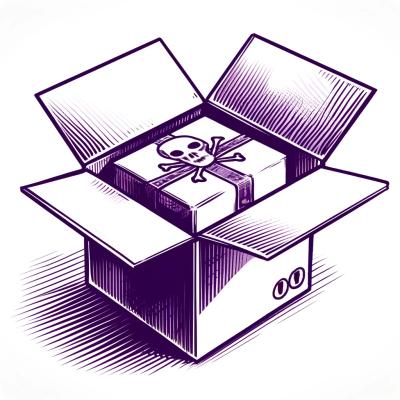
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@aws-amplify/storage
Advanced tools
@aws-amplify/storage is a package that provides a simple and powerful way to manage user files and data in Amazon S3. It is part of the AWS Amplify library, which is designed to help developers build cloud-enabled applications. The package offers functionalities such as uploading, downloading, and managing files in S3, as well as configuring access levels and handling file metadata.
Upload a File
This feature allows you to upload a file to an S3 bucket. The code sample demonstrates how to upload a text file with the content 'Hello, world!' to S3 using the Storage.put method.
const { Storage } = require('@aws-amplify/storage');
async function uploadFile(file) {
try {
const result = await Storage.put('example.txt', file, {
contentType: 'text/plain'
});
console.log('File uploaded successfully:', result);
} catch (error) {
console.error('Error uploading file:', error);
}
}
uploadFile('Hello, world!');
Download a File
This feature allows you to download a file from an S3 bucket. The code sample demonstrates how to download a file named 'example.txt' from S3 using the Storage.get method and log its content.
const { Storage } = require('@aws-amplify/storage');
async function downloadFile() {
try {
const file = await Storage.get('example.txt', { download: true });
console.log('File downloaded successfully:', file.Body.toString('utf-8'));
} catch (error) {
console.error('Error downloading file:', error);
}
}
downloadFile();
List Files
This feature allows you to list all files in an S3 bucket. The code sample demonstrates how to list all files using the Storage.list method and log the result.
const { Storage } = require('@aws-amplify/storage');
async function listFiles() {
try {
const files = await Storage.list('');
console.log('Files in bucket:', files);
} catch (error) {
console.error('Error listing files:', error);
}
}
listFiles();
Delete a File
This feature allows you to delete a file from an S3 bucket. The code sample demonstrates how to delete a file named 'example.txt' from S3 using the Storage.remove method.
const { Storage } = require('@aws-amplify/storage');
async function deleteFile() {
try {
await Storage.remove('example.txt');
console.log('File deleted successfully');
} catch (error) {
console.error('Error deleting file:', error);
}
}
deleteFile();
The aws-sdk package is the official AWS SDK for JavaScript. It provides a comprehensive set of tools for interacting with AWS services, including S3. While it offers more granular control and a wider range of functionalities compared to @aws-amplify/storage, it requires more setup and configuration.
The s3 package is a lightweight wrapper around the AWS SDK specifically for S3 operations. It simplifies common tasks such as uploading and downloading files, but it does not offer the same level of integration with other AWS services as @aws-amplify/storage.
The s3-upload-stream package provides a streaming interface for uploading large files to S3. It is useful for handling large file uploads that might not fit into memory, but it lacks the broader functionality and ease of use provided by @aws-amplify/storage.
INTERNAL USE ONLY
This package contains the AWS Amplify Storage category and is intended for internal use only. To integrate Amplify into your app, please use aws-amplify.
FAQs
Storage category of aws-amplify
We found that @aws-amplify/storage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.