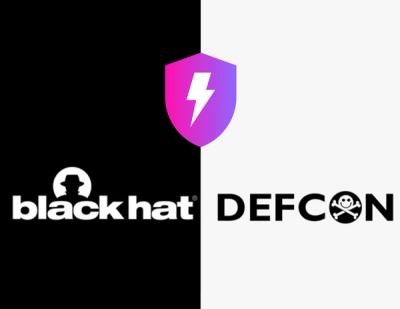
Security News
Meet Socket at BlackHat and DEF CON in Las Vegas
Come meet the Socket team at BlackHat and DEF CON! We're sponsoring some fun networking events and we would love to see you there.
@azure/identity
Advanced tools
Provides credential implementations for Azure SDK libraries that can authenticate with Azure Active Directory
Package description
The @azure/identity package provides Azure Active Directory token authentication support across the Azure SDK. It enables developers to authenticate with Azure services using various credentials and manage tokens.
DefaultAzureCredential
DefaultAzureCredential is a comprehensive credential chain that tries multiple methods of authentication, suitable for most Azure SDK clients.
const { DefaultAzureCredential } = require('@azure/identity');
const credential = new DefaultAzureCredential();
ClientSecretCredential
ClientSecretCredential authenticates with Azure AD using a client ID and secret. It's typically used for server-to-server authentication.
const { ClientSecretCredential } = require('@azure/identity');
const credential = new ClientSecretCredential(tenantId, clientId, clientSecret);
ManagedIdentityCredential
ManagedIdentityCredential allows an Azure service to authenticate to other Azure services using the managed identity of an Azure resource.
const { ManagedIdentityCredential } = require('@azure/identity');
const credential = new ManagedIdentityCredential();
EnvironmentCredential
EnvironmentCredential reads authentication details from environment variables and can be used for automated deployments or local development.
const { EnvironmentCredential } = require('@azure/identity');
const credential = new EnvironmentCredential();
InteractiveBrowserCredential
InteractiveBrowserCredential is used for applications that require interactive authentication by opening a default system browser.
const { InteractiveBrowserCredential } = require('@azure/identity');
const credential = new InteractiveBrowserCredential({ clientId: 'your-client-id' });
The AWS SDK for JavaScript provides a similar set of functionalities for AWS services as @azure/identity does for Azure. It includes various authentication methods and credential management.
Google's authentication library for Node.js offers OAuth2 client capabilities and other authentication features, similar to @azure/identity but for Google Cloud services.
This is a collection of Passport strategies to help you integrate with Azure Active Directory. It's more focused on web app scenarios and differs from @azure/identity which is more general-purpose.
Readme
This library simplifies authentication against Azure Active Directory for Azure SDK libraries.
It provides a set of TokenCredential
implementations which can be passed into SDK libraries
to authenticate API requests. It supports token authentication using an Azure Active Directory service principal or managed identity.
Install Azure Identity with npm
:
npm install --save @azure/identity
If this is your first time using @azure/identity
or the Microsoft identity platform (Azure Active Directory), we recommend that you read Using @azure/identity
with Microsoft Identity Platform first. This document will give you a deeper understanding of the platform and how to configure your Azure account correctly.
Azure Identity offers a variety of credential classes that are accepted by Azure SDK data plane clients. Each client library documents its Azure Identity integration in its README and samples. Azure SDK management plane libraries (those starting with @azure/arm-*
) do not accept these credentials.
Credentials differ mostly in configuration:
credential class | identity | configuration |
---|---|---|
DefaultAzureCredential | service principal or managed identity | none for managed identity; environment variables for service principal |
ManagedIdentityCredential | managed identity | none |
EnvironmentCredential | service principal | environment variables |
ClientSecretCredential | service principal | constructor parameters |
ClientCertificateCredential | service principal | constructor parameters |
DeviceCodeCredential | app registration details | constructor parameters |
AuthorizationCodeCredential | app registration details | constructor parameters |
InteractiveBrowserCredential | app registration details | constructor parameters |
UsernamePasswordCredential | user principal | constructor parameters |
Credentials can be chained and tried in turn until one succeeds; see chaining credentials for details.
DefaultAzureCredential
is appropriate for most scenarios. It supports authenticating as a service principal or managed identity. To authenticate as a service principal, provide configuration in environment variables as described in the next section. Currently this credential attempts to use the EnvironmentCredential
and ManagedIdentityCredential
, in that order.
