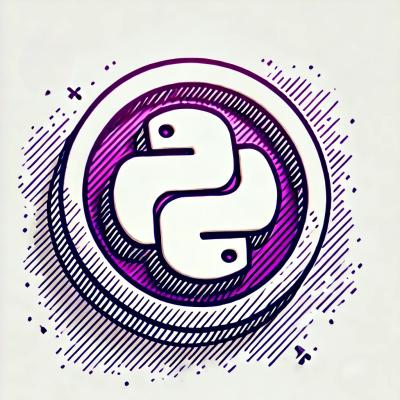
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@axe-core/react
Advanced tools
@axe-core/react is a library that integrates the axe-core accessibility testing engine with React applications. It helps developers identify and fix accessibility issues in their React components during development.
Basic Accessibility Testing
This code demonstrates how to use @axe-core/react with Jest and Testing Library to perform basic accessibility testing on a React component. It checks for accessibility violations in the rendered output of the App component.
import React from 'react';
import ReactDOM from 'react-dom';
import { axe, toHaveNoViolations } from 'jest-axe';
import { render } from '@testing-library/react';
import App from './App';
expect.extend(toHaveNoViolations);
test('should not have any accessibility violations', async () => {
const { container } = render(<App />);
const results = await axe(container);
expect(results).toHaveNoViolations();
});
Automated Accessibility Testing in Development
This code snippet shows how to integrate @axe-core/react for automated accessibility testing during development. It sets up axe to run accessibility checks on the React component tree and logs any violations to the console.
import React from 'react';
import ReactDOM from 'react-dom';
import { axe, toHaveNoViolations } from 'jest-axe';
import { render } from '@testing-library/react';
import App from './App';
if (process.env.NODE_ENV !== 'production') {
const { axe } = require('@axe-core/react');
axe(React, ReactDOM, 1000);
}
ReactDOM.render(<App />, document.getElementById('root'));
react-axe is a similar package that integrates the axe-core accessibility testing engine with React applications. It provides real-time accessibility feedback in the browser console during development. Compared to @axe-core/react, react-axe is more focused on providing immediate feedback during development rather than being used in automated tests.
eslint-plugin-jsx-a11y is an ESLint plugin that provides static analysis of JSX to identify accessibility issues. It helps enforce best practices and accessibility standards in React components. Unlike @axe-core/react, which performs runtime accessibility testing, eslint-plugin-jsx-a11y focuses on linting and static code analysis.
pa11y is an accessibility testing tool that can be used to test web pages and applications for accessibility issues. It can be integrated into CI/CD pipelines and used for automated testing. While @axe-core/react is specifically designed for React applications, pa11y is a more general tool that can be used with any web application.
Test your React application with the axe-core accessibility testing library. Results will show in the Chrome DevTools console.
Previous versions of this program were maintained at dequelabs/react-axe.
This package does not support React 18 and above. Deque released a product called axe Developer Hub. This product has numerous JavaScript/TypeScript test framework integrations and is Deque's recommended path forward for users of this library who wish to use more modern versions of React.
The product has a free plan where each licensed user gets 1 API key. This is a good option for open-source projects or solo developers looking for high-quality accessibility feedback. Sign up for the free plan.
For more information, read the blog post: Introducing axe Developer Hub, now available as part of axe DevTools for Web
Install the module from NPM or elsewhere
npm install --save-dev @axe-core/react
Call the exported function passing in the React and ReactDOM objects as well as a timing delay in milliseconds that will be observed between each component change and the time the analysis starts.
const React = require('react');
const ReactDOM = require('react-dom');
if (process.env.NODE_ENV !== 'production') {
const axe = require('@axe-core/react');
axe(React, ReactDOM, 1000);
}
Be sure to only run the module in your development environment (as shown in the code above) or else your application will use more resources than necessary when in production. You can use envify to do this as is shown in the example.
Once initialized, the module will output accessibility defect information to the Chrome Devtools console every time a component updates.
@axe-core/react will deduplicate violations using the rule that raised the violation and the CSS selector and the failureSummary of the specific node. This will ensure that each unique issue will only be printed to the console once. This can be disabled by setting disableDeduplicate: true
in the configuration object as shown in the example here.
The third argument to the exported function is the number of milliseconds to wait for component updates to cease before performing an analysis of all the changes. The changes will be batched and analyzed from the closest common ancestor of all the components that changed within the batch. This generally leads to the first analysis for a dynamic application, analyzing the entire page (which is what you want), while subsequent updates will only analyze a portion of the page (which is probably also what you want).
With version 3.0.0, @axe-core/react now runs accessibility tests inside of open Shadow DOM. You don't have to do anything special other than run @axe-core/react on an component encapsulated with open Shadow DOM (as opposed to closed). For more information, see the axe-core repo.
There is a fourth optional argument that is a configuration object for axe-core. Read about the object here: https://github.com/dequelabs/axe-core/blob/master/doc/API.md#api-name-axeconfigure
const config = {
rules: [
{
id: 'skip-link',
enabled: true
}
],
disableDeduplicate: true
};
axe(React, ReactDOM, 1000, config);
Axe-core's context object can be given as a fifth optional argument to specify which element should (and which should not) be tested. Read more from the Axe-core documentation: https://github.com/dequelabs/axe-core/blob/master/doc/API.md#context-parameter
const context = {
include: [['#preview']]
};
axe(React, ReactDOM, 1000, undefined, context);
Run a build in the example directory and start a server to see React-aXe in action in the Chrome Devtools console (opens on localhost:8888):
npm install
cd example
npm install
npm install -g http-server
npm start
Install dependencies in the root directory (which also installs them in the example directory) and then run the tests:
npm install
npm test
To debug tests in the Cypress application:
npm run test:debug
react-axe uses advanced console logging features and works best in the Chrome browser, with limited functionality in Safari and Firefox.
FAQs
Dynamic accessibility analysis for React using axe-core
The npm package @axe-core/react receives a total of 67,749 weekly downloads. As such, @axe-core/react popularity was classified as popular.
We found that @axe-core/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.