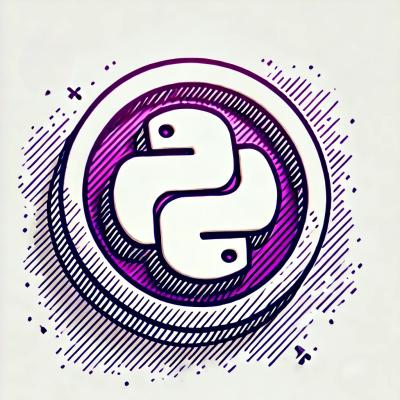
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@aws-sdk/client-kms
Advanced tools
@aws-sdk/client-kms is an AWS SDK for JavaScript package that allows you to interact with the AWS Key Management Service (KMS). This service enables you to create and manage cryptographic keys and control their use across a wide range of AWS services and in your applications.
Create a Key
This feature allows you to create a new KMS key. The code sample demonstrates how to create a key with a description, usage type, and origin.
const { KMSClient, CreateKeyCommand } = require('@aws-sdk/client-kms');
const client = new KMSClient({ region: 'us-west-2' });
const command = new CreateKeyCommand({
Description: 'My test key',
KeyUsage: 'ENCRYPT_DECRYPT',
Origin: 'AWS_KMS'
});
client.send(command).then(
(data) => console.log('Key Created:', data.KeyMetadata.KeyId),
(error) => console.error('Error:', error)
);
Encrypt Data
This feature allows you to encrypt data using a specified KMS key. The code sample demonstrates how to encrypt a plaintext string.
const { KMSClient, EncryptCommand } = require('@aws-sdk/client-kms');
const client = new KMSClient({ region: 'us-west-2' });
const command = new EncryptCommand({
KeyId: 'your-key-id',
Plaintext: Buffer.from('Hello, World!')
});
client.send(command).then(
(data) => console.log('Encrypted Data:', data.CiphertextBlob.toString('base64')),
(error) => console.error('Error:', error)
);
Decrypt Data
This feature allows you to decrypt data that was encrypted using a KMS key. The code sample demonstrates how to decrypt a base64-encoded ciphertext.
const { KMSClient, DecryptCommand } = require('@aws-sdk/client-kms');
const client = new KMSClient({ region: 'us-west-2' });
const command = new DecryptCommand({
CiphertextBlob: Buffer.from('your-encrypted-data', 'base64')
});
client.send(command).then(
(data) => console.log('Decrypted Data:', data.Plaintext.toString()),
(error) => console.error('Error:', error)
);
Generate Data Key
This feature allows you to generate a data key that you can use to encrypt data locally. The code sample demonstrates how to generate a 256-bit AES data key.
const { KMSClient, GenerateDataKeyCommand } = require('@aws-sdk/client-kms');
const client = new KMSClient({ region: 'us-west-2' });
const command = new GenerateDataKeyCommand({
KeyId: 'your-key-id',
KeySpec: 'AES_256'
});
client.send(command).then(
(data) => console.log('Data Key:', data.Plaintext.toString('base64')),
(error) => console.error('Error:', error)
);
node-forge is a JavaScript library that provides a native implementation of various cryptographic functions, including key generation, encryption, and decryption. Unlike @aws-sdk/client-kms, node-forge does not integrate with AWS services and is used for local cryptographic operations.
crypto-js is a JavaScript library that provides a variety of cryptographic algorithms for encryption, decryption, hashing, and more. Similar to node-forge, it does not integrate with AWS services and is used for local cryptographic operations.
aws-sdk is the older version of the AWS SDK for JavaScript, which includes support for KMS among many other AWS services. It is a more comprehensive package compared to @aws-sdk/client-kms, which is part of the modular v3 SDK.
FAQs
AWS SDK for JavaScript Kms Client for Node.js, Browser and React Native
The npm package @aws-sdk/client-kms receives a total of 966,836 weekly downloads. As such, @aws-sdk/client-kms popularity was classified as popular.
We found that @aws-sdk/client-kms demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.