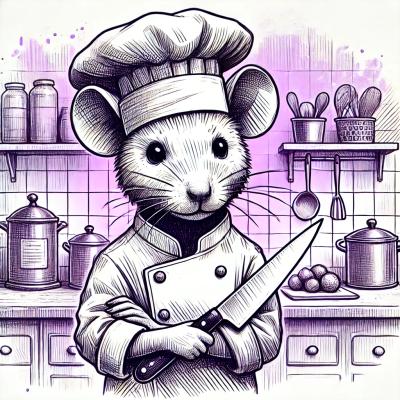
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@avalabs/bridge-unified
Advanced tools
<img alt="Avalanche Logo" src="https://images.ctfassets.net/gcj8jwzm6086/Gse8dqDEnJtT87RsbbEf4/1609daeb09e9db4a6617d44623028356/Avalanche_Horizontal_White.svg" width="au
A package for routing tokens from Chain A to Chain B, and unifying multiple bridge tools into one.
The bridging ecosystem is complex. There are often multiple tools that can be used to bridge tokens from one chain to another, and sometimes to get a token from chain A to C you need to use multiple bridging tools and route through chain B first. This package simplifies that process by creating the Unified Bridge API, a standard interface for bridging tokens from one chain to another without having to worry about the underlying tools or the underlying intermediary chains.
These are the bridges we currently support:
bridges/cctp
folder.Future bridges we plan to support:
npm install @avalabs/bridge-unified
# or
yarn add @avalabs/bridge-unified
# or
pnpm add @avalabs/bridge-unified
import { createUnifiedBridgeService, getEnabledBridgeServices, Environment, BridgeTransfer } from '@avalabs/bridge-unified';
const environment = Environment.TEST;
const evmSigner: EvmSigner = {
sign: async ({ data, from, to, value }) => {
return await window.ethereum.request({
method: 'eth_sendTransaction,',
params:{
account: from,
data: data ?? undefined,
from,
to: to ?? null,
chain: undefined,
value
}
});
},
};
const btcSigner: BtcSigner = {
sign: async ({ inputs, outputs }) => {
return await window.ethereum.request({
method: 'bitcoin_signTransaction',
params: { inputs, outputs },
});
},
};
const cctpInitializer: EvmBridgeInitializer = {
type: BridgeType.CCTP,
signer: evmSigner,
};
const icttErc20Initializer: EvmBridgeInitializer = {
type: BridgeType.ICTT_ERC20_ERC20,
signer: evmSigner,
};
const avalancheEvmInitializer: EvmBridgeInitializer = {
type: BridgeType.AVALANCHE_EVM,
signer: evmSigner,
};
const avalancheBtcInitializer: AvaToBtcBridgeInitializer = {
type: BridgeType.AVALANCHE_AVA_BTC,
signer: evmSigner,
bitcoinFunctions: bitcoinProvider as BitcoinFunctions,
};
const bitcoinAvaInitializer: BtcToAvaBridgeInitializer = {
type: BridgeType.AVALANCHE_BTC_AVA,
signer: btcSigner,
bitcoinFunctions: bitcoinProvider as BitcoinFunctions,
};
// use all available bridges
const enabledBridgeInitializers: BridgeInitializer[] = [
cctpInitializer,
icttErc20Initializer,
avalancheEvmInitializer,
avalancheBtcInitializer,
bitcoinAvaInitializer,
]
// fetch all available bridge services
const enabledBridgeServices = await getEnabledBridgeServices(environment, disabledBridgeTypes);
// create a new service for a given environment and list of bridge services
const unifiedService = createUnifiedBridgeService({
environment,
enabledBridgeServices
});
// get the list of supported assets, grouped by caip2 (https://chainagnostic.org/CAIPs/caip-2) chain IDs
const assets = await unifiedService.getAssets()
// get the bridge fee(s) of the provided transfer
const fees = await unifiedService.getFees({...})
// start a new bridge transfer and store its state
const bridgeTransfer = await unifiedService.transferAsset({...})
// create an update listener for tracking
const updateListener = (transfer: BridgeTransfer) => {
console.log(transfer)
}
// start tracking the transfer's state. whenever the state changes, it will call the provided `updateListener`
const { cancel, result } = await unifiedService.trackTransfer({bridgeTransfer, updateListener, ...})
// immediately stops tracking and rejects the tracker's promise
// cancel()
// wait for the transfer to finish and get its final state
const finalizedBridgeTransfer = await result
Type: (environment: Environment, enabledBridgeInitializers: BridgeInitializer[]) => Promise<BridgeServicesMap>
Returns all available bridge services for a given environment (excluding disabledBridgeTypes). Any bridge service which fails to initialize will be absent from this returned value.
