collectibles for the given wallets
fetchClient.getSolanaCollectibles([...]).then(res => console.log(res))
For more examples, see the /examples directory
FAQs
A utility to fetch Ethereum & Solana NFTs
The npm package @audius/fetch-nft receives a total of 178 weekly downloads. As such, @audius/fetch-nft popularity was classified as not popular.
We found that @audius/fetch-nft demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.