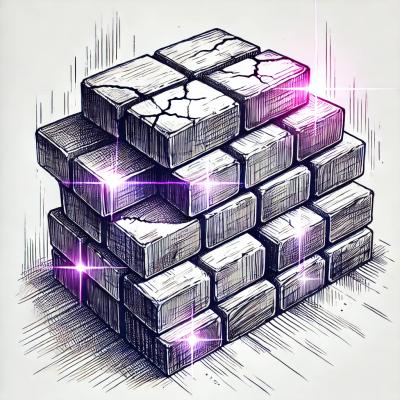
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@benev/argv
Advanced tools
@benev/argv
the greatest command line parser for typescript, maybe
🤖 for making node cli programs
🕵️ incredible typescript type inference
🧼 zero dependencies
💖 made free and open source, just for you
--help
pagespizza --help
pizza large --pepperoni --slices 3
# or, any way you like it
pizza --slices=9 large
pizza medium -p --slices=5
pizza small --pepperoni="no" --slices="2"
cli
@benev/argv
via npm
npm i @benev/argv
import {cli, command, arg, param, string, number} from "@benev/argv"
const {args, params} = cli(process.argv, {
name: "pizza",
commands: command({
args: [
arg("size").required(string),
],
params: {
slices: param.default(number, "1"),
pepperoni: param.flag("p"),
},
}),
}).tree
args.size // "large"
params.slices // 5
params.pepperoni // true
args
and params
command({
args: [],
params: {},
})
help
string
command({
help: "what a time to be alive!",
args: [],
params: {},
})
command({
args: [
arg("active").required(boolean),
arg("count").default(number, "101"),
arg("name").optional(string),
],
params: {},
})
required
, default
, and optional
default
requires a fallback valuestring
, number
, and boolean
, but you can make your own typescommand({
args: [],
params: {
active: param.required(boolean),
count: param.default(number, "101"),
name: param.optional(string),
verbose: param.flag("-v"),
},
})
flag
, of course, it's automatically a boolean
(how could it be otherwise?)validate
function on any arg
or param
arg("quality").optional(number, {
validate: n => {
if (n > 100) throw new Error("to big")
if (n < 0) throw new Error("to smol")
return n
},
})
validate
, it will be printed all nice-like to the userhelp
literally everywhere!arg
and param
can have its own help
command({
help: "it's the best command, nobody makes commands like me",
args: [
arg("active").required(boolean, {
help: "all systems go?",
}),
arg("count").default(number, "101", {
help: "number of dalmatians",
}),
arg("name").optional(string, {
help: `
see this multi-line string?
it will be trimmed all nicely on the help page.
`
}),
],
params: {
active: param.required(boolean, {
help: "toggle this carefully!",
}),
count: param.default(number, "101", {
help: "classroom i'm late for",
}),
name: param.optional(string, {
help: "pick your pseudonym",
}),
verbose: param.flag("-v", {
help: "going loud",
}),
},
})
choice
helperchoice
helper to set up a multiple choice string
param.required(string, choice(["thick", "thin"]))
param.required(string, choice(["thick", "thin"], {
help: "made with organic whole-wheat flour",
}))
list
helperparam.required(list(string))
list
helper
mp3,wav,ogg
["mp3", "wav", "ogg"]
param.required(list(number))
number[]
array (not strings)list
preserves the type's validationcommands
commands
object is a recursive tree with command
leaves
const {tree} = cli(process.argv, {
name: "converter",
commands: {
image: command({
args: [],
params: {
quality: param.required(number),
},
}),
media: {
audio: command({
args: [],
params: {
mono: param.required(boolean),
},
}),
video: command({
args: [],
params: {
codec: param.required(string),
},
})
},
},
})
tree
object that reflects its shape
tree.image?.params.quality // 9
tree.media.audio?.mono // false
tree.media.video?.codec // "av1"
undefined
except for the "selected" commandexecute
function
command({
args: [],
params: {
active: param.required(boolean),
count: param.default(number, "101"),
},
async execute({params}) {
params.active // true
params.count // 101
},
})
args
, params
, and some more stuffexecute
function can opt-into pretty-printing errors (with colors) by throwing an ExecutionError
import {ExecutionError, command} from "@benev/argv"
async execute({params}) {
throw new ExecutionError("scary error printed in red!")
}
execute
function
// 👇 awaiting cli execution
await cli(process.argv, {
name: "pizza",
commands: {
meatlovers: command({
args: [],
params: {
meatiness: param.required(number),
},
async execute({params}) {
console.log(params.meatiness) // 9
},
}),
hawaiian: command({
args: [],
params: {
pineappleyness: param.required(number),
},
async execute({params}) {
console.log(params.pineappleyness) // 8
},
}),
},
}).execute()
// ☝️ calling cli final execute
const date = asType({
name: "date",
coerce: string => new Date(string),
})
name
is shown in help pagescoerce
function takes a string input, and you turn it into anything you likeparam.required(date)
param.required(list(date))
const date = asTypes({
date: string => new Date(string),
integer: string => Math.floor(Number(string)),
})
asTypes
will use your object's property names as the type name
import {themes} from
await cli(process.argv, {
// the default theme
theme: themes.standard,
...otherStuff,
}).execute()
themes.seaside
for a more chill vibethemes.noColor
to disable ansi colorsimport {theme, color} from
const seaside = theme({
plain: [color.white],
error: [color.brightRed, color.bold],
program: [color.brightCyan, color.bold],
command: [color.cyan, color.bold],
property: [color.blue],
link: [color.brightBlue, color.underline],
arg: [color.brightBlue, color.bold],
param: [color.brightBlue, color.bold],
flag: [color.brightBlue],
required: [color.cyan],
mode: [color.blue],
type: [color.brightBlue],
value: [color.cyan],
})
v0.3.1
seaside
themeFAQs
command line argument parser
The npm package @benev/argv receives a total of 129 weekly downloads. As such, @benev/argv popularity was classified as not popular.
We found that @benev/argv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.