Type: Environment
Defines if the bridge service should use testnet
or mainnet
.
Type: BridgeInitializer[]
Enables the integration of the provided BridgeType
s based on each BridgeInitializer
.
Type: BridgeServicesMap
=> Map<BridgeType, BridgeService>
This includes all the bridge services which were initialized successfully, to pass to createUnifiedBridgeService
.
Returns a new unifiedBridgeService
for the given environment
and enabledBridgeServices
map.
Contains all the required properties and methods to prepare, initiate or track a bridge transfer. Automatically picks the right (enabled) bridge integration to use based on the provided params.
{
environment, // the provided Environment during initialization
getAssets,
getFees,
estimateGas,
canTransferAsset,
transferAsset,
trackTransfer,
}
Type: () => Promise<ChainAssetMap>
Returns the aggregated list of assets supported by the enabled bridges grouped by caip2 chain IDs.
Type: (params: FeeParams) => Promise<AssetFeeMap>
Calculates and returns the bridge fees in tokenAddress
- amount
pairs for a given bridge transfer.
Type: (params: TransferParams) => Promise<bigint>
Estimates the gas cost of a specific transfer.
Type: (params: FeeParams) => Promise<bigint>
Calculates and returns the minimum transfer amount for a given bridge transfer.
Type: (params: TransferParams) => Promise<BridgeTransfer>
Starts a new bridge transfer, executing every required step in a single call.
Transactions signing is done by a custom sign
callback. The custom sign
implementation may use their own solution or the default dispatch
callback to submit the transaction to the network.
Returns a BridgeTransfer
containing all the (known) initial values such as: environment, addresses, amount, fee, transaction hash, required and actual block confirmation counts, etc.
Notes about TransferParams: fromAddress: The address where the bridge amount is from.
toAddress: The address where the bridge amount is going to end up.
For example, A user has an account with AddressC and AddressBtc. The user wants to bridge some funds from Ethereum to Avalanche using the same address. FromAddress and toAddress will be both AddressC.
The user wants to bridge some funds from Bitcoin to Avalanche. FromAddress is AddressBtc and toAddress is AddressC.
Some bridges allows you to bridge the tokens to a different address. (CCTP and ICTT ERC20). In this case, fromAddress is the address of the token is getting bridged from. And toAddress is the address which is going to receive the bridged funds.
Type: (params: TrackingParams) => ({cancel, result})
Tracks the given BridgeTransfer
's progress and invokes the provided listener callback whenever a change happens.
Type: () => void
If it's still pending, rejects the tracker's promise (result
) immediately and breaks its loop under the hood.
Type: Promise<BridgeTransfer>
Resolves with the finalized BridgeTransfer
(if not canceled before).
FAQs
<img alt="Avalanche Logo" src="https://images.ctfassets.net/gcj8jwzm6086/Gse8dqDEnJtT87RsbbEf4/1609daeb09e9db4a6617d44623028356/Avalanche_Horizontal_White.svg" width="au
The npm package @avalabs/bridge-unified receives a total of 730 weekly downloads. As such, @avalabs/bridge-unified popularity was classified as not popular.
We found that @avalabs/bridge-unified demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.