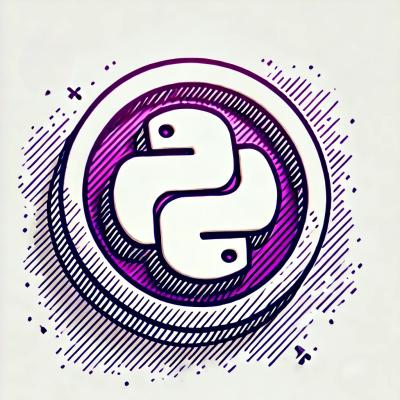
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@autonomys/auto-consensus
Advanced tools

The Autonomys Auto Consensus SDK (@autonomys/auto-consensus
) offers a suite of functions for interacting with the Autonomys Network's consensus layer. It enables developers to:
Install the package via npm or yarn:
# Using npm
npm install @autonomys/auto-consensus
# Using yarn
yarn add @autonomys/auto-consensus
You can import specific functions from the package as needed:
import {
account,
balance,
totalIssuance,
transfer,
registerOperator,
nominateOperator,
blockNumber,
blockHash,
networkTimestamp,
domains,
domainStakingSummary,
latestConfirmedDomainBlock,
} from '@autonomys/auto-consensus'
Below are examples demonstrating how to use the functions provided by @autonomys/auto-consensus
.
Get detailed account information, including the nonce and balance data.
import { account } from '@autonomys/auto-consensus'
import { activate } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'your_network_id' })
const accountData = await account(api, 'your_address')
console.log(`Nonce: ${accountData.nonce}`)
console.log(`Free Balance: ${accountData.data.free}`)
console.log(`Reserved Balance: ${accountData.data.reserved}`)
await api.disconnect()
})()
Parameters:
api
(ApiPromise): Connected API instance.address
(string): The account address.Returns:
nonce
: The account's transaction nonce.data
: An object with balance details (free
, reserved
, miscFrozen
, feeFrozen
).Get the free balance of an account.
import { balance } from '@autonomys/auto-consensus'
import { activate } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'your_network_id' })
const accountBalance = await balance(api, 'your_address')
console.log(`Free Balance: ${accountBalance.free}`)
await api.disconnect()
})()
Retrieve the total token issuance in the network.
import { totalIssuance } from '@autonomys/auto-consensus'
;(async () => {
const total = await totalIssuance('your_network_id')
console.log(`Total Issuance: ${total.toString()}`)
})()
Parameters:
networkId
(string, optional): The network ID.Returns:
Transfer tokens from one account to another.
import { transfer, events } from '@autonomys/auto-consensus'
import { activate, activateWallet, signAndSendTx, disconnect } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'your_network_id' })
const { accounts } = await activateWallet({
networkId: 'your_network_id',
mnemonic: 'your_mnemonic',
})
const sender = accounts[0]
const recipientAddress = 'recipient_address'
const amount = '1000000000000' // Amount in smallest units (e.g., wei)
const tx = await transfer(api, recipientAddress, amount)
// Sign and send the transaction
await signAndSendTx(sender, tx, [events.transfer])
console.log(`Transferred ${amount} tokens to ${recipientAddress}`)
await disconnect(api)
})()
Parameters:
api
(ApiPromise): Connected API instance.recipient
(string): Recipient's address.amount
(number | string | BN): Amount to transfer.Returns:
Register a new operator for staking.
import { registerOperator, events } from '@autonomys/auto-consensus'
import { activate, activateWallet, signAndSendTx } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'your_network_id' })
// Sender's account (who will register the operator)
const { accounts: senderAccounts } = await activateWallet({
networkId: 'your_network_id',
mnemonic: 'sender_mnemonic',
})
const sender = senderAccounts[0]
// Operator's account
const { accounts: operatorAccounts } = await activateWallet({
networkId: 'your_network_id',
mnemonic: 'operator_mnemonic',
})
const operatorAccount = operatorAccounts[0]
const tx = await registerOperator({
api,
senderAddress: sender.address,
Operator: operatorAccount,
domainId: '0', // Domain ID where the operator will be registered
amountToStake: '1000000000000000000', // Amount in smallest units
minimumNominatorStake: '10000000000000000',
nominationTax: '5', // Percentage as a string (e.g., '5' for 5%)
})
// Sign and send the transaction
await signAndSendTx(sender, tx, [events.operatorRegistered])
console.log('Operator registered successfully')
})()
Parameters:
api
(ApiPromise): Connected API instance.senderAddress
(string): Address of the sender registering the operator.Operator
(KeyringPair): Key pair of the operator account.domainId
(string): ID of the domain.amountToStake
(string): Amount to stake in smallest units.minimumNominatorStake
(string): Minimum stake required from nominators.nominationTax
(string): Percentage tax for nominations.Returns:
Nominate an existing operator by staking tokens.
import { nominateOperator, events } from '@autonomys/auto-consensus'
import { activate, activateWallet, signAndSendTx } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'your_network_id' })
const { accounts } = await activateWallet({
networkId: 'your_network_id',
mnemonic: 'nominator_mnemonic',
})
const nominator = accounts[0]
const operatorId = '1' // The ID of the operator to nominate
const amountToStake = '5000000000000000000' // Amount in smallest units
const tx = await nominateOperator({
api,
operatorId,
amountToStake,
})
// Sign and send the transaction
await signAndSendTx(nominator, tx, [events.operatorNominated])
console.log(`Nominated operator ${operatorId} with ${amountToStake} stake`)
})()
Parameters:
api
(ApiPromise): Connected API instance.operatorId
(string): ID of the operator to nominate.amountToStake
(string): Amount to stake in smallest units.Returns:
Retrieve the current block number, block hash, and network timestamp.
