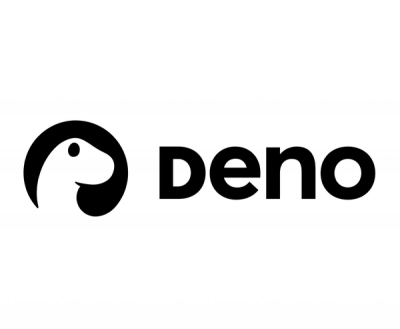
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@aws-cdk/aws-events
Advanced tools
Amazon CloudWatch Events delivers a near real-time stream of system events that describe changes in AWS resources. For example, an AWS CodePipeline emits the State Change event when the pipeline changes it's state.
The EventRule
construct defines a CloudWatch events rule which monitors an
event based on an event
pattern
and invoke event targets when the pattern is matched against a triggered
event. Event targets are objects that implement the IEventTarget
interface.
Normally, you will use one of the source.onXxx(name[, target[, options]]) -> EventRule
methods on the event source to define an event rule associated with
the specific activity. You can targets either via props, or add targets using
rule.addTarget
.
For example, to define an rule that triggers a CodeBuild project build when a commit is pushed to the "master" branch of a CodeCommit repository:
const onCommitRule = repo.onCommit('OnCommitToMaster', project, 'master');
You can add additional targets, with optional input
transformer
using eventRule.addTarget(target[, input])
. For example, we can add a SNS
topic target which formats a human-readable message for the commit.
For example, this adds an SNS topic as a target:
onCommitRule.addTarget(topic, {
template: 'A commit was pushed to the repository <repo> on branch <branch>',
pathsMap: {
branch: '$.detail.referenceName',
repo: '$.detail.repositoryName'
}
});
0.23.0 (2019-02-04)
selectionPattern
to integrationResponses
(#1636) (7cdbcec), closes #1608roleName
available on IRole
(#1589) (9128390)role
property in the CloudFormation Actions has been renamed to deploymentRole
.role
property in the app-delivery
package has been renamed to deploymentRole
.FAQs
Amazon EventBridge Construct Library
The npm package @aws-cdk/aws-events receives a total of 84,088 weekly downloads. As such, @aws-cdk/aws-events popularity was classified as popular.
We found that @aws-cdk/aws-events demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.