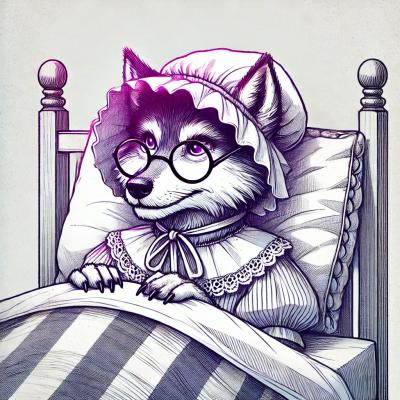
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@aws-sdk/client-elastic-load-balancing-v2
Advanced tools
AWS SDK for JavaScript Elastic Load Balancing V2 Client for Node.js, Browser and React Native
AWS SDK for JavaScript ElasticLoadBalancingV2 Client for Node.js, Browser and React Native.
Elastic Load Balancing
A load balancer distributes incoming traffic across targets, such as your EC2 instances. This enables you to increase the availability of your application. The load balancer also monitors the health of its registered targets and ensures that it routes traffic only to healthy targets. You configure your load balancer to accept incoming traffic by specifying one or more listeners, which are configured with a protocol and port number for connections from clients to the load balancer. You configure a target group with a protocol and port number for connections from the load balancer to the targets, and with health check settings to be used when checking the health status of the targets.
Elastic Load Balancing supports the following types of load balancers: Application Load Balancers, Network Load Balancers, Gateway Load Balancers, and Classic Load Balancers. This reference covers the following load balancer types:
Application Load Balancer - Operates at the application layer (layer 7) and supports HTTP and HTTPS.
Network Load Balancer - Operates at the transport layer (layer 4) and supports TCP, TLS, and UDP.
Gateway Load Balancer - Operates at the network layer (layer 3).
For more information, see the Elastic Load Balancing User Guide.
All Elastic Load Balancing operations are idempotent, which means that they complete at most one time. If you repeat an operation, it succeeds.
To install the this package, simply type add or install @aws-sdk/client-elastic-load-balancing-v2 using your favorite package manager:
npm install @aws-sdk/client-elastic-load-balancing-v2
yarn add @aws-sdk/client-elastic-load-balancing-v2
pnpm add @aws-sdk/client-elastic-load-balancing-v2
The AWS SDK is modulized by clients and commands.
To send a request, you only need to import the ElasticLoadBalancingV2Client
and
the commands you need, for example DescribeListenersCommand
:
// ES5 example
const { ElasticLoadBalancingV2Client, DescribeListenersCommand } = require("@aws-sdk/client-elastic-load-balancing-v2");
// ES6+ example
import { ElasticLoadBalancingV2Client, DescribeListenersCommand } from "@aws-sdk/client-elastic-load-balancing-v2";
To send a request, you:
send
operation on client with command object as input.destroy()
to close open connections.// a client can be shared by different commands.
const client = new ElasticLoadBalancingV2Client({ region: "REGION" });
const params = {
/** input parameters */
};
const command = new DescribeListenersCommand(params);
We recommend using await operator to wait for the promise returned by send operation as follows:
// async/await.
try {
const data = await client.send(command);
// process data.
} catch (error) {
// error handling.
} finally {
// finally.
}
Async-await is clean, concise, intuitive, easy to debug and has better error handling as compared to using Promise chains or callbacks.
You can also use Promise chaining to execute send operation.
client.send(command).then(
(data) => {
// process data.
},
(error) => {
// error handling.
}
);
Promises can also be called using .catch()
and .finally()
as follows:
client
.send(command)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
})
.finally(() => {
// finally.
});
We do not recommend using callbacks because of callback hell, but they are supported by the send operation.
// callbacks.
client.send(command, (err, data) => {
// process err and data.
});
The client can also send requests using v2 compatible style. However, it results in a bigger bundle size and may be dropped in next major version. More details in the blog post on modular packages in AWS SDK for JavaScript
import * as AWS from "@aws-sdk/client-elastic-load-balancing-v2";
const client = new AWS.ElasticLoadBalancingV2({ region: "REGION" });
// async/await.
try {
const data = await client.describeListeners(params);
// process data.
} catch (error) {
// error handling.
}
// Promises.
client
.describeListeners(params)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
});
// callbacks.
client.describeListeners(params, (err, data) => {
// process err and data.
});
When the service returns an exception, the error will include the exception information, as well as response metadata (e.g. request id).
try {
const data = await client.send(command);
// process data.
} catch (error) {
const { requestId, cfId, extendedRequestId } = error.$metadata;
console.log({ requestId, cfId, extendedRequestId });
/**
* The keys within exceptions are also parsed.
* You can access them by specifying exception names:
* if (error.name === 'SomeServiceException') {
* const value = error.specialKeyInException;
* }
*/
}
Please use these community resources for getting help. We use the GitHub issues for tracking bugs and feature requests, but have limited bandwidth to address them.
aws-sdk-js
on AWS Developer Blog.aws-sdk-js
.To test your universal JavaScript code in Node.js, browser and react-native environments, visit our code samples repo.
This client code is generated automatically. Any modifications will be overwritten the next time the @aws-sdk/client-elastic-load-balancing-v2
package is updated.
To contribute to client you can check our generate clients scripts.
This SDK is distributed under the Apache License, Version 2.0, see LICENSE for more information.
FAQs
AWS SDK for JavaScript Elastic Load Balancing V2 Client for Node.js, Browser and React Native
The npm package @aws-sdk/client-elastic-load-balancing-v2 receives a total of 40,265 weekly downloads. As such, @aws-sdk/client-elastic-load-balancing-v2 popularity was classified as popular.
We found that @aws-sdk/client-elastic-load-balancing-v2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.