import { blockNumber, blockHash, networkTimestamp } from '@autonomys/auto-consensus'
;(async () => {
const currentBlockNumber = await blockNumber()
const currentBlockHash = await blockHash()
const currentTimestamp = await networkTimestamp()
console.log(`Current Block Number: ${currentBlockNumber}`)
console.log(`Current Block Hash: ${currentBlockHash}`)
console.log(`Network Timestamp: ${currentTimestamp}`)
})()
Functions:
blockNumber()
: Returns the current block number as a BigInt
.blockHash()
: Returns the current block hash as a hex string.networkTimestamp()
: Returns the network timestamp as a BigInt
.Get the list of domains registered on the network.
import { domains } from '@autonomys/auto-consensus'
import { activate } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'your_network_id' })
const domainList = await domains(api)
domainList.forEach((domain) => {
console.log(`Domain ID: ${domain.id}`)
console.log(`Owner Address: ${domain.owner}`)
console.log(`Creation Block: ${domain.creationBlock}`)
// ...other domain properties
})
await api.disconnect()
})()
Retrieve staking summaries for all domains.
import { domainStakingSummary } from '@autonomys/auto-consensus'
import { activate } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'your_network_id' })
const stakingSummaries = await domainStakingSummary(api)
stakingSummaries.forEach((summary) => {
console.log(`Domain ID: ${summary.domainId}`)
console.log(`Total Stake: ${summary.totalStake}`)
// ...other summary properties
})
await api.disconnect()
})()
Fetch the latest confirmed blocks for each domain.
import { latestConfirmedDomainBlock } from '@autonomys/auto-consensus'
import { activate } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'your_network_id' })
const confirmedBlocks = await latestConfirmedDomainBlock(api)
confirmedBlocks.forEach((blockInfo) => {
console.log(`Domain ID: ${blockInfo.id}`)
console.log(`Block Number: ${blockInfo.number}`)
console.log(`Block Hash: ${blockInfo.hash}`)
// ...other block properties
})
await api.disconnect()
})()
account(api: Api, address: string): Promise<AccountData>
Retrieve detailed account information.
api
(Api
): Connected API instance.address
(string
): The account address.Promise<AccountData>
containing nonce
and data
with balance details.balance(api: Api, address: string): Promise<BalanceData>
Get the balance data of an account.
api
(Api
): Connected API instance.address
(string
): The account address.Promise<BalanceData>
with balance details.totalIssuance(networkId?: string): Promise<Codec>
Retrieve the total token issuance in the network.
networkId
(string
, optional): The network ID.Promise<Codec>
representing the total issuance.transfer(api: ApiPromise, recipient: string, amount: BN | string | number): SubmittableExtrinsic
Create a transfer transaction.
api
(ApiPromise
): Connected API instance.recipient
(string
): Recipient's address.amount
(BN | string | number
): Amount to transfer.SubmittableExtrinsic
transaction object.registerOperator(params: RegisterOperatorParams): Promise<SubmittableExtrinsic>
Register a new operator.
params
(RegisterOperatorParams
): Parameters for operator registration.Promise<SubmittableExtrinsic>
nominateOperator(params: NominateOperatorParams): Promise<SubmittableExtrinsic>
Nominate an existing operator.
params
(NominateOperatorParams
): Parameters for nominating an operator.Promise<SubmittableExtrinsic>
blockNumber(): Promise<bigint>
Get the current block number.
Promise<bigint>
blockHash(): Promise<string>
Get the current block hash.
Promise<string>
networkTimestamp(): Promise<bigint>
Get the network timestamp.
Promise<bigint>
domains(api: Api): Promise<DomainRegistry[]>
Retrieve the list of domains.
api
(Api
): Connected API instance.Promise<DomainRegistry[]>
domainStakingSummary(api: Api): Promise<DomainStakingSummary[]>
Get staking summaries for domains.
api
(Api
): Connected API instance.Promise<DomainStakingSummary[]>
latestConfirmedDomainBlock(api: Api): Promise<ConfirmedDomainBlock[]>
Fetch the latest confirmed domain blocks.
api
(Api
): Connected API instance.Promise<ConfirmedDomainBlock[]>
When using @autonomys/auto-consensus
, make sure to handle errors, especially when interacting with network operations.
Example:
import { account } from '@autonomys/auto-consensus'
import { activate } from '@autonomys/auto-utils'
;(async () => {
try {
const api = await activate({ networkId: 'your_network_id' })
const accountData = await account(api, 'your_address')
// Use accountData...
await api.disconnect()
} catch (error) {
console.error('Error occurred:', error)
}
})()
We welcome contributions to @autonomys/auto-consensus
! Please follow these guidelines:
Fork the repository on GitHub.
Clone your fork locally:
git clone https://github.com/your-username/auto-sdk.git
cd auto-sdk/packages/auto-consensus
Install dependencies:
yarn install
Make your changes and ensure all tests pass:
yarn test
Commit your changes with clear and descriptive messages.
Push to your fork and create a pull request against the main
branch of the original repository.
yarn lint
to ensure code style consistency.This project is licensed under the MIT License. See the LICENSE file for details.
auto-consensus
can be found in @autonomys/auto-utils
.If you have any questions or need support, feel free to reach out:
We appreciate your feedback and contributions!
FAQs

The npm package @autonomys/auto-consensus receives a total of 82 weekly downloads. As such, @autonomys/auto-consensus popularity was classified as not popular.
We found that @autonomys/auto-consensus demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.