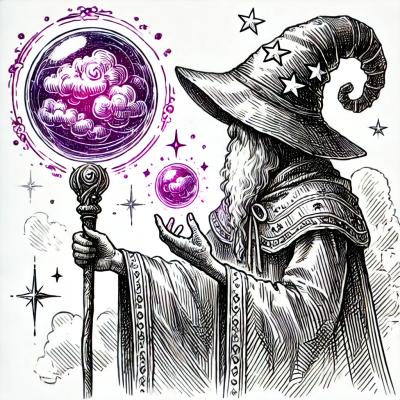
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@axflow/models
Advanced tools
Zero-dependency module to run, stream, and render results across the most popular LLMs and embedding models
Zero-dependency module to run, stream, and render results across the most popular LLMs and embedding models.
npm i @axflow/models
fetch
implementation, allowing for older node environments or request middleware (e.g., logging)import {OpenAIChat} from '@axflow/models/openai/chat';
import {CohereGenerate} from '@axflow/models/cohere/generate';
import {StreamToIterable} from '@axflow/models/utils';
const gpt4Stream = OpenAIChat.stream(
{
model: 'gpt-4',
messages: [{ role: 'user', content: 'What is the Eiffel tower?' }],
},
{
apiKey: '<openai api key>',
},
);
const cohereStream = CohereGenerate.stream(
{
model: 'command-nightly',
prompt: 'What is the Eiffel tower?',
},
{
apiKey: '<cohere api key>',
},
);
// StreamToIterable is optional in recent node versions as
// ReadableStreams already implement the async iterator protocol
for await (const chunk of StreamToIterable(gpt4Stream)) {
console.log(chunk.choices[0].delta.content);
}
for await (const chunk of StreamToIterable(cohereStream)) {
console.log(chunk.text);
}
In NextJS, it's as simple as:
import {OpenAIChat} from '@axflow/models/openai/chat';
export async function POST(request: NextRequest) {
// Byte stream is more efficient here because we do not parse the stream and
// re-encode it, but rather just pass the bytes directly through to the client.
const stream = await OpenAIChat.streamBytes(
{
model: 'gpt-4',
messages: [{ role: 'user', content: 'What is the Eiffel tower?' }],
},
{
apiKey: process.env.OPENAI_API_KEY,
},
);
return new Response(stream);
}
import {OpenAIChat} from '@axflow/models/openai/chat';
OpenAIChat.run(/* args */)
OpenAIChat.stream(/* args */)
OpenAIChat.streamBytes(/* args */)
import {OpenAICompletion} from '@axflow/models/openai/completion';
OpenAICompletion.run(/* args */)
OpenAICompletion.stream(/* args */)
OpenAICompletion.streamBytes(/* args */)
import {OpenAIEmbedding} from '@axflow/models/openai/embedding';
OpenAIEmbedding.run(/* args */)
import {CohereGeneration} from '@axflow/models/cohere/generation';
CohereGeneration.run(/* args */)
CohereGeneration.stream(/* args */)
CohereGeneration.streamBytes(/* args */)
import {CohereEmbedding} from '@axflow/models/cohere/embedding';
CohereEmbedding.run(/* args */)
import {AnthropicCompletion} from '@axflow/models/anthropic/completion';
AnthropicCompletion.run(/* args */)
AnthropicCompletion.stream(/* args */)
AnthropicCompletion.streamBytes(/* args */)
FAQs
Zero-dependency, modular SDK for building robust natural language applications
The npm package @axflow/models receives a total of 139 weekly downloads. As such, @axflow/models popularity was classified as not popular.
We found that @axflow/models demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.