Authenticating as a managed identity requires no configuration, but does require platform support. See the managed identity documentation for more information.
DefaultAzureCredential
and EnvironmentCredential
are configured for service principal authentication with these environment variables:
variable name | value |
---|---|
AZURE_CLIENT_ID | service principal's app id |
AZURE_TENANT_ID | id of the principal's Azure Active Directory tenant |
AZURE_CLIENT_SECRET | one of the service principal's client secrets (implies ClientSecretCredential ) |
AZURE_CLIENT_CERTIFICATE_PATH | one of the service principal's client secrets (implies ClientCertificateCredential ) |
AZURE_USERNAME | the username of a user in the tenant (implies UsernamePasswordCredential ) |
AZURE_PASSWORD | the password of the user specified in AZURE_USERNAME |
DefaultAzureCredential
// The default credential first checks environment variables for configuration as described above.
// If environment configuration is incomplete, it will try managed identity.
const { KeyClient } = require("@azure/keyvault-keys");
const { DefaultAzureCredential } = require("@azure/identity");
// Azure SDK clients accept the credential as a parameter
const credential = new DefaultAzureCredential();
const client = new KeyClient(vaultUrl, credential);
const getResult = await client.getKey("MyKeyName");
// Using a client secret
const { ClientSecretCredential } = require("@azure/identity");
const credential = new ClientSecretCredential(tenantId, clientId, clientSecret);
// Using a PEM-encoded certificate with a private key, not password protected
const { ClientCertificateCredential } = require("@azure/identity");
const credential = new ClientCertificateCredential(
tenantId,
clientId,
"/app/certs/certificate.pem"
);
// Using environment variables (see "Environment variables" above for variable names)
const { EnvironmentCredential } = require("@azure/identity");
const credential = new EnvironmentCredential();
AuthorizationCodeCredential
The AuthorizationCodeCredential
takes more up-front work to use than the other credential types at this time. A full sample demonstrating how to use this credential can be found in samples/authorizationCodeSample.ts
.
const { ClientSecretCredential, ChainedTokenCredential } = require("@azure/identity");
// When an access token is requested, the chain will try each
// credential in order, stopping when one provides a token
const firstCredential = new ClientSecretCredential(tenantId, clientId, clientSecret);
const secondCredential = new ClientSecretCredential(tenantId, anotherClientId, anotherSecret);
const credentialChain = new ChainedTokenCredential(firstCredential, secondCredential);
// The chain can be used anywhere a credential is required
const { KeysClient } = require("@azure/keyvault-keys");
const client = new KeysClient(vaultUrl, credentialChain);
Credentials raise AuthenticationError
when they fail to authenticate. This class has a message
field which describes why authentication failed. An AggregateAuthenticationError
will be raised by ChainedTokenCredential
with an errors
field containing an array of errors from each credential in the chain.
API documentation for this library can be found on our documentation site.
If you encounter bugs or have suggestions, please open an issue.
This project welcomes contributions and suggestions. Most contributions require you to agree to a Contributor License Agreement (CLA) declaring that you have the right to, and actually do, grant us the rights to use your contribution. For details, visit https://cla.microsoft.com.
When you submit a pull request, a CLA-bot will automatically determine whether you need to provide a CLA and decorate the PR appropriately (e.g., label, comment). Simply follow the instructions provided by the bot. You will only need to do this once across all repos using our CLA.
If you'd like to contribute to this library, please read the contributing guide to learn more about how to build and test the code.
This project has adopted the Microsoft Open Source Code of Conduct. For more information, see the Code of Conduct FAQ or contact opencode@microsoft.com with any additional questions or comments.
FAQs
Provides credential implementations for Azure SDK libraries that can authenticate with Microsoft Entra ID
We found that @azure/identity demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Come meet the Socket team at BlackHat and DEF CON! We're sponsoring some fun networking events and we would love to see you there.
Security News
Learn how Socket's 'Non-Existent Author' alert helps safeguard your dependencies by identifying npm packages published by deleted accounts. This is one of the fastest ways to determine if a package may be abandoned.
Security News
In July, the Python Software Foundation mounted a quick response to address a leaked GitHub token, elected new board members, and added more members to the team supporting PSF and PyPI infrastructure.