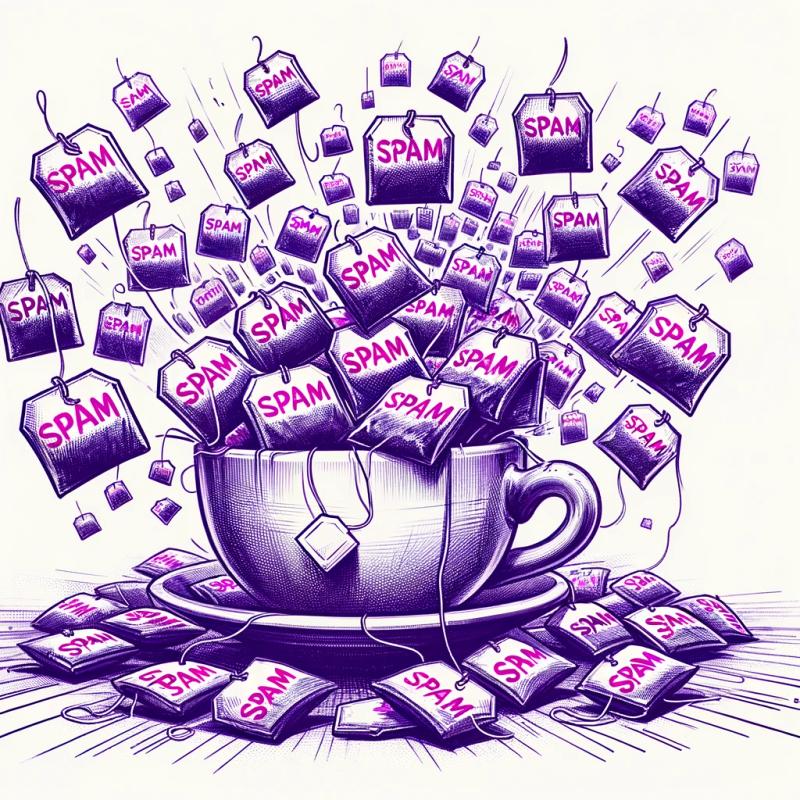
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@axmit/express-core-mongodb
Advanced tools
Readme
This package provide Mongodb module for @axmit/express-core
using Mongoose ODM
Before using this package you need to authorize yourself into npm registry using npm adduser
npm i @axmit/express-core-mongodb
or yarn add @axmit/express-core-mongodb
import { ExpressApplication } from '@axmit/express-core';
import { MongodbModule } from '@axmit/express-core-mongodb';
class YourApp extends ExpressApplication {
public async start() {
await this.addModule(new MongodbModule());
}
}
You must also specify MONGO_DB_URL
variable in your .env
file.
This package also provide gulp CLI command to seed you DB, you can add it in your gulp file like that:
require('@axmit/express-core-mongodb/gulp');
Use gulp mongoose-seed
to load you MongoDB seeders from seeders
folder
See MongooseSeeder
section for more info
Builder for mongoose models, which provides amount of methods to create your model and contain following methods:
Creates builder instance using provided model name
Adds model schema by fields
param containing classic object defining mongoose schema
Adds mongoose model index on specified fields
params, and also you can provide additional mongoose index options
Adds Mongoose schema options
Adds mongoose method to model instance
Params:
this
is mongoose instance model)Adds mongoose method to model instance
Params:
this
is mongoose instance model)Builds only mongoose Schema
and returns it
Build schema and model and return mongoose model class
Usage:
import { MongooseModelBuilder } from '@axmit/express-core-mongodb';
const AmazingModel = new MongooseModelBuilder('ModelName')
.useFields({ name: { required: true, type: String },
description: { required: true, type: String }
})
.build();
await new AmazingModel({ name: 'test', description: 'test' }).save();
Note: if you want TypeScript to correct handle your custom methods and statics, you must specify interfaces and pass it into builder generic params as follows:
import { MongooseDocument, MongooseModel, MongooseModelBuilder } from '@axmit/express-core-mongodb';
interface IAmazingDocumentFields extends MongooseDocument {
name: string;
description: string;
}
interface IAmazingDocument extends IAmazingDocumentFields {
printName(): void;
}
interface IAmazingModel extends MongooseModel<IAmazingDocument> {
printAllRecords(): void;
}
const AmazingModel = new MongooseModelBuilder<IAmazingDocument, IAmazingModel>('ModelName')
.useFields({ name: { required: true, type: String },
description: { required: true, type: String }
})
.addMethod('printName', function() {
console.log(this.name);
})
.addStatic('printAllRecords', async function() {
const records = await this.find({});
console.log(records);
})
.build();
Now you can use your static and method as usual
await AmazingModel.printAllRecords(); //prints all records in the collection
const amazingInstance = await new AmazingModel({ name: 'test', description: 'test1' }).save();
amazingInstance.printName(); //prints test
This helper cleans up all you collections
Usage:
import { clearMongoDB } from '@axmit/express-core-mongodb';
await clearMongoDB();
This abstract class helps you to implement mongoose seeders, you need
to create seeders
folder in your application root and make it return set of
seeders classes like this
import { MongooseSeeder } from '@axmit/express-core-mongodb';
export class TestSeeder extends MongooseSeeder {
public async shouldRun() {
return true;
}
public async run() {
//Fill your model here and return amount of created documents
}
}
Note: you must create index
file in seeders
dir which will export all seeders
FAQs
A package provide Mongodb module for @axmit/express-core using Mongoose ODM
The npm package @axmit/express-core-mongodb receives a total of 2 weekly downloads. As such, @axmit/express-core-mongodb popularity was classified as not popular.
We found that @axmit/express-core-mongodb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.