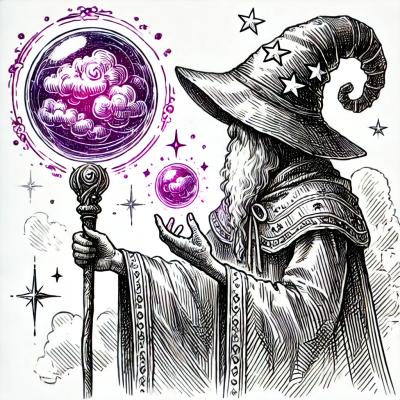
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@babel/plugin-transform-typeof-symbol
Advanced tools
This transformer wraps all typeof expressions with a method that replicates native behaviour. (ie. returning “symbol” for symbols)
The @babel/plugin-transform-typeof-symbol package is a plugin for Babel, a JavaScript compiler, that ensures the behavior of the 'typeof' operator is spec-compliant when used with symbols. In environments that do not fully support symbols, this plugin transforms 'typeof' checks to a method that can correctly identify 'symbol' types.
Transform typeof checks for symbols
This code represents the transformation that the plugin applies to 'typeof' checks. It defines a function that can correctly identify symbols, even in environments that do not natively support the 'symbol' type.
var _typeof = function(obj) { return obj && typeof Symbol !== 'undefined' && obj.constructor === Symbol ? 'symbol' : typeof obj; };
This package helps to reuse Babel's injected helper code to save on codesize. It includes a typeof helper for symbols, similar to what @babel/plugin-transform-typeof-symbol provides, but it's part of a broader set of transformations.
Although not a direct alternative, core-js is a modular standard library for JavaScript, which includes polyfills for symbols. It ensures that 'typeof' checks for symbols work correctly in older environments, similar to the goal of @babel/plugin-transform-typeof-symbol.
ES6 introduces a new native type called symbols. This transformer wraps all
typeof
expressions with a method that replicates native behaviour. (ie. returning "symbol" for symbols)
In
typeof Symbol() === "symbol";
Out
var _typeof = function (obj) {
return obj && obj.constructor === Symbol ? "symbol" : typeof obj;
};
_typeof(Symbol()) === "symbol";
npm install --save-dev @babel/plugin-transform-typeof-symbol
.babelrc
(Recommended).babelrc
{
"plugins": ["@babel/transform-typeof-symbol"]
}
babel --plugins @babel/transform-typeof-symbol script.js
require("@babel/core").transform("code", {
plugins: ["@babel/transform-typeof-symbol"]
});
FAQs
This transformer wraps all typeof expressions with a method that replicates native behaviour. (ie. returning “symbol” for symbols)
The npm package @babel/plugin-transform-typeof-symbol receives a total of 19,350,941 weekly downloads. As such, @babel/plugin-transform-typeof-symbol popularity was classified as popular.
We found that @babel/plugin-transform-typeof-symbol demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.