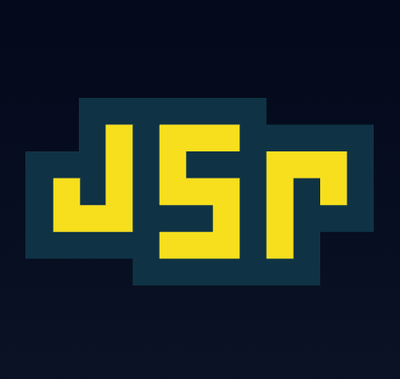
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@aws-sdk/client-s3
Advanced tools
AWS SDK for JavaScript S3 Client for Node.js, Browser and React Native
The @aws-sdk/client-s3 npm package is a client library for Amazon Simple Storage Service (S3) that allows developers to interact with S3 services using JavaScript or TypeScript. It provides methods to perform operations such as creating and managing buckets, uploading and downloading objects, and managing access permissions.
Creating a Bucket
This feature allows you to create a new S3 bucket in a specified AWS region.
const { S3Client, CreateBucketCommand } = require('@aws-sdk/client-s3');
const client = new S3Client({ region: 'us-west-2' });
const command = new CreateBucketCommand({ Bucket: 'my-bucket-name' });
client.send(command);
Uploading an Object
This feature allows you to upload an object to a specified bucket in S3.
const { S3Client, PutObjectCommand } = require('@aws-sdk/client-s3');
const client = new S3Client({ region: 'us-west-2' });
const command = new PutObjectCommand({ Bucket: 'my-bucket-name', Key: 'my-object-key', Body: 'Hello, world!' });
client.send(command);
Downloading an Object
This feature allows you to download an object from S3 by specifying the bucket and object key.
const { S3Client, GetObjectCommand } = require('@aws-sdk/client-s3');
const client = new S3Client({ region: 'us-west-2' });
const command = new GetObjectCommand({ Bucket: 'my-bucket-name', Key: 'my-object-key' });
client.send(command).then((data) => console.log(data.Body));
Listing Objects in a Bucket
This feature allows you to list all objects in a specified S3 bucket.
const { S3Client, ListObjectsCommand } = require('@aws-sdk/client-s3');
const client = new S3Client({ region: 'us-west-2' });
const command = new ListObjectsCommand({ Bucket: 'my-bucket-name' });
client.send(command).then((data) => console.log(data.Contents));
Deleting an Object
This feature allows you to delete an object from a specified S3 bucket.
const { S3Client, DeleteObjectCommand } = require('@aws-sdk/client-s3');
const client = new S3Client({ region: 'us-west-2' });
const command = new DeleteObjectCommand({ Bucket: 'my-bucket-name', Key: 'my-object-key' });
client.send(command);
The 'aws-sdk' package is the predecessor of '@aws-sdk/client-s3' and provides a comprehensive AWS SDK for JavaScript. It includes support for S3 and other AWS services. Compared to '@aws-sdk/client-s3', it is less modular and typically larger in size, which might affect bundle sizes in frontend applications.
The 's3' package is a high-level client for Amazon S3 with a more simplified API. It provides easy-to-use methods for common S3 operations. It is not an official AWS SDK, and it may not support all S3 features or the latest AWS updates as '@aws-sdk/client-s3' does.
AWS SDK for JavaScript S3 Client for Node.js, Browser and React Native.
To install the this package, simply type add or install @aws-sdk/client-s3 using your favorite package manager:
npm install @aws-sdk/client-s3
yarn add @aws-sdk/client-s3
pnpm add @aws-sdk/client-s3
The AWS SDK is modulized by clients and commands.
To send a request, you only need to import the S3Client
and
the commands you need, for example ListBucketsCommand
:
// ES5 example
const { S3Client, ListBucketsCommand } = require("@aws-sdk/client-s3");
// ES6+ example
import { S3Client, ListBucketsCommand } from "@aws-sdk/client-s3";
To send a request, you:
send
operation on client with command object as input.destroy()
to close open connections.// a client can be shared by different commands.
const client = new S3Client({ region: "REGION" });
const params = {
/** input parameters */
};
const command = new ListBucketsCommand(params);
We recommend using await operator to wait for the promise returned by send operation as follows:
// async/await.
try {
const data = await client.send(command);
// process data.
} catch (error) {
// error handling.
} finally {
// finally.
}
Async-await is clean, concise, intuitive, easy to debug and has better error handling as compared to using Promise chains or callbacks.
You can also use Promise chaining to execute send operation.
client.send(command).then(
(data) => {
// process data.
},
(error) => {
// error handling.
}
);
Promises can also be called using .catch()
and .finally()
as follows:
client
.send(command)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
})
.finally(() => {
// finally.
});
We do not recommend using callbacks because of callback hell, but they are supported by the send operation.
// callbacks.
client.send(command, (err, data) => {
// process err and data.
});
The client can also send requests using v2 compatible style. However, it results in a bigger bundle size and may be dropped in next major version. More details in the blog post on modular packages in AWS SDK for JavaScript
import * as AWS from "@aws-sdk/client-s3";
const client = new AWS.S3({ region: "REGION" });
// async/await.
try {
const data = await client.listBuckets(params);
// process data.
} catch (error) {
// error handling.
}
// Promises.
client
.listBuckets(params)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
});
// callbacks.
client.listBuckets(params, (err, data) => {
// process err and data.
});
When the service returns an exception, the error will include the exception information, as well as response metadata (e.g. request id).
try {
const data = await client.send(command);
// process data.
} catch (error) {
const { requestId, cfId, extendedRequestId } = error.$metadata;
console.log({ requestId, cfId, extendedRequestId });
/**
* The keys within exceptions are also parsed.
* You can access them by specifying exception names:
* if (error.name === 'SomeServiceException') {
* const value = error.specialKeyInException;
* }
*/
}
Please use these community resources for getting help. We use the GitHub issues for tracking bugs and feature requests, but have limited bandwidth to address them.
aws-sdk-js
on AWS Developer Blog.aws-sdk-js
.To test your universal JavaScript code in Node.js, browser and react-native environments, visit our code samples repo.
This client code is generated automatically. Any modifications will be overwritten the next time the @aws-sdk/client-s3
package is updated.
To contribute to client you can check our generate clients scripts.
This SDK is distributed under the Apache License, Version 2.0, see LICENSE for more information.
3.685.0 (2024-11-01)
FAQs
AWS SDK for JavaScript S3 Client for Node.js, Browser and React Native
The npm package @aws-sdk/client-s3 receives a total of 4,696,796 weekly downloads. As such, @aws-sdk/client-s3 popularity was classified as popular.
We found that @aws-sdk/client-s3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.