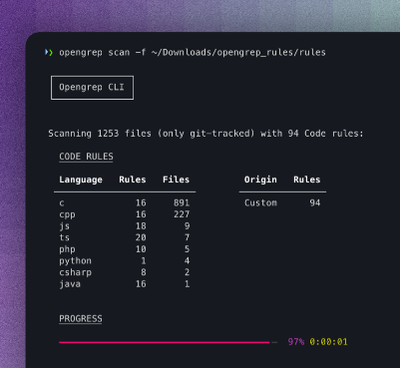
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
@aws-amplify/predictions
Advanced tools
@aws-amplify/predictions is a part of the AWS Amplify library that provides machine learning capabilities to your web and mobile applications. It allows you to easily integrate features like text translation, speech generation, text recognition, and more using AWS services.
Text Translation
This feature allows you to translate text from one language to another. In the code sample, the text 'Hello, how are you?' is translated from English to Spanish.
const { Predictions } = require('@aws-amplify/predictions');
Predictions.convert({
translateText: {
source: {
text: "Hello, how are you?",
language: "en"
},
targetLanguage: "es"
}
}).then(result => {
console.log(result.text);
}).catch(err => {
console.error(err);
});
Speech Generation
This feature converts text into speech. The code sample demonstrates converting the text 'Hello, how are you?' into an audio stream.
const { Predictions } = require('@aws-amplify/predictions');
Predictions.convert({
textToSpeech: {
source: {
text: "Hello, how are you?"
}
}
}).then(result => {
console.log(result.audioStream);
}).catch(err => {
console.error(err);
});
Text Recognition
This feature allows you to recognize text from an image. The code sample shows how to identify text from an image located at 'path/to/image.jpg'.
const { Predictions } = require('@aws-amplify/predictions');
Predictions.identify({
text: {
source: {
key: "path/to/image.jpg"
}
}
}).then(result => {
console.log(result.text);
}).catch(err => {
console.error(err);
});
The aws-sdk package is the official AWS SDK for JavaScript. It provides a wide range of AWS services, including those used by @aws-amplify/predictions. However, it requires more configuration and setup compared to the simplified interface provided by @aws-amplify/predictions.
Tesseract.js is a pure JavaScript OCR (Optical Character Recognition) library. It provides text recognition capabilities similar to the text recognition feature in @aws-amplify/predictions but does not offer other machine learning functionalities like text translation or speech generation.
This package contains the AWS Amplify Predictions category. For more information on using Predictions in your application please reference the Amplify Dev Center.
FAQs
Machine learning category of aws-amplify
We found that @aws-amplify/predictions demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.