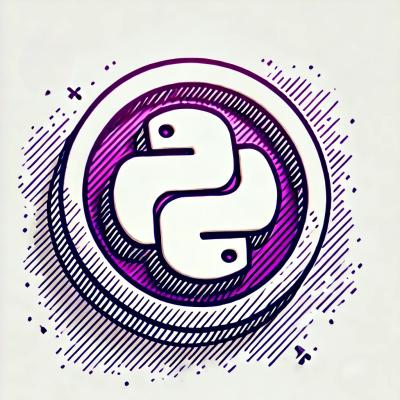
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
@babel/plugin-transform-for-of
Advanced tools
The @babel/plugin-transform-for-of package is a plugin for Babel, a JavaScript compiler, that transforms for...of loops into more compatible ES5 syntax. This is particularly useful for ensuring that your JavaScript code can run in environments that do not support the latest ECMAScript features, such as older browsers. The transformation helps in converting iterable objects in a way that can be understood by environments without native support for the for...of loop.
Transform for...of loops to ES5
This feature automatically converts for...of loops into a compatible ES5 syntax using simple iteration over arrays. This is useful for ensuring compatibility with older JavaScript environments.
"use strict";
var _arr = [1, 2, 3];
for (var _i = 0; _i < _arr.length; _i++) {
var i = _arr[_i];
console.log(i);
}
Optionally use loose mode for simpler output
In loose mode, the transformation is even simpler, avoiding the use of iterators altogether and directly accessing array elements by index. This results in faster code but assumes the iterated object is an array.
"use strict";
var _arr = [1, 2, 3];
for (var i = 0; i < _arr.length; i++) {
console.log(_arr[i]);
}
Similar to @babel/plugin-transform-for-of, this plugin transforms spread syntax (e.g., ...arr) into a form that can be understood by older JavaScript engines. While it focuses on spread syntax rather than for...of loops, it similarly aims to enhance compatibility with older environments.
This package transforms ES6 block scoping (let and const) into ES5 syntax. It's similar to @babel/plugin-transform-for-of in that it targets a specific ES6 feature for transformation to ensure compatibility with older JavaScript engines.
Compile ES2015 for...of to ES5
In
for (var i of foo) {}
Out
var _iteratorNormalCompletion = true;
var _didIteratorError = false;
var _iteratorError = undefined;
try {
for (var _iterator = foo[Symbol.iterator](), _step; !(_iteratorNormalCompletion = (_step = _iterator.next()).done); _iteratorNormalCompletion = true) {
var i = _step.value;
}
} catch (err) {
_didIteratorError = true;
_iteratorError = err;
} finally {
try {
if (!_iteratorNormalCompletion && _iterator.return != null) {
_iterator.return();
}
} finally {
if (_didIteratorError) {
throw _iteratorError;
}
}
}
npm install --save-dev @babel/plugin-transform-for-of
.babelrc
(Recommended).babelrc
Without options:
{
"plugins": ["@babel/plugin-transform-for-of"]
}
With options:
{
"plugins": [
["@babel/plugin-transform-for-of", {
"loose": true, // defaults to false
"assumeArray": true // defaults to false
}]
]
}
babel --plugins @babel/plugin-transform-for-of script.js
require("@babel/core").transform("code", {
plugins: ["@babel/plugin-transform-for-of"]
});
loose
boolean
, defaults to false
In loose mode, arrays are put in a fast path, thus heavily increasing performance. All other iterables will continue to work fine.
In
for (var i of foo) {}
Out
for (var _iterator = foo, _isArray = Array.isArray(_iterator), _i = 0, _iterator = _isArray ? _iterator : _iterator[Symbol.iterator]();;) {
var _ref;
if (_isArray) {
if (_i >= _iterator.length) break;
_ref = _iterator[_i++];
} else {
_i = _iterator.next();
if (_i.done) break;
_ref = _i.value;
}
var i = _ref;
}
In loose mode an iterator's return
method will not be called on abrupt completions caused by thrown errors.
Please see google/traceur-compiler#1773 and babel/babel#838 for more information.
assumeArray
boolean
, defaults to false
This will apply the optimization shown below to all for-of loops by assuming that all loops are arrays.
Can be useful when you just want a for-of loop to represent a basic for loop over an array.
If a basic array is used, Babel will compile the for-of loop down to a regular for loop.
In
for (let a of [1,2,3]) {}
Out
var _arr = [1, 2, 3];
for (var _i = 0; _i < _arr.length; _i++) {
var a = _arr[_i];
}
FAQs
Compile ES2015 for...of to ES5
The npm package @babel/plugin-transform-for-of receives a total of 19,787,190 weekly downloads. As such, @babel/plugin-transform-for-of popularity was classified as popular.
We found that @babel/plugin-transform-for-of